Merge branch 'master' into develop
commit
f1a3ce49cc
|
@ -10,9 +10,11 @@ If you want to use RGB LED's you should use the [RGB Matrix Subsystem](feature_r
|
|||
|
||||
There is basic support for addressable LED matrix lighting with the I2C IS31FL3731 RGB controller. To enable it, add this to your `rules.mk`:
|
||||
|
||||
LED_MATRIX_ENABLE = yes
|
||||
LED_MATRIX_DRIVER = IS31FL3731
|
||||
|
||||
```make
|
||||
LED_MATRIX_ENABLE = yes
|
||||
LED_MATRIX_DRIVER = IS31FL3731
|
||||
```
|
||||
|
||||
You can use between 1 and 4 IS31FL3731 IC's. Do not specify `LED_DRIVER_ADDR_<N>` defines for IC's that are not present on your keyboard. You can define the following items in `config.h`:
|
||||
|
||||
| Variable | Description | Default |
|
||||
|
@ -28,33 +30,38 @@ You can use between 1 and 4 IS31FL3731 IC's. Do not specify `LED_DRIVER_ADDR_<N>
|
|||
|
||||
Here is an example using 2 drivers.
|
||||
|
||||
// This is a 7-bit address, that gets left-shifted and bit 0
|
||||
// set to 0 for write, 1 for read (as per I2C protocol)
|
||||
// The address will vary depending on your wiring:
|
||||
// 0b1110100 AD <-> GND
|
||||
// 0b1110111 AD <-> VCC
|
||||
// 0b1110101 AD <-> SCL
|
||||
// 0b1110110 AD <-> SDA
|
||||
#define LED_DRIVER_ADDR_1 0b1110100
|
||||
#define LED_DRIVER_ADDR_2 0b1110110
|
||||
```c
|
||||
// This is a 7-bit address, that gets left-shifted and bit 0
|
||||
// set to 0 for write, 1 for read (as per I2C protocol)
|
||||
// The address will vary depending on your wiring:
|
||||
// 0b1110100 AD <-> GND
|
||||
// 0b1110111 AD <-> VCC
|
||||
// 0b1110101 AD <-> SCL
|
||||
// 0b1110110 AD <-> SDA
|
||||
#define LED_DRIVER_ADDR_1 0b1110100
|
||||
#define LED_DRIVER_ADDR_2 0b1110110
|
||||
|
||||
#define LED_DRIVER_COUNT 2
|
||||
#define LED_DRIVER_1_LED_COUNT 25
|
||||
#define LED_DRIVER_2_LED_COUNT 24
|
||||
#define LED_DRIVER_LED_COUNT LED_DRIVER_1_LED_TOTAL + LED_DRIVER_2_LED_TOTAL
|
||||
#define LED_DRIVER_COUNT 2
|
||||
#define LED_DRIVER_1_LED_COUNT 25
|
||||
#define LED_DRIVER_2_LED_COUNT 24
|
||||
#define LED_DRIVER_LED_COUNT LED_DRIVER_1_LED_TOTAL + LED_DRIVER_2_LED_TOTAL
|
||||
```
|
||||
|
||||
Currently only 2 drivers are supported, but it would be trivial to support all 4 combinations.
|
||||
|
||||
Define these arrays listing all the LEDs in your `<keyboard>.c`:
|
||||
|
||||
const is31_led g_is31_leds[DRIVER_LED_TOTAL] = {
|
||||
/* Refer to IS31 manual for these locations
|
||||
* driver
|
||||
* | LED address
|
||||
* | | */
|
||||
{0, C3_3},
|
||||
....
|
||||
}
|
||||
```c
|
||||
const is31_led g_is31_leds[DRIVER_LED_TOTAL] = {
|
||||
/* Refer to IS31 manual for these locations
|
||||
* driver
|
||||
* | LED address
|
||||
* | | */
|
||||
{ 0, C1_1 },
|
||||
{ 0, C1_15 },
|
||||
// ...
|
||||
}
|
||||
```
|
||||
|
||||
Where `Cx_y` is the location of the LED in the matrix defined by [the datasheet](https://www.issi.com/WW/pdf/31FL3731.pdf) and the header file `drivers/issi/is31fl3731-simple.h`. The `driver` is the index of the driver you defined in your `config.h` (`0`, `1`, `2`, or `3` ).
|
||||
|
||||
|
@ -66,26 +73,28 @@ All LED matrix keycodes are currently shared with the [backlight system](feature
|
|||
|
||||
Currently no LED matrix effects have been created.
|
||||
|
||||
## Custom layer effects
|
||||
## Custom Layer Effects
|
||||
|
||||
Custom layer effects can be done by defining this in your `<keyboard>.c`:
|
||||
|
||||
void led_matrix_indicators_kb(void) {
|
||||
led_matrix_set_index_value(index, value);
|
||||
}
|
||||
```c
|
||||
void led_matrix_indicators_kb(void) {
|
||||
led_matrix_set_index_value(index, value);
|
||||
}
|
||||
```
|
||||
|
||||
A similar function works in the keymap as `led_matrix_indicators_user`.
|
||||
|
||||
## Suspended state
|
||||
## Suspended State
|
||||
|
||||
To use the suspend feature, add this to your `<keyboard>.c`:
|
||||
|
||||
void suspend_power_down_kb(void)
|
||||
{
|
||||
led_matrix_set_suspend_state(true);
|
||||
}
|
||||
```c
|
||||
void suspend_power_down_kb(void) {
|
||||
led_matrix_set_suspend_state(true);
|
||||
}
|
||||
|
||||
void suspend_wakeup_init_kb(void)
|
||||
{
|
||||
led_matrix_set_suspend_state(false);
|
||||
}
|
||||
void suspend_wakeup_init_kb(void) {
|
||||
led_matrix_set_suspend_state(false);
|
||||
}
|
||||
```
|
|
@ -0,0 +1,155 @@
|
|||
# ADC ドライバ
|
||||
|
||||
<!---
|
||||
original document: 0.10.52:docs/adc_driver.md
|
||||
git diff 0.10.52 HEAD -- docs/adc_driver.md | cat
|
||||
-->
|
||||
|
||||
QMK は対応している MCU のアナログ・デジタルコンバータ(ADC) を使用し、特定のピンの電圧を計測することができます。この機能はデジタル出力の[ロータリーエンコーダ](ja/feature_encoders.md)などではなく、アナログ計測が必要な可変抵抗器を使用したボリュームコントロールや Bluetooth キーボードのバッテリー残量表示などの実装に役立ちます。
|
||||
|
||||
このドライバは現在 AVR と一部の ARM デバイスをサポートしています。返される値は 0V と VCC (通常 AVR の場合は 5V または 3.3V、ARM の場合は 3.3V)の間でマッピングされた 10ビットの整数 (0-1023) ですが、ARM の場合、もしもより精度が必要であれば `#define` を使うと操作をより柔軟に制御できます。
|
||||
|
||||
## 使い方
|
||||
|
||||
このドライバを使うには、`rules.mk` に以下を追加します:
|
||||
|
||||
```make
|
||||
SRC += analog.c
|
||||
```
|
||||
|
||||
そして、コードの先頭に以下の include を置きます:
|
||||
|
||||
```c
|
||||
#include "analog.h"
|
||||
```
|
||||
|
||||
## チャンネル
|
||||
|
||||
### AVR
|
||||
|
||||
|Channel|AT90USB64/128|ATmega16/32U4|ATmega32A|ATmega328/P|
|
||||
|-------|-------------|-------------|---------|-----------|
|
||||
|0 |`F0` |`F0` |`A0` |`C0` |
|
||||
|1 |`F1` |`F1` |`A1` |`C1` |
|
||||
|2 |`F2` | |`A2` |`C2` |
|
||||
|3 |`F3` | |`A3` |`C3` |
|
||||
|4 |`F4` |`F4` |`A4` |`C4` |
|
||||
|5 |`F5` |`F5` |`A5` |`C5` |
|
||||
|6 |`F6` |`F6` |`A6` |* |
|
||||
|7 |`F7` |`F7` |`A7` |* |
|
||||
|8 | |`D4` | | |
|
||||
|9 | |`D6` | | |
|
||||
|10 | |`D7` | | |
|
||||
|11 | |`B4` | | |
|
||||
|12 | |`B5` | | |
|
||||
|13 | |`B6` | | |
|
||||
|
||||
<sup>\* ATmega328/P には余分な2つの ADC チャンネルがありますが、DIP ピンアウトには存在せず、GPIO ピンとは共有されません。これらに直接アクセスするために、`adc_read()` を使えます。
|
||||
|
||||
### ARM
|
||||
|
||||
これらのピンの一部は同じチャンネルを使って ADC 上でダブルアップされることに注意してください。これは、これらのピンがどちらかの ADC に使われる可能性があるからです。
|
||||
|
||||
また、F0 と F3 は異なるナンバリングスキーマを使うことに注意してください。F0 には1つの ADC があり、チャンネルは0から始まるインデックスですが、F3 には4つの ADC があり、チャンネルは1から始まるインデックスです。これは、F0 が ADC の `ADCv1` 実装を使用するのに対し、F3 が `ADCv3` 実装を使用するためです。
|
||||
|
||||
|ADC|Channel|STM32F0xx|STM32F3xx|
|
||||
|---|-------|---------|---------|
|
||||
|1 |0 |`A0` | |
|
||||
|1 |1 |`A1` |`A0` |
|
||||
|1 |2 |`A2` |`A1` |
|
||||
|1 |3 |`A3` |`A2` |
|
||||
|1 |4 |`A4` |`A3` |
|
||||
|1 |5 |`A5` |`F4` |
|
||||
|1 |6 |`A6` |`C0` |
|
||||
|1 |7 |`A7` |`C1` |
|
||||
|1 |8 |`B0` |`C2` |
|
||||
|1 |9 |`B1` |`C3` |
|
||||
|1 |10 |`C0` |`F2` |
|
||||
|1 |11 |`C1` | |
|
||||
|1 |12 |`C2` | |
|
||||
|1 |13 |`C3` | |
|
||||
|1 |14 |`C4` | |
|
||||
|1 |15 |`C5` | |
|
||||
|1 |16 | | |
|
||||
|2 |1 | |`A4` |
|
||||
|2 |2 | |`A5` |
|
||||
|2 |3 | |`A6` |
|
||||
|2 |4 | |`A7` |
|
||||
|2 |5 | |`C4` |
|
||||
|2 |6 | |`C0` |
|
||||
|2 |7 | |`C1` |
|
||||
|2 |8 | |`C2` |
|
||||
|2 |9 | |`C3` |
|
||||
|2 |10 | |`F2` |
|
||||
|2 |11 | |`C5` |
|
||||
|2 |12 | |`B2` |
|
||||
|2 |13 | | |
|
||||
|2 |14 | | |
|
||||
|2 |15 | | |
|
||||
|2 |16 | | |
|
||||
|3 |1 | |`B1` |
|
||||
|3 |2 | |`E9` |
|
||||
|3 |3 | |`E13` |
|
||||
|3 |4 | | |
|
||||
|3 |5 | | |
|
||||
|3 |6 | |`E8` |
|
||||
|3 |7 | |`D10` |
|
||||
|3 |8 | |`D11` |
|
||||
|3 |9 | |`D12` |
|
||||
|3 |10 | |`D13` |
|
||||
|3 |11 | |`D14` |
|
||||
|3 |12 | |`B0` |
|
||||
|3 |13 | |`E7` |
|
||||
|3 |14 | |`E10` |
|
||||
|3 |15 | |`E11` |
|
||||
|3 |16 | |`E12` |
|
||||
|4 |1 | |`E14` |
|
||||
|4 |2 | |`B12` |
|
||||
|4 |3 | |`B13` |
|
||||
|4 |4 | |`B14` |
|
||||
|4 |5 | |`B15` |
|
||||
|4 |6 | |`E8` |
|
||||
|4 |7 | |`D10` |
|
||||
|4 |8 | |`D11` |
|
||||
|4 |9 | |`D12` |
|
||||
|4 |10 | |`D13` |
|
||||
|4 |11 | |`D14` |
|
||||
|4 |12 | |`D8` |
|
||||
|4 |13 | |`D9` |
|
||||
|4 |14 | | |
|
||||
|4 |15 | | |
|
||||
|4 |16 | | |
|
||||
|
||||
## 関数
|
||||
|
||||
### AVR
|
||||
|
||||
|関数 |説明 |
|
||||
|----------------------------|------------------------------------------------------------------------------------------------------------------------------------|
|
||||
|`analogReference(mode)` |アナログの電圧リファレンスソースを設定する。`ADC_REF_EXTERNAL`、`ADC_REF_POWER`、`ADC_REF_INTERNAL` のいずれかでなければなりません。|
|
||||
|`analogReadPin(pin)` |指定されたピンから値を読み取ります。例えば、ATmega32U4 の ADC6 の場合 `F6`。 |
|
||||
|`pinToMux(pin)` |指定されたピンを mux 値に変換します。サポートされていないピンが指定された場合、"0V (GND)" の mux 値を返します。 |
|
||||
|`adc_read(mux)` |指定された mux に従って ADC から値を読み取ります。詳細は、MCU のデータシートを見てください。 |
|
||||
|
||||
### ARM
|
||||
|
||||
|関数 |説明 |
|
||||
|----------------------------|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
|
||||
|`analogReadPin(pin)` |指定されたピンから値を読み取ります。STM32F0 では チャンネル 0 の `A0`、STM32F3 ではチャンネル 1 の ADC1。ピンを複数の ADC に使える場合は、この関数のために番号の小さい ADC が選択されることに注意してください。例えば、`C0` は、ADC2 にも使える場合、ADC1 のチャンネル 6 になります。 |
|
||||
|`analogReadPinAdc(pin, adc)`|指定されたピンと ADC から値を読み取ります。例えば、`C0, 1` は、ADC1 ではなく ADC2 のチャンネル 6 から読み取ります。この関数では、ADC はインデックス 0 から始まることに注意してください。 |
|
||||
|`pinToMux(pin)` |指定されたピンをチャンネルと ADC の組み合わせに変換します。サポートされていないピンが指定された場合、"0V (GND)" の mux 値を返します。 |
|
||||
|`adc_read(mux)` |指定されたピンと ADC の組み合わせに応じて ADC から値を読み取ります。詳細は、MCU のデータシートを見てください。 |
|
||||
|
||||
## 設定
|
||||
|
||||
## ARM
|
||||
|
||||
ADC の ARM 実装には、独自のキーボードとキーマップでオーバーライドして動作方法を変更できる幾つかの追加オプションがあります。利用可能なオプションの詳細については、特定のマイクロコントローラについて ChibiOS の対応する `hal_adc_lld.h` を調べてください。
|
||||
|
||||
|`#define` |型 |既定値 |説明 |
|
||||
|---------------------|------|---------------------|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
|
||||
|`ADC_CIRCULAR_BUFFER`|`bool`|`false` |`true` の場合、この実装は循環バッファを使います。 |
|
||||
|`ADC_NUM_CHANNELS` |`int` |`1` |ADC 動作の一部としてスキャンされるチャンネル数を設定します。現在の実装は `1` のみをサポートします。 |
|
||||
|`ADC_BUFFER_DEPTH` |`int` |`2` |各結果の深さを設定します。デフォルトでは12ビットの結果しか取得できないため、これを2バイトに設定して1つの値を含めることができます。8ビット以下の結果を選択した場合は、これを 1 に設定できます。 |
|
||||
|`ADC_SAMPLING_RATE` |`int` |`ADC_SMPR_SMP_1P5` |ADC のサンプリングレートを設定します。デフォルトでは、最も速い設定に設定されています。 |
|
||||
|`ADC_RESOLUTION` |`int` |`ADC_CFGR1_RES_12BIT`|結果の分解能。デフォルトでは12ビットを選択しますが、12、10、8、6ビットを選択できます。 |
|
|
@ -0,0 +1,277 @@
|
|||
# Unicode サポート
|
||||
|
||||
<!---
|
||||
original document: 0.10.53:docs/feature_unicode.md
|
||||
git diff 0.10.53 HEAD -- docs/feature_unicode.md | cat
|
||||
-->
|
||||
|
||||
Unicode 文字はキーボードから直接入力することができます!ただし幾つかの制限があります。
|
||||
|
||||
キーボードで Unicode サポートを有効にするには、以下の事をする必要があります:
|
||||
|
||||
1. サポートされている Unicode 実装のいずれかを選択します: [Basic Unicode](#basic-unicode)、[Unicode Map](#unicode-map)、[UCIS](#ucis)。
|
||||
2. オペレーティングシステムとセットアップに最適な[入力モード](#input-modes)を見つけます。
|
||||
3. コンフィギュレーションに適切な入力モード(または複数のモード)を[設定](#setting-the-input-mode)します。
|
||||
4. キーマップに Unicode キーコードを追加します。
|
||||
|
||||
|
||||
## 1. メソッド :id=methods
|
||||
|
||||
QMK は、Unicode 入力を有効にし、キーマップに Unicode 文字を追加するための3つの異なる方法をサポートします。それぞれに柔軟性と使いやすさの点で長所と短所があります。あなたの使い方に最適なものを選んでください。
|
||||
|
||||
ほとんどのユーザには Basic Unicode で十分です。ただし、サポートされる文字の範囲が広い(絵文字、珍しい記号など)ことが必要な場合には、Unicode Map を使う必要があります。
|
||||
|
||||
<br>
|
||||
|
||||
### 1.1. Basic Unicode :id=basic-unicode
|
||||
|
||||
多少制限はありますが、最も使いやすい方法です。Unicode 文字をキーコードとしてキーマップ自体に格納するため、`0x7FFF` までのコードポイントのみをサポートします。これは、ほとんどの現代言語(東アジアを含む)の文字と記号を対象としますが、絵文字は対象外です。
|
||||
|
||||
以下を `rules.mk` に追加します:
|
||||
|
||||
```make
|
||||
UNICODE_ENABLE = yes
|
||||
```
|
||||
|
||||
次に、`UC(c)` キーコードをキーマップに追加します。ここで、_c_ は目的の文字のコードポイントです (できれば16進数で最大4桁の長さが望ましいです)。例えば、`UC(0x40B)` は [Ћ](https://unicode-table.com/en/040B/) を出力し、`UC(0x30C4)` は [ツ](https://unicode-table.com/en/30C4) を出力します。
|
||||
|
||||
<br>
|
||||
|
||||
### 1.2. Unicode Map :id=unicode-map
|
||||
|
||||
このメソッドは、標準の文字の範囲に加えて、絵文字、古代文字、珍しい記号なども対象にしています。実際、可能な全てのコードポイント(`0x10FFFF`まで)がサポートされています。Unicode 文字は独立のマッピングテーブルに格納されています。キーマップファイルに `unicode_map` 配列を維持する必要があります。これには最大 16384 エントリを含めることができます。
|
||||
|
||||
以下を `rules.mk` に追加します:
|
||||
|
||||
```make
|
||||
UNICODEMAP_ENABLE = yes
|
||||
```
|
||||
|
||||
次に、`X(i)` キーコードをキーマップに追加します。ここで _i_ はマッピングテーブル内の目的の文字のインデックスです。これは数値にできますが、インデックスを列挙型に保持し、名前でアクセスすることをお勧めします。
|
||||
|
||||
```c
|
||||
enum unicode_names {
|
||||
BANG,
|
||||
IRONY,
|
||||
SNEK
|
||||
};
|
||||
|
||||
const uint32_t PROGMEM unicode_map[] = {
|
||||
[BANG] = 0x203D, // ‽
|
||||
[IRONY] = 0x2E2E, // ⸮
|
||||
[SNEK] = 0x1F40D, // 🐍
|
||||
};
|
||||
```
|
||||
|
||||
そして、キーマップで `X(BANG)`、`X(SNEK)` などを使うことができます。
|
||||
|
||||
#### 小文字と大文字
|
||||
|
||||
文字は å や Å のような小文字と大文字のペアで提供されることがあります。これらの文字を入力しやすくするために、キーマップで `XP(i, j)` を使うことができます。ここで、_i_ および _j_ はそれぞれ小文字と大文字のマッピングテーブルのインデックスです。キーを押した時に、シフトを押したままか Caps Lock をオンにしている場合は、2番目(大文字)の文字が挿入されます; そうでなければ最初(小文字)バージョンが出力されます。
|
||||
|
||||
これは特殊文字がある国際レイアウトのためのキーマップを作成している時に最も役立ちます。別々のキーに文字の小文字および大文字バージョンを置く代わりに、`XP()` を使ってそれら両方を同じキーに持つことができます。これは Unicode キーを通常のアルファベットと混ぜるのに役立ちます。
|
||||
|
||||
キーコードのサイズの制約により、_i_ と _j_ はそれぞれ `unicode_map` の最初の128文字のうち1つだけを参照できます。別の言い方をすると、0 ≤ _i_ ≤ 127 かつ 0 ≤ _j_ ≤ 127 です。これはほとんどのユースケースで十分ですが、インデックス計算をカスタマイズしたい場合は、[`unicodemap_index()`](https://github.com/qmk/qmk_firmware/blob/71f640d47ee12c862c798e1f56392853c7b1c1a8/quantum/process_keycode/process_unicodemap.c#L36) 関数をオーバーライドすることができます。これにより、例えば Shift/Caps の代わりに Ctrl をチェックすることもできます。
|
||||
|
||||
<br>
|
||||
|
||||
### 1.3. UCIS :id=ucis
|
||||
|
||||
この方法も全ての可能なコードポイントをサポートします。Unicode Map の方法と同様に、キーマップファイル内にマッピングテーブルを保持する必要があります。ただし、この機能のための組み込みのキーコードはありません — この機能を起動するカスタムキーコードあるいは関数を作成する必要があります。
|
||||
|
||||
以下を `rules.mk` に追加します:
|
||||
|
||||
```make
|
||||
UCIS_ENABLE = yes
|
||||
```
|
||||
|
||||
次に、キーマップファイルでこのようにテーブルを定義します:
|
||||
|
||||
```c
|
||||
const qk_ucis_symbol_t ucis_symbol_table[] = UCIS_TABLE(
|
||||
UCIS_SYM("poop", 0x1F4A9), // 💩
|
||||
UCIS_SYM("rofl", 0x1F923), // 🤣
|
||||
UCIS_SYM("cuba", 0x1F1E8, 0x1F1FA), // 🇨🇺
|
||||
UCIS_SYM("look", 0x0CA0, 0x005F, 0x0CA0), // ಠ_ಠ
|
||||
);
|
||||
```
|
||||
|
||||
デフォルトでは、各テーブルエントリの長さは、最大3コードポイントです。この番号は `#define UCIS_MAX_CODE_POINTS n` を `config.h` ファイルに追加することで変更できます。
|
||||
|
||||
UCIS 入力を使うには、`qk_ucis_start()` を呼び出します。次に、文字のニーモニック ("rofl" など) を入力し、Space か Enter か Esc を押します。QMK は "rofl" テキストを消去し、笑っている絵文字を挿入するはずです。
|
||||
|
||||
#### カスタマイズ
|
||||
|
||||
この機能をカスタマイズするためにキーマップで定義できる幾つかの関数があります。
|
||||
|
||||
* `void qk_ucis_start_user(void)` – これは "start" 関数を呼び出す時に実行され、フィードバックを提供するために使うことができます。デフォルトでは、キーボードの絵文字を入力します。
|
||||
* `void qk_ucis_success(uint8_t symbol_index)` – これは入力が何かに一致して完了した時に実行されます。デフォルトでは何もしません。
|
||||
* `void qk_ucis_symbol_fallback (void)` – これは入力が何にも一致しない時に実行されます。デフォルトでは、入力を Unicode コードとして試そうとします。
|
||||
|
||||
[`process_ucis.c`](https://github.com/qmk/qmk_firmware/blob/master/quantum/process_keycode/process_ucis.c) でこれらの関数のデフォルトの実装を見つけることができます。
|
||||
|
||||
|
||||
## 2. Input モード :id=input-modes
|
||||
|
||||
QMK での Unicode の入力は、マクロのように、OS への一連の文字列を入力することで動作します。残念ながら、これが行われる方法はプラットフォームによって異なります。特に各プラットフォームでは Unicode 入力を引き起こすために、異なるキーの組み合わせが必要です。従って、対応する入力モードが QMK で設定されなければなりません。
|
||||
|
||||
以下の入力モードが利用可能です:
|
||||
|
||||
* **`UC_MAC`**: macOS の組み込み Unicode 16進数入力。`0x10FFFF` までのコードポイント(全ての利用可能なコードポイント)をサポートします。
|
||||
|
||||
有効にするには、_システム環境設定 > キーボード > 入力ソース_ に移動し、(_その他_ の下の) _Unicode 16進数入力_ をリストに追加し、次にメニューバーの入力ドロップダウンからそれをアクティブにします。
|
||||
デフォルトでは、このモードは Unicode 入力のために左 Option キー (`KC_LALT`) を使いますが、これは他のキーで [`UNICODE_KEY_MAC`](#input-key-configuration) を定義することで変更できます。
|
||||
|
||||
!> _Unicode 16進数入力_ 入力ソースの使用は、Option + 左矢印および Option + 右矢印 のような、幾つかの Option ベースのショートカットを無効にするかもしれません。
|
||||
|
||||
!> `UC_OSX` は `UC_MAC` の非推奨のエイリアスで、QMK の将来のバージョンで削除されます。全ての新しいキーマップは、`UC_MAC` を使うべきです。
|
||||
|
||||
* **`UC_LNX`**: Linux の組み込み IBus Unicode 入力。`0x10FFFF` までのコードポイント(全ての利用可能なコードポイント)をサポートします。
|
||||
|
||||
デフォルトで有効になっていて、IBus が有効になったディストリビューションのほとんどどれでも動作します。IBus が無い場合、このモードは GTK アプリ下で動作しますが、他の場所ではほとんど動作しません。
|
||||
デフォルトでは、このモードは Unicode 入力を開始するために Ctrl+Shift+U (`LCTL(LSFT(KC_U))`) を使いますが、これは他のキーコードで [`UNICODE_KEY_LNX`](#input-key-configuration) を定義することで変更できます。これは、Ctrl+Shift+U の挙動が Ctrl+Shift+E に統合された IBus バージョン 1.5.15 以上を必要とするかもしれません。
|
||||
|
||||
* **`UC_WIN`**: _(非推奨)_ Windows の組み込み16進数テンキー Unicode 入力。`0xFFFF` までのコードポイントをサポートします。
|
||||
|
||||
有効にするには、`HKEY_CURRENT_USER\Control Panel\Input Method` の下に、`EnableHexNumpad` という名前の `REG_SZ` 型のレジストリキーを作成し、その値を `1` に設定します。これは、管理者権限でコマンドラインプロンプトから `reg add "HKCU\Control Panel\Input Method" -v EnableHexNumpad -t REG_SZ -d 1` を実行することでできます。その後再起動します。
|
||||
信頼性と互換性の問題から、このモードはお勧めできません; 代わりに `UC_WINC` モードを使ってください。
|
||||
|
||||
* **`UC_BSD`**: _(未実装)_ BSD での Unicode 入力。現時点では実装されていません。BSD ユーザでサポートを追加したい場合は、[GitHub で issue を開いて](https://github.com/qmk/qmk_firmware/issues)ください。
|
||||
|
||||
* **`UC_WINC`**: [WinCompose](https://github.com/samhocevar/wincompose) を使った Windows Unicode 入力。v0.9.0 の時点で、`0x10FFFF` までのコードポイント(全ての利用可能なコードポイント)をサポートします。
|
||||
|
||||
有効にするには、[最新のリリース](https://github.com/samhocevar/wincompose/releases/latest)をインストールします。インストールすると、起動時に WinCompose が自動的に実行されます。このモードはアプリがサポートする全てのバージョンの Windows で確実に動作します。
|
||||
デフォルトでは、このモードは Compose キーとして右 Alt (`KC_RALT`) を使いますが、これは WinCompose 設定と他のキーで [`UNICODE_KEY_WINC`](#input-key-configuration) を定義することで変更できます。
|
||||
|
||||
|
||||
## 3. 入力モードの設定 :id=setting-the-input-mode
|
||||
|
||||
目的の入力モードを設定するには、以下の定義を `config.h` に追加します:
|
||||
|
||||
```c
|
||||
#define UNICODE_SELECTED_MODES UC_LNX
|
||||
```
|
||||
|
||||
この例では、キーボードのデフォルトの入力モードを `UC_LNX` に設定します。これは、`UC_MAC` か `UC_WINC` か[上記](#input-modes)に列挙されている他のモードのいずれかに置き換えることができます。手動で別のモード([下記](#keycodes)を見てください)に切り替えない限り、キーボードは起動時に選択したモードを自動的に使います。
|
||||
|
||||
複数の入力モードを選択することもできます。これにより、`UC_MOD`/`UC_RMOD` キーコードを使ってそれらを簡単に切り替えることができます。
|
||||
|
||||
```c
|
||||
#define UNICODE_SELECTED_MODES UC_MAC, UC_LNX, UC_WINC
|
||||
```
|
||||
|
||||
値はカンマで区切られていることに注意してください。キーボードは最後に使われた入力モードを記憶し、次の電源投入時にそれを使い続けます。`config.h` に `#define UNICODE_CYCLE_PERSIST false` を追加することで、これを無効にして常にリストの最初のモードで開始するように強制できます。
|
||||
|
||||
#### キーコード
|
||||
|
||||
以下のキーコードを使って、いつでも入力モードを切り替えることができます。これらをキーマップに追加すると、`UNICODE_SELECTED_MODES` に列挙されていないモードを含む特定の入力モードに素早く切り替えることができます。
|
||||
|
||||
| キーコード |エイリアス | 入力モード | 説明 |
|
||||
|------------------------|-----------|--------------|--------------------------------------------------------------------|
|
||||
| `UNICODE_MODE_FORWARD` | `UC_MOD` | リストの次へ | 選択したモードを切り替えます。Shift が押された場合は逆方向 |
|
||||
| `UNICODE_MODE_REVERSE` | `UC_RMOD` | リストの前へ | 逆方向に選択したモードを切り替えます。Shift が押された場合は順方向 |
|
||||
| `UNICODE_MODE_MAC` | `UC_M_MA` | `UC_MAC` | macOS 入力に切り替え |
|
||||
| `UNICODE_MODE_LNX` | `UC_M_LN` | `UC_LNX` | Linux 入力に切り替え |
|
||||
| `UNICODE_MODE_WIN` | `UC_M_WI` | `UC_WIN` | Windows 入力に切り替え |
|
||||
| `UNICODE_MODE_BSD` | `UC_M_BS` | `UC_BSD` | BSD 入力に切り替え _(未実装)_ |
|
||||
| `UNICODE_MODE_WINC` | `UC_M_WC` | `UC_WINC` | WinCompose を使う Windows 入力に切り替え |
|
||||
|
||||
コード内で `set_unicode_input_mode(x)` を呼び出すことで、入力モードを切り替えることもできます。ここで、_x_ は上記の入力モード定数のいずれか (例えば、`UC_LNX`) です。
|
||||
|
||||
?> `matrix_init_user()` または同様の関数の中で `set_unicode_input_mode()` を呼び出すよりも、`UNICODE_SELECTED_MODES` を使うほうが望ましいです。Unicode システムとの統合性が高く、EEPROM への不要な書き込みを回避できるという利点があるからです。
|
||||
|
||||
#### オーディオフィードバック
|
||||
|
||||
キーボードで[オーディオ機能](ja/feature_audio.md)を有効にした場合、上記のキーを押したときにメロディーを再生するように設定できます。そのようにして、入力モードを切り替えた時になんらかのオーディオフィードバックを得ることができます。
|
||||
|
||||
例えば、`config.h` ファイルに下記の定義を追加することができます:
|
||||
|
||||
```c
|
||||
#define UNICODE_SONG_MAC AUDIO_ON_SOUND
|
||||
#define UNICODE_SONG_LNX UNICODE_LINUX
|
||||
#define UNICODE_SONG_BSD TERMINAL_SOUND
|
||||
#define UNICODE_SONG_WIN UNICODE_WINDOWS
|
||||
#define UNICODE_SONG_WINC UNICODE_WINDOWS
|
||||
```
|
||||
|
||||
|
||||
## 追加のカスタマイズ
|
||||
|
||||
Unicode は大規模で多目的な機能のため、システムでより適切に動作するようにカスタマイズできるオプションが幾つかあります。
|
||||
|
||||
### 入力関数の開始と終了
|
||||
|
||||
プラットフォームで Unicode 入力を開始および終了する機能は、ローカルで上書きできます。可能な用途には、デフォルトキーを使用しない場合の入力モードの挙動のカスタマイズ、あるいは Unicode 入力への視覚/音声フィードバックの追加があります。
|
||||
|
||||
* `void unicode_input_start(void)` – これはプラットフォームに Unicode 入力モードの入力を指示する初期シーケンスを送信します。例えば、Windows では左 Alt キーの後に Num+ を押したままにし、Linux では `UNICODE_KEY_LNX` の組み合わせ(デフォルト: Ctrl+Shift+U) を押します。
|
||||
* `void unicode_input_finish(void)` – これは、例えば Space を押すか Alt キーを放すなどして、Unicode 入力モードを終了するために呼ばれます。
|
||||
|
||||
[`process_unicode_common.c`](https://github.com/qmk/qmk_firmware/blob/master/quantum/process_keycode/process_unicode_common.c) でこれらの関数のデフォルトの実装を見つけることができます。
|
||||
|
||||
### 入力キーの設定
|
||||
|
||||
`config.h` に対応する定義を追加することで、macOS、Linux、WinCompose で Unicode 入力を引き起こすために使われるキーをカスタマイズできます。デフォルト値はプラットフォームのデフォルト設定に一致するため、Unicode 入力が動作しない、あるいは(例えば左あるいは右 Alt を解放するために)異なるキーを使いたい場合以外はこれを変更する必要はありません。
|
||||
|
||||
| 定義 | 型 | 既定値 | 例 |
|
||||
|--------------------|------------|--------------------|---------------------------------------------|
|
||||
| `UNICODE_KEY_MAC` | `uint8_t` | `KC_LALT` | `#define UNICODE_KEY_MAC KC_RALT` |
|
||||
| `UNICODE_KEY_LNX` | `uint16_t` | `LCTL(LSFT(KC_U))` | `#define UNICODE_KEY_LNX LCTL(LSFT(KC_E))` |
|
||||
| `UNICODE_KEY_WINC` | `uint8_t` | `KC_RALT` | `#define UNICODE_KEY_WINC KC_RGUI` |
|
||||
|
||||
|
||||
## Unicode 文字列の送信
|
||||
|
||||
QMK は、Unicode 入力をプログラムでホストに送信できるようにする幾つかの関数を提供します:
|
||||
|
||||
### `send_unicode_string()`
|
||||
|
||||
この関数は、`send_string()` によく似ていますが、UTF-8 文字を直接入力できます。選択された入力モードでもサポートされている場合は、全てのコードポイントをサポートします。`keymap.c` ファイルが UTF-8 エンコーディングを使ってフォーマットされていることを確認してください。
|
||||
|
||||
```c
|
||||
send_unicode_string("(ノಠ痊ಠ)ノ彡┻━┻");
|
||||
```
|
||||
|
||||
使用例には、[Macros](ja/feature_macros.md) で説明されているように、キーが押された時に Unicode 文字列を送信することが含まれます。
|
||||
|
||||
### `send_unicode_hex_string()`
|
||||
|
||||
`send_unicode_string()` に似ていますが、文字は Unicode コードポイントで表され、16進数で記述され、空白で区切られています。例えば、上記のちゃぶ台返しは以下で表されます:
|
||||
|
||||
```c
|
||||
send_unicode_hex_string("0028 30CE 0CA0 75CA 0CA0 0029 30CE 5F61 253B 2501 253B");
|
||||
```
|
||||
|
||||
[このサイト](https://r12a.github.io/app-conversion/)で結果を "Hex/UTF-32" で受け取ることで、Unicode 文字列をこの形式に簡単に変換できます。
|
||||
|
||||
|
||||
## 追加の言語サポート
|
||||
|
||||
`quantum/keymap_extras` には、様々な言語ファイルがあります — これらは Colemak または BÉPO のような代替レイアウトのファイルと同じように動作します。これらの言語ヘッダのいずれかを `#include` すると、その言語/国のレイアウトに固有のキーコードにアクセスできます。このようなキーコードは、2文字の国/言語コードの後に、アンダースコアとキーが対応する4文字の略語が続くことで定義されます。例えば、キーマップに `keymap_french.h` を含め、`FR_UGRV` を使うと、ネイティブのフランス語 AZERTY レイアウトを使うシステムで入力すると、`ù` が出力されます。
|
||||
|
||||
マシンで使うプライマリシステムレイアウトが US ANSI と異なる場合、これらの言語固有のキーコードを使うと、QMK キーマップが実際に画面に出力されるものとより一致するようになります。ただし、これらのキーコードは、内部の対応するデフォルトの US キーコードのエイリアスに過ぎず、キーボードで使われる HID プロトコル自体は本質的に US ANSI に基づいていることに注意してください。
|
||||
|
||||
|
||||
## Windows での国際文字
|
||||
|
||||
### AutoHotkey
|
||||
|
||||
この方法はキーボード自体で Unicode サポートを必要としませんが、代わりにバックグラウンドで [AutoHotkey](https://autohotkey.com) が実行されていることを当てにします。
|
||||
|
||||
最初にプログラムで使われていないモディファイアの組み合わせを選択する必要があります。
|
||||
Ctrl+Alt+Win はあまり広く使われていないため、これに最適なはずです。
|
||||
mod-tab コンボ `LCAG_T` 用に定義されたマクロがあります。
|
||||
この mod-tab マクロをキーボードのキーに追加します。例えば: `LCAG_T(KC_TAB)`。
|
||||
これにより、キーを押してすぐ放すとキーはタブキーのように振る舞いますが、他のキーと一緒に使うとモディファイアに変わります。
|
||||
|
||||
AutoHotkey のデフォルトのスクリプトで、カスタムホットキーを定義できます。
|
||||
|
||||
<^<!<#a::Send, ä
|
||||
<^<!<#<+a::Send, Ä
|
||||
|
||||
上のホットキーは、CtrlAltGui と CtrlAltGuiShift + 文字 a の組み合わせです。
|
||||
この組み合わせが押されると、AutoHotkey は `Send, ` の右側にあるテキストを挿入します。
|
||||
|
||||
### 米国インターナショナル
|
||||
|
||||
システム上で米国インターナショナルレイアウトを有効にすると、文字にアクセントをつけるために区切り文字を使います。例えば、"\`a" は à になります。
|
||||
これを有効にする方法は[ここ](https://support.microsoft.com/en-us/help/17424/windows-change-keyboard-layout)で見つかります。
|
|
@ -20,162 +20,109 @@
|
|||
|
||||
const is31_led g_is31_leds[LED_DRIVER_LED_COUNT] = {
|
||||
/* Refer to IS31 manual for these locations
|
||||
* driver
|
||||
* | LED address
|
||||
* | | */
|
||||
{0, C1_1}, // k00 KC_GESC
|
||||
{0, C1_2}, // k01 KC_1
|
||||
{0, C1_3}, // k02 KC_2
|
||||
{0, C1_4}, // k03 KC_3
|
||||
{0, C1_5}, // k04 KC_4
|
||||
{0, C1_6}, // k05 KC_5
|
||||
{0, C1_7}, // k06 KC_6
|
||||
{0, C1_8}, // k07 KC_7
|
||||
{0, C1_9}, // k50 KC_8
|
||||
{0, C1_10}, // k51 KC_9
|
||||
{0, C1_11}, // k52 KC_0
|
||||
{0, C1_12}, // k53 KC_MINS
|
||||
{0, C1_13}, // k54 KC_EQL
|
||||
{0, C1_14}, // k55 KC_BSPC
|
||||
{0, C1_15}, // k57 KC_PGUP
|
||||
{0, C2_1}, // k10 KC_TAB
|
||||
{0, C2_2}, // k11 KC_Q
|
||||
{0, C2_3}, // k12 KC_W
|
||||
{0, C2_4}, // k13 KC_E
|
||||
{0, C2_5}, // k14 KC_R
|
||||
{0, C2_6}, // k15 KC_T
|
||||
{0, C2_7}, // k16 KC_Y
|
||||
{0, C2_8}, // k17 KC_U
|
||||
{0, C2_9}, // k60 KC_I
|
||||
{0, C2_10}, // k61 KC_O
|
||||
{0, C2_11}, // k62 KC_P
|
||||
{0, C2_12}, // k63 KC_LBRC
|
||||
{0, C2_13}, // k64 KC_RBRC
|
||||
{0, C2_14}, // k65 KC_BSLS
|
||||
{0, C2_15}, // k67 KC_PGDN
|
||||
{0, C3_1}, // k20 KC_CAPS
|
||||
{0, C3_2}, // k21 KC_A
|
||||
{0, C3_3}, // k22 KC_S
|
||||
{0, C3_4}, // k23 KC_D
|
||||
{0, C3_5}, // k24 KC_F
|
||||
{0, C3_6}, // k25 KC_G
|
||||
{0, C3_7}, // k26 KC_H
|
||||
{0, C3_8}, // k27 KC_J
|
||||
{0, C3_9}, // k70 KC_K
|
||||
{0, C3_10}, // k71 KC_L
|
||||
{0, C3_11}, // k72 KC_SCLN
|
||||
{0, C3_12}, // k73 KC_QUOT
|
||||
{0, C3_14}, // k75 KC_ENT
|
||||
{0, C4_1}, // k30 KC_LSFT
|
||||
{0, C4_3}, // k32 KC_Z
|
||||
{0, C4_4}, // k33 KC_X
|
||||
{0, C4_5}, // k34 KC_C
|
||||
{0, C4_6}, // k35 KC_V
|
||||
{0, C4_7}, // k36 KC_B
|
||||
{0, C4_8}, // k37 KC_N
|
||||
{0, C4_9}, // k80 KC_M
|
||||
{0, C4_10}, // k81 KC_COMM
|
||||
{0, C4_11}, // k82 KC_DOT
|
||||
{0, C4_12}, // k83 KC_SLSH
|
||||
{0, C4_13}, // k85 KC_RSFT
|
||||
{0, C4_14}, // k86 KC_UP
|
||||
{0, C5_1}, // k40 KC_LCTL
|
||||
{0, C5_2}, // k41 KC_LGUI
|
||||
{0, C5_3}, // k42 KC_LALT
|
||||
{0, C5_4}, // Unassociated between LALT and SPACE_2.75
|
||||
{0, C5_5}, // k45 KC_SPC SPACE_2.75
|
||||
{0, C5_6}, // k45 KC_SPC SPACE_6.75
|
||||
{0, C5_7}, // k46 KC_SPC SPACE_2.25
|
||||
{0, C5_8}, // Unassociated between SPACE_2.25 and SPACE_1.25
|
||||
{0, C5_9}, // k90 KC_RGUI
|
||||
{0, C5_10}, // k92 KC_RALT
|
||||
{0, C5_11}, // k93 MO(_FL)
|
||||
{0, C5_12}, // k94 KC_RCTL
|
||||
{0, C5_13}, // k95 KC_LEFT
|
||||
{0, C5_14}, // k96 KC_DOWN
|
||||
{0, C5_15} // k97 KC_RGHT
|
||||
* driver
|
||||
* | LED address
|
||||
* | | */
|
||||
{ 0, C1_1 }, // k00 KC_GESC
|
||||
{ 0, C1_2 }, // k01 KC_1
|
||||
{ 0, C1_3 }, // k02 KC_2
|
||||
{ 0, C1_4 }, // k03 KC_3
|
||||
{ 0, C1_5 }, // k04 KC_4
|
||||
{ 0, C1_6 }, // k05 KC_5
|
||||
{ 0, C1_7 }, // k06 KC_6
|
||||
{ 0, C1_8 }, // k07 KC_7
|
||||
{ 0, C1_9 }, // k50 KC_8
|
||||
{ 0, C1_10 }, // k51 KC_9
|
||||
{ 0, C1_11 }, // k52 KC_0
|
||||
{ 0, C1_12 }, // k53 KC_MINS
|
||||
{ 0, C1_13 }, // k54 KC_EQL
|
||||
{ 0, C1_14 }, // k55 KC_BSPC
|
||||
{ 0, C1_15 }, // k57 KC_PGUP
|
||||
{ 0, C2_1 }, // k10 KC_TAB
|
||||
{ 0, C2_2 }, // k11 KC_Q
|
||||
{ 0, C2_3 }, // k12 KC_W
|
||||
{ 0, C2_4 }, // k13 KC_E
|
||||
{ 0, C2_5 }, // k14 KC_R
|
||||
{ 0, C2_6 }, // k15 KC_T
|
||||
{ 0, C2_7 }, // k16 KC_Y
|
||||
{ 0, C2_8 }, // k17 KC_U
|
||||
{ 0, C2_9 }, // k60 KC_I
|
||||
{ 0, C2_10 }, // k61 KC_O
|
||||
{ 0, C2_11 }, // k62 KC_P
|
||||
{ 0, C2_12 }, // k63 KC_LBRC
|
||||
{ 0, C2_13 }, // k64 KC_RBRC
|
||||
{ 0, C2_14 }, // k65 KC_BSLS
|
||||
{ 0, C2_15 }, // k67 KC_PGDN
|
||||
{ 0, C3_1 }, // k20 KC_CAPS
|
||||
{ 0, C3_2 }, // k21 KC_A
|
||||
{ 0, C3_3 }, // k22 KC_S
|
||||
{ 0, C3_4 }, // k23 KC_D
|
||||
{ 0, C3_5 }, // k24 KC_F
|
||||
{ 0, C3_6 }, // k25 KC_G
|
||||
{ 0, C3_7 }, // k26 KC_H
|
||||
{ 0, C3_8 }, // k27 KC_J
|
||||
{ 0, C3_9 }, // k70 KC_K
|
||||
{ 0, C3_10 }, // k71 KC_L
|
||||
{ 0, C3_11 }, // k72 KC_SCLN
|
||||
{ 0, C3_12 }, // k73 KC_QUOT
|
||||
{ 0, C3_14 }, // k75 KC_ENT
|
||||
{ 0, C4_1 }, // k30 KC_LSFT
|
||||
{ 0, C4_3 }, // k32 KC_Z
|
||||
{ 0, C4_4 }, // k33 KC_X
|
||||
{ 0, C4_5 }, // k34 KC_C
|
||||
{ 0, C4_6 }, // k35 KC_V
|
||||
{ 0, C4_7 }, // k36 KC_B
|
||||
{ 0, C4_8 }, // k37 KC_N
|
||||
{ 0, C4_9 }, // k80 KC_M
|
||||
{ 0, C4_10 }, // k81 KC_COMM
|
||||
{ 0, C4_11 }, // k82 KC_DOT
|
||||
{ 0, C4_12 }, // k83 KC_SLSH
|
||||
{ 0, C4_13 }, // k85 KC_RSFT
|
||||
{ 0, C4_14 }, // k86 KC_UP
|
||||
{ 0, C5_1 }, // k40 KC_LCTL
|
||||
{ 0, C5_2 }, // k41 KC_LGUI
|
||||
{ 0, C5_3 }, // k42 KC_LALT
|
||||
{ 0, C5_4 }, // Unassociated between LALT and SPACE_2.75
|
||||
{ 0, C5_5 }, // k45 KC_SPC SPACE_2.75
|
||||
{ 0, C5_6 }, // k45 KC_SPC SPACE_6.75
|
||||
{ 0, C5_7 }, // k46 KC_SPC SPACE_2.25
|
||||
{ 0, C5_8 }, // Unassociated between SPACE_2.25 and SPACE_1.25
|
||||
{ 0, C5_9 }, // k90 KC_RGUI
|
||||
{ 0, C5_10 }, // k92 KC_RALT
|
||||
{ 0, C5_11 }, // k93 MO(_FL)
|
||||
{ 0, C5_12 }, // k94 KC_RCTL
|
||||
{ 0, C5_13 }, // k95 KC_LEFT
|
||||
{ 0, C5_14 }, // k96 KC_DOWN
|
||||
{ 0, C5_15 } // k97 KC_RGHT
|
||||
};
|
||||
|
||||
const led_matrix g_leds[LED_DRIVER_LED_COUNT] = {
|
||||
|
||||
/*{row | col << 4}
|
||||
| LED_ROW_COL(row, col)
|
||||
| | modifier
|
||||
| | | */
|
||||
{{0|(1<<4)}, {0, 0}, 1}, // k00 KC_GESC
|
||||
{{0|(2<<4)}, {14.45, 0}, 0}, // k01 KC_1
|
||||
{{0|(3<<4)}, {28.9, 0}, 0}, // k02 KC_2
|
||||
{{0|(4<<4)}, {43.35, 0}, 0}, // k03 KC_3
|
||||
{{0|(5<<4)}, {57.8, 0}, 0}, // k04 KC_4
|
||||
{{0|(6<<4)}, {72.25, 0}, 0}, // k05 KC_5
|
||||
{{0|(7<<4)}, {86.7, 0}, 0}, // k06 KC_6
|
||||
{{0|(8<<4)}, {101.2, 0}, 0}, // k07 KC_7
|
||||
{{0|(9<<4)}, {115.6, 0}, 0}, // k50 KC_8
|
||||
{{0|(10<<4)}, {130, 0}, 0}, // k51 KC_9
|
||||
{{0|(11<<4)}, {144.5, 0}, 0}, // k52 KC_0
|
||||
{{0|(12<<4)}, {159, 0}, 0}, // k53 KC_MINS
|
||||
{{0|(13<<4)}, {173.4, 0}, 0}, // k54 KC_EQL
|
||||
{{0|(14<<4)}, {195.1, 0}, 1}, // k55 KC_BSPC
|
||||
{{0|(15<<4)}, {224, 0}, 1}, // k57 KC_PGUP
|
||||
|
||||
{{1|(0<<4)}, {3.6125, 16}, 1}, // k10 KC_TAB
|
||||
{{1|(1<<4)}, {21.675, 16}, 0}, // k11 KC_Q
|
||||
{{1|(2<<4)}, {36.125, 16}, 0}, // k12 KC_W
|
||||
{{1|(3<<4)}, {50.575, 16}, 0}, // k13 KC_E
|
||||
{{1|(4<<4)}, {65.025, 16}, 0}, // k14 KC_R
|
||||
{{1|(5<<4)}, {79.475, 16}, 0}, // k15 KC_T
|
||||
{{1|(6<<4)}, {93.925, 16}, 0}, // k16 KC_Y
|
||||
{{1|(7<<4)}, {108.375, 16}, 0}, // k17 KC_U
|
||||
{{1|(8<<4)}, {122.825, 16}, 0}, // k60 KC_I
|
||||
{{1|(9<<4)}, {137.275, 16}, 0}, // k61 KC_O
|
||||
{{1|(10<<4)}, {151.725, 16}, 0}, // k62 KC_P
|
||||
{{1|(11<<4)}, {166.175, 16}, 0}, // k63 KC_LBRC
|
||||
{{1|(12<<4)}, {180.625, 16}, 0}, // k64 KC_RBRC
|
||||
{{1|(13<<4)}, {198.6875, 16}, 1}, // k65 KC_BSLS
|
||||
{{1|(14<<4)}, {224, 16}, 1}, // k67 KC_PGDN
|
||||
|
||||
{{2|(0<<4)}, {5.41875, 32}, 1}, // k20 KC_CAPS
|
||||
{{2|(1<<4)}, {25.2875, 32}, 0}, // k21 KC_A
|
||||
{{2|(2<<4)}, {39.7375, 32}, 0}, // k22 KC_S
|
||||
{{2|(3<<4)}, {54.1875, 32}, 0}, // k23 KC_D
|
||||
{{2|(4<<4)}, {68.6375, 32}, 0}, // k24 KC_F
|
||||
{{2|(5<<4)}, {83.0875, 32}, 0}, // k25 KC_G
|
||||
{{2|(6<<4)}, {97.5375, 32}, 0}, // k26 KC_H
|
||||
{{2|(7<<4)}, {111.9875, 32}, 0}, // k27 KC_J
|
||||
{{2|(8<<4)}, {126.4375, 32}, 0}, // k70 KC_K
|
||||
{{2|(9<<4)}, {140.8875, 32}, 0}, // k71 KC_L
|
||||
{{2|(10<<4)}, {155.3375, 32}, 0}, // k72 KC_SCLN
|
||||
{{2|(11<<4)}, {169.7875, 32}, 0}, // k73 KC_QUOT
|
||||
{{2|(12<<4)}, {184.2375, 32}, 1}, // k75 KC_ENT
|
||||
|
||||
{{3|(0<<4)}, {16.25625, 48}, 1}, // k30 KC_LSFT
|
||||
{{3|(1<<4)}, {32.5125, 48}, 0}, // k32 KC_Z
|
||||
{{3|(2<<4)}, {46.9625, 48}, 0}, // k33 KC_X
|
||||
{{3|(3<<4)}, {61.4125, 48}, 0}, // k34 KC_C
|
||||
{{3|(4<<4)}, {75.8625, 48}, 0}, // k35 KC_V
|
||||
{{3|(5<<4)}, {90.3125, 48}, 0}, // k36 KC_B
|
||||
{{3|(6<<4)}, {104.7625, 48}, 0}, // k37 KC_N
|
||||
{{3|(7<<4)}, {119.2125, 48}, 0}, // k80 KC_M
|
||||
{{3|(8<<4)}, {133.6625, 48}, 0}, // k81 KC_COMM
|
||||
{{3|(9<<4)}, {148.1125, 48}, 0}, // k82 KC_DOT
|
||||
{{3|(10<<4)}, {162.5625, 48}, 0}, // k83 KC_SLSH
|
||||
{{3|(11<<4)}, {187.85, 48}, 1}, // k85 KC_RSFT
|
||||
{{3|(12<<4)}, {209.525, 48}, 1}, // k86 KC_UP
|
||||
|
||||
{{4|(0<<4)}, {9.03125, 64}, 1}, // k40 KC_LCTL
|
||||
{{4|(1<<4)}, {27.09375, 64}, 1}, // k41 KC_LGUI
|
||||
{{4|(2<<4)}, {45.15625, 64}, 1}, // k42 KC_LALT
|
||||
{{4|(3<<4)}, {59.45, 64}, 1}, // Unassociated between LALT and SPACE_2.75
|
||||
{{4|(4<<4)}, {73.9, 64}, 1}, // k45 KC_SPC SPACE_2.75
|
||||
{{4|(5<<4)}, {88.35, 64}, 1}, // k45 KC_SPC SPACE_6.25
|
||||
{{4|(6<<4)}, {102.8, 64}, 1}, // k46 KC_SPC SPACE_2.25
|
||||
{{4|(7<<4)}, {117.40625, 64}, 1}, // Unassociated between SPACE_2.25 and SPACE_2.75
|
||||
{{4|(8<<4)}, {135.46875, 64}, 1}, // k90 KC_RGUI
|
||||
{{4|(9<<4)}, {153.53125, 64}, 1}, // k92 KC_RALT
|
||||
{{4|(10<<4)}, {167.98125, 64}, 1}, // k93 MO(_FL)
|
||||
{{4|(11<<4)}, {186.04375, 64}, 1}, // k94 KC_RCTL
|
||||
{{4|(12<<4)}, {195.075, 64}, 1}, // k95 KC_LEFT
|
||||
{{4|(13<<4)}, {209.525, 64}, 1}, // k96 KC_DOWN
|
||||
{{4|(14<<4)}, {224, 64}, 1} // k97 KC_RGHT
|
||||
led_config_t g_led_config = {
|
||||
{
|
||||
// Key Matrix to LED Index
|
||||
{ 0, 1, 2, 3, 4, 5, 6, 7 },
|
||||
{ 15, 16, 17, 18, 19, 20, 21, 22 },
|
||||
{ 30, 31, 32, 33, 34, 35, 36, 37 },
|
||||
{ 43, NO_LED, 44, 45, 46, 47, 48, 49 },
|
||||
{ 56, 57, 58, NO_LED, NO_LED, 60, 61, NO_LED },
|
||||
{ 8, 9, 10, 11, 12, 13, NO_LED, 14 },
|
||||
{ 23, 24, 25, 26, 27, 28, NO_LED, 29 },
|
||||
{ 38, 39, 40, 41, NO_LED, 42, NO_LED, NO_LED },
|
||||
{ 50, 51, 52, 53, NO_LED, 54, 55, NO_LED },
|
||||
{ 64, NO_LED, 65, 66, 67, 68, 69, 70 }
|
||||
}, {
|
||||
// LED Index to Physical Position
|
||||
{ 0, 0 }, { 15, 0 }, { 29, 0 }, { 43, 0 }, { 58, 0 }, { 72, 0 }, { 87, 0 }, { 101, 0 }, { 116, 0 }, { 130, 0 }, { 145, 0 }, { 159, 0 }, { 173, 0 }, { 195, 0 }, { 224, 0 },
|
||||
{ 4, 16 }, { 22, 16 }, { 36, 16 }, { 51, 16 }, { 65, 16 }, { 80, 16 }, { 94, 16 }, { 108, 16 }, { 123, 16 }, { 137, 16 }, { 152, 16 }, { 166, 16 }, { 181, 16 }, { 199, 16 }, { 224, 16 },
|
||||
{ 5, 32 }, { 25, 32 }, { 40, 32 }, { 54, 32 }, { 69, 32 }, { 83, 32 }, { 98, 32 }, { 112, 32 }, { 126, 32 }, { 141, 32 }, { 155, 32 }, { 170, 32 }, { 184, 32 },
|
||||
{ 16, 48 }, { 33, 48 }, { 47, 48 }, { 61, 48 }, { 76, 48 }, { 90, 48 }, { 105, 48 }, { 119, 48 }, { 134, 48 }, { 148, 48 }, { 163, 48 }, { 188, 48 }, { 210, 48 },
|
||||
{ 9, 64 }, { 27, 64 }, { 45, 64 }, { 60, 64 }, { 74, 64 }, { 88, 64 }, { 103, 64 }, { 117, 64 }, { 136, 64 }, { 154, 64 }, { 168, 64 }, { 186, 64 }, { 195, 64 }, { 210, 64 }, { 224, 64 }
|
||||
}, {
|
||||
// LED Index to Flag
|
||||
1, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 1, 1,
|
||||
1, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 1, 1,
|
||||
1, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 1,
|
||||
1, 4, 4, 4, 4, 4, 4, 4, 4, 4, 4, 1, 1,
|
||||
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
|
||||
}
|
||||
};
|
||||
#endif
|
||||
|
|
|
@ -26,13 +26,12 @@ void on_all_leds(void);
|
|||
|
||||
// This a shortcut to help you visually see your layout.
|
||||
#define LAYOUT_tkl_ansi( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1E, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, K2E, K2F, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3D, K3E, K3F, \
|
||||
K40, K42, K43, K44, K45, K46, K47, K48, K49, K4A, K4B, K4D, K4E, K4F, \
|
||||
K50, K51, K52, K56, K5A, K5B, K5C, K5D, K5E, K5F, \
|
||||
K6F \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1E, K2E, K2F, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, K3D, K3E, K3F, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K4E, \
|
||||
K40, K42, K43, K44, K45, K46, K47, K48, K49, K4A, K4B, K4D, K4F, \
|
||||
K50, K51, K52, K56, K5A, K5B, K5C, K5D, K5E, K5F, K6F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, XXX, K1E, K1F }, \
|
||||
|
@ -43,6 +42,7 @@ void on_all_leds(void);
|
|||
{ XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, XXX, K6F } \
|
||||
}
|
||||
|
||||
|
||||
// This a shortcut to help you visually see your layout.
|
||||
#define LAYOUT_tkl_iso( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, K0F, \
|
||||
|
|
|
@ -45,13 +45,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_BASE] = LAYOUT_tkl_ansi( /* Base Layer */
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SLCK, KC_PAUS,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_INS, KC_HOME,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_ENT, KC_UP,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, MO(_FUNC),KC_APP, KC_RCTL, KC_LEFT, KC_DOWN,
|
||||
KC_RGHT
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SLCK, KC_PAUS,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, MO(_FUNC),KC_APP, KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
/* Keymap _FUNC: Function Layer
|
||||
* ,-----------------------------------------------------------. ,--------------.
|
||||
|
@ -69,13 +68,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_FUNC] = LAYOUT_tkl_ansi( /* Function Layer */
|
||||
_______, KC_MPLY, KC_MSTP, KC_MRWD, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, KC_TGUI, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______
|
||||
_______, KC_MPLY, KC_MSTP, KC_MRWD, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, KC_TGUI, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
)
|
||||
};
|
||||
|
||||
|
|
|
@ -67,13 +67,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_WBL] = LAYOUT_tkl_ansi( /* Windows Base Layer */
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SLCK, KC_PAUS,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_INS, KC_HOME,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_ENT, KC_UP,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, KC_WFN, KC_APP, KC_RCTL, KC_LEFT, KC_DOWN,
|
||||
KC_RGHT
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SLCK, KC_PAUS,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, KC_WFN, KC_APP, KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
/* Keymap _WFL: Windows Function Layer
|
||||
* ,-----------------------------------------------------------. ,--------------.
|
||||
|
@ -91,13 +90,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_WFL] = LAYOUT_tkl_ansi( /* Windows First Layer */
|
||||
_______, KC_MPLY, KC_MSTP, KC_MRWD, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, KC_TGUI, _______, _______, _______, _______, MO_WSL, _______, _______, _______,
|
||||
_______
|
||||
_______, KC_MPLY, KC_MSTP, KC_MRWD, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, KC_TGUI, _______, _______, _______, _______, MO_WSL, _______, _______, _______, _______
|
||||
),
|
||||
/* Keymap _WSL: Windows System Layer
|
||||
* ,-----------------------------------------------------------. ,--------------.
|
||||
|
@ -115,13 +113,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_WSL] = LAYOUT_tkl_ansi( /* Windows Second / System Layer */
|
||||
RESET, KC_SLEP, XXXXXXX, XXXXXXX, KC_PWR, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, DF_W2MBL, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, DEBUG, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, _______, _______, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX
|
||||
RESET, KC_SLEP, XXXXXXX, XXXXXXX, KC_PWR, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, DF_W2MBL, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, DEBUG, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, _______, _______, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX
|
||||
),
|
||||
|
||||
/* Keymap _MBL: Mac Base Layer (Alternate Layout)
|
||||
|
@ -140,13 +137,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_MBL] = LAYOUT_tkl_ansi( /* Mac Base Layer */
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_F13, KC_F14, KC_F15,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_INS, KC_HOME,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_ENT, KC_UP,
|
||||
KC_LCTL, KC_LALT, KC_LGUI, KC_SPC, KC_RGUI, KC_RALT, KC_MFN, KC_RCTL, KC_LEFT, KC_DOWN,
|
||||
KC_RGHT
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_F13, KC_F14, KC_F15,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP,
|
||||
KC_LCTL, KC_LALT, KC_LGUI, KC_SPC, KC_RGUI, KC_RALT, KC_MFN, KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
/* Keymap _MFL: Mac Function Layer
|
||||
* ,-----------------------------------------------------------. ,--------------.
|
||||
|
@ -164,13 +160,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_MFL] = LAYOUT_tkl_ansi( /* Mac First Layer */
|
||||
_______, KC_BRID, KC_BRIU, _______, _______, _______, _______, KC_MRWD, KC_MPLY, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, KC_TMED, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, MO_MSL, _______, _______, _______, _______,
|
||||
_______
|
||||
_______, KC_BRID, KC_BRIU, _______, _______, _______, _______, KC_MRWD, KC_MPLY, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, KC_TMED, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, MO_MSL, _______, _______, _______, _______, _______
|
||||
),
|
||||
/* Keymap _MSL: Mac System Layer
|
||||
* ,-----------------------------------------------------------. ,--------------.
|
||||
|
@ -188,13 +183,12 @@ const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
|||
* `-----------------------------------------------------------' `-------------'
|
||||
*/
|
||||
[_MSL] = LAYOUT_tkl_ansi( /* Mac Second / System Layer */
|
||||
RESET, XXXXXXX, XXXXXXX, XXXXXXX, KC_SLEP, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, DF_M2WBL, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, DEBUG, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, _______, _______, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX
|
||||
RESET, XXXXXXX, XXXXXXX, XXXXXXX, KC_SLEP, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, DF_M2WBL, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, DEBUG, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, _______, _______, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX
|
||||
)
|
||||
};
|
||||
|
||||
|
|
|
@ -109,43 +109,39 @@ const uint32_t PROGMEM unicode_map[] = {
|
|||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[_BL] = LAYOUT_tkl_ansi( /* Base Layer */
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SLCK, KC_PAUS,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_INS, KC_HOME,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_ENT, KC_UP,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, MO(_FL), KC_APP, KC_RCTL, KC_LEFT, KC_DOWN,
|
||||
KC_RGHT
|
||||
KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SLCK, KC_PAUS,
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, MO(_FL), KC_APP, KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
|
||||
[_FL] = LAYOUT_tkl_ansi( /* First Layer */
|
||||
KC_TRNS, KC_MPLY, KC_MSTP, KC_MRWD, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_CALC, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_PDTP,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_TASK, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_MSEL, XXXXXXX, XXXXXXX, XXXXXXX, KC_ADTP, KC_STOP,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_FIND, KC_WHOM, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_DDTP, KC_SEND, KC_NDTP,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, KC_MYCM, XXXXXXX, XXXXXXX, XXXXXXX, KC_MAIL, XXXXXXX, XXXXXXX, XXXXXXX, KC_MENU, XXXXXXX, KC_WINU,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, MO(_SL), KC_TRNS, MO(_UL), XXXXXXX, KC_WINL, KC_WIND,
|
||||
KC_WINR
|
||||
KC_TRNS, KC_MPLY, KC_MSTP, KC_MRWD, KC_MFFD, KC_MUTE, KC_VOLD, KC_VOLU, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_CALC, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_ADTP, KC_STOP, KC_PDTP,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_TASK, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_MSEL, XXXXXXX, XXXXXXX, XXXXXXX, KC_DDTP, KC_SEND, KC_NDTP,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_FIND, KC_WHOM, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, KC_MYCM, XXXXXXX, XXXXXXX, XXXXXXX, KC_MAIL, XXXXXXX, XXXXXXX, XXXXXXX, KC_MENU, KC_WINU,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, MO(_SL), KC_TRNS, MO(_UL), XXXXXXX, KC_WINL, KC_WIND, KC_WINR
|
||||
),
|
||||
|
||||
[_SL] = LAYOUT_tkl_ansi( /* Second Layer */
|
||||
XXXXXXX, KC_SLEP, XXXXXXX, XXXXXXX, KC_PWR, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_TRNS, KC_TRNS, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX
|
||||
XXXXXXX, KC_SLEP, XXXXXXX, XXXXXXX, KC_PWR, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_TRNS, KC_TRNS, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX
|
||||
),
|
||||
|
||||
[_UL] = LAYOUT_tkl_ansi( /* Unicode Layer */
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
X(APPR), X(NEQU), X(POW2), X(POW3), XXXXXXX, XXXXXXX, X(BSQR), X(WSQR), X(INFI), X(BDOT), X(WDOT), XXXXXXX, X(PONE), XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, X(SUM), XXXXXXX, X(MYU), X(SAME), XXXXXXX, X(OHM), X(DLAR), X(DRAR), XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, X(SQRT), X(DELT), XXXXXXX, XXXXXXX, X(THFR), XXXXXXX, XXXXXXX, XXXXXXX, X(THET), X(DEGR), XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, X(COPY), XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, X(LTOE), X(MTOE), X(DIV), XXXXXXX, XXXXXXX, X(UARR),
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_TRNS, KC_TRNS, XXXXXXX, X(LARR), X(DARR),
|
||||
X(RARR)
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
X(APPR), X(NEQU), X(POW2), X(POW3), XXXXXXX, XXXXXXX, X(BSQR), X(WSQR), X(INFI), X(BDOT), X(WDOT), XXXXXXX, X(PONE), XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, X(SUM), XXXXXXX, X(MYU), X(SAME), XXXXXXX, X(OHM), X(DLAR), X(DRAR), XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, X(SQRT), X(DELT), XXXXXXX, XXXXXXX, X(THFR), XXXXXXX, XXXXXXX, XXXXXXX, X(THET), X(DEGR), XXXXXXX,
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, X(COPY), XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, X(LTOE), X(MTOE), X(DIV), XXXXXXX, X(UARR),
|
||||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_TRNS, KC_TRNS, XXXXXXX, X(LARR), X(DARR), X(RARR)
|
||||
)
|
||||
};
|
||||
|
||||
|
|
|
@ -19,9 +19,14 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
|
||||
#define EE_HANDS
|
||||
|
||||
#define NO_ACTION_MACRO
|
||||
#define NO_ACTION_FUNCTION
|
||||
|
||||
#undef RGBLED_NUM
|
||||
#define RGBLIGHT_ANIMATIONS
|
||||
#define RGBLED_NUM 12
|
||||
#define RGBLIGHT_HUE_STEP 8
|
||||
#define RGBLIGHT_SAT_STEP 8
|
||||
#define RGBLIGHT_VAL_STEP 8
|
||||
|
||||
#define USB_POLLING_INTERVAL_MS 1
|
||||
|
|
|
@ -0,0 +1,19 @@
|
|||
/* Copyright 2021 Atsushi Nagase
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
// place overrides here
|
|
@ -0,0 +1,45 @@
|
|||
/* Copyright 2021 Atsushi Nagase
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
enum meishi2_moc_layers {
|
||||
_DEFAULT,
|
||||
_RAISE
|
||||
};
|
||||
|
||||
#define PRO_MICRO_LED_TX D5
|
||||
#define RAISE MO(_RAISE)
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[_DEFAULT] = LAYOUT( /* Base */
|
||||
RAISE, KC_B, KC_N, KC_SPC
|
||||
),
|
||||
[_RAISE] = LAYOUT( /* Raise */
|
||||
_______, KC_LEFT, KC_RGHT, LSFT(KC_S)
|
||||
)
|
||||
};
|
||||
|
||||
void matrix_init_user(void) {
|
||||
setPinOutput(PRO_MICRO_LED_TX);
|
||||
writePinHigh(PRO_MICRO_LED_TX);
|
||||
}
|
||||
|
||||
bool process_record_user(uint16_t keycode, keyrecord_t *record) {
|
||||
if (keycode == RAISE) {
|
||||
writePin(PRO_MICRO_LED_TX, !record->event.pressed);
|
||||
}
|
||||
return true;
|
||||
}
|
|
@ -0,0 +1,17 @@
|
|||
# [MOC] keymap for meishi2
|
||||
|
||||
```
|
||||
,--------- ------ ------ -----,
|
||||
| RAISE | ⏮ | ⏭ | ⏯ |
|
||||
`--------- ------ ------ -----'
|
||||
```
|
||||
|
||||
## Raise Layer
|
||||
|
||||
```
|
||||
,--------- ------ ------ -----,
|
||||
| | ⏪ | ⏩ | 🔀 |
|
||||
`--------- ------ ------ -----'
|
||||
```
|
||||
|
||||
[moc]: http://moc.daper.net/
|
|
@ -0,0 +1,67 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "config_common.h"
|
||||
|
||||
/* USB Device descriptor parameter */
|
||||
#define VENDOR_ID 0x4E4B // "NK"
|
||||
#define PRODUCT_ID 0x5048
|
||||
#define DEVICE_VER 0x0100
|
||||
#define MANUFACTURER NEO Keys
|
||||
#define PRODUCT Palette G67 Hotswap
|
||||
|
||||
/* key matrix size */
|
||||
#define MATRIX_ROWS 5
|
||||
#define MATRIX_COLS 15
|
||||
|
||||
/*
|
||||
* Keyboard Matrix Assignments
|
||||
*
|
||||
* Change this to how you wired your keyboard
|
||||
* COLS: AVR pins used for columns, left to right
|
||||
* ROWS: AVR pins used for rows, top to bottom
|
||||
* DIODE_DIRECTION: COL2ROW = COL = Anode (+), ROW = Cathode (-, marked on diode)
|
||||
* ROW2COL = ROW = Anode (+), COL = Cathode (-, marked on diode)
|
||||
*
|
||||
*/
|
||||
#define MATRIX_ROW_PINS { B0, B1, B2, B3, F7 }
|
||||
#define MATRIX_COL_PINS { C7, F6, F5, F4, F1, E6, D0, D1, D2, D3, D4, D5, D6, D7, B4 }
|
||||
|
||||
#define DIODE_DIRECTION COL2ROW
|
||||
|
||||
#if defined(RGBLIGHT_ENABLE)
|
||||
#define RGB_DI_PIN F0
|
||||
#define RGBLED_NUM 77
|
||||
#define RGBLIGHT_HUE_STEP 8
|
||||
#define RGBLIGHT_SAT_STEP 8
|
||||
#define RGBLIGHT_VAL_STEP 8
|
||||
#define RGBLIGHT_LIMIT_VAL 150 /* The maximum brightness level */
|
||||
#define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */
|
||||
/*== all animations enable ==*/
|
||||
#define RGBLIGHT_ANIMATIONS
|
||||
// /*== or choose animations ==*/
|
||||
// #define RGBLIGHT_EFFECT_BREATHING
|
||||
// #define RGBLIGHT_EFFECT_RAINBOW_MOOD
|
||||
// #define RGBLIGHT_EFFECT_RAINBOW_SWIRL
|
||||
// #define RGBLIGHT_EFFECT_SNAKE
|
||||
// #define RGBLIGHT_EFFECT_KNIGHT
|
||||
// #define RGBLIGHT_EFFECT_CHRISTMAS
|
||||
// #define RGBLIGHT_EFFECT_STATIC_GRADIENT
|
||||
// #define RGBLIGHT_EFFECT_RGB_TEST
|
||||
// #define RGBLIGHT_EFFECT_ALTERNATING
|
||||
#endif
|
|
@ -0,0 +1,17 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include "hotswap.h"
|
|
@ -0,0 +1,33 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "quantum.h"
|
||||
|
||||
#define LAYOUT_65_ansi_blocker( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1D, K1E, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2D, K2E, \
|
||||
K30, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3D, K3E, \
|
||||
K40, K41, K43, K46, K4A, K4B, K4C, K4D, K4E \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1D, K1E }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, KC_NO, K2D, K2E }, \
|
||||
{ K30, KC_NO, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3D, K3E }, \
|
||||
{ K40, K41, KC_NO, K43, KC_NO, KC_NO, K46, KC_NO, KC_NO, KC_NO, K4A, K4B, K4C, K4D, K4E }, \
|
||||
}
|
|
@ -0,0 +1,84 @@
|
|||
{
|
||||
"keyboard_name": "NEO Keys Palette G67 Hotswap",
|
||||
"url": "",
|
||||
"maintainer": "qmk",
|
||||
"width": 16,
|
||||
"height": 5,
|
||||
"layouts": {
|
||||
"LAYOUT_65_ansi_blocker": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":13.5, "y":1, "w":1.5},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2, "w":2.25},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":2.25},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.25},
|
||||
{"x":1.25, "y":4, "w":1.25},
|
||||
{"x":2.5, "y":4, "w":1.25},
|
||||
{"x":3.75, "y":4, "w":6.25},
|
||||
{"x":10, "y":4, "w":1.25},
|
||||
{"x":11.25, "y":4, "w":1.25},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,34 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[0] = LAYOUT_65_ansi_blocker(
|
||||
KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_TILD,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_PGUP,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, KC_PGDN,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, MO(1), KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
[1] = LAYOUT_65_ansi_blocker(
|
||||
_______, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, _______, KC_HOME,
|
||||
_______, RGB_TOG, KC_UP, RGB_MOD, RGB_HUI, RGB_HUD, RGB_SAI, RGB_SAD, RGB_VAI, RGB_VAD, KC_PSCR, KC_SLCK, KC_PAUS, _______, KC_END,
|
||||
_______, KC_LEFT, KC_DOWN, KC_RGHT, _______, _______, _______, _______, _______, KC_INS, KC_HOME, KC_PGUP, _______, KC_PGUP,
|
||||
_______, RESET, BL_DEC, BL_TOGG, BL_INC, KC_VOLD, KC_MUTE, KC_VOLU, _______, KC_DEL, KC_END, KC_PGDN, _______, KC_PGDN,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
};
|
|
@ -0,0 +1,49 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[0] = LAYOUT_65_ansi_blocker(
|
||||
KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_TILD,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_PGUP,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, KC_PGDN,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, MO(1), KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
[1] = LAYOUT_65_ansi_blocker(
|
||||
_______, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, _______, KC_HOME,
|
||||
_______, RGB_TOG, KC_UP, RGB_MOD, RGB_HUI, RGB_HUD, RGB_SAI, RGB_SAD, RGB_VAI, RGB_VAD, KC_PSCR, KC_SLCK, KC_PAUS, _______, KC_END,
|
||||
_______, KC_LEFT, KC_DOWN, KC_RGHT, _______, _______, _______, _______, _______, KC_INS, KC_HOME, KC_PGUP, _______, KC_PGUP,
|
||||
_______, RESET, BL_DEC, BL_TOGG, BL_INC, KC_VOLD, KC_MUTE, KC_VOLU, _______, KC_DEL, KC_END, KC_PGDN, _______, KC_PGDN,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
[2] = LAYOUT_65_ansi_blocker(
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
|
||||
),
|
||||
[3] = LAYOUT_65_ansi_blocker(
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
};
|
|
@ -0,0 +1,3 @@
|
|||
VIA_ENABLE = yes
|
||||
LTO_ENABLE = yes
|
||||
KEY_LOCK_ENABLE = no
|
|
@ -0,0 +1,21 @@
|
|||
# Palette G67 Hotswap
|
||||
|
||||
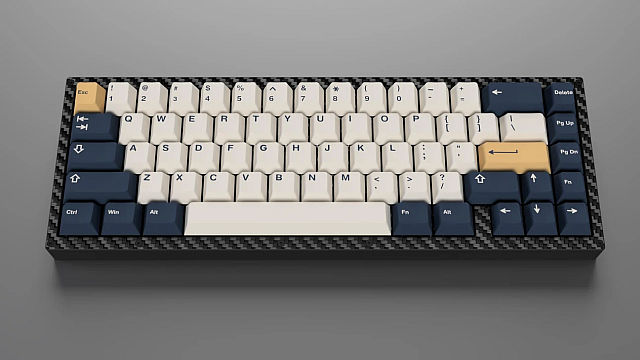
|
||||
|
||||
A blockered 65% with per-key RGB, underglow, and USB Type C.
|
||||
|
||||
* Keyboard Maintainer: [The QMK Community](https://github.com/qmk)
|
||||
* Hardware Supported: Palette G67 Hotswap (ATmega32U4)
|
||||
* Hardware Availability: [NEO Keys](https://www.neokeys.net/)
|
||||
|
||||
Make example for this keyboard (after setting up your build environment):
|
||||
|
||||
make neokeys/palette_g67/hotswap:default
|
||||
|
||||
Flashing example for this keyboard:
|
||||
|
||||
make neokeys/palette_g67/hotswap:default:flash
|
||||
|
||||
To reset the board into bootloader mode, hold the key at the top left of the keyboard while connecting the USB cable (also erases persistent settings).
|
||||
|
||||
See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
|
|
@ -0,0 +1,26 @@
|
|||
# MCU name
|
||||
MCU = atmega32u4
|
||||
|
||||
# Bootloader selection
|
||||
BOOTLOADER = atmel-dfu
|
||||
|
||||
# Build Options
|
||||
# change yes to no to disable
|
||||
#
|
||||
BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration
|
||||
MOUSEKEY_ENABLE = yes # Mouse keys
|
||||
EXTRAKEY_ENABLE = yes # Audio control and System control
|
||||
CONSOLE_ENABLE = no # Console for debug
|
||||
COMMAND_ENABLE = no # Commands for debug and configuration
|
||||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
|
||||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
|
||||
# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
|
||||
NKRO_ENABLE = no # USB Nkey Rollover
|
||||
BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality
|
||||
RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow
|
||||
BLUETOOTH_ENABLE = no # Enable Bluetooth
|
||||
AUDIO_ENABLE = no # Audio output
|
||||
|
||||
KEY_LOCK_ENABLE = yes # Enable KC_LOCK support
|
||||
|
||||
LAYOUTS = 65_ansi_blocker
|
|
@ -0,0 +1,71 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "config_common.h"
|
||||
|
||||
/* USB Device descriptor parameter */
|
||||
#define VENDOR_ID 0x4E4B // "NK"
|
||||
#define PRODUCT_ID 0x5053
|
||||
#define DEVICE_VER 0x0100
|
||||
#define MANUFACTURER NEO Keys
|
||||
#define PRODUCT Palette G67 Soldered
|
||||
|
||||
/* key matrix size */
|
||||
#define MATRIX_ROWS 5
|
||||
#define MATRIX_COLS 16
|
||||
|
||||
/*
|
||||
* Keyboard Matrix Assignments
|
||||
*
|
||||
* Change this to how you wired your keyboard
|
||||
* COLS: AVR pins used for columns, left to right
|
||||
* ROWS: AVR pins used for rows, top to bottom
|
||||
* DIODE_DIRECTION: COL2ROW = COL = Anode (+), ROW = Cathode (-, marked on diode)
|
||||
* ROW2COL = ROW = Anode (+), COL = Cathode (-, marked on diode)
|
||||
*
|
||||
*/
|
||||
#define MATRIX_ROW_PINS { B0, B1, B2, B3, F7 }
|
||||
#define MATRIX_COL_PINS { C7, F6, F5, F4, F1, E6, D0, D1, D2, D3, D4, D5, D6, D7, B5, B4 }
|
||||
|
||||
#define DIODE_DIRECTION COL2ROW
|
||||
|
||||
#define BACKLIGHT_PIN B6
|
||||
#define BACKLIGHT_BREATHING
|
||||
#define BACKLIGHT_LEVELS 10
|
||||
|
||||
#if defined(RGBLIGHT_ENABLE)
|
||||
#define RGB_DI_PIN F0
|
||||
#define RGBLED_NUM 18
|
||||
#define RGBLIGHT_HUE_STEP 8
|
||||
#define RGBLIGHT_SAT_STEP 8
|
||||
#define RGBLIGHT_VAL_STEP 8
|
||||
#define RGBLIGHT_LIMIT_VAL 150 /* The maximum brightness level */
|
||||
#define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */
|
||||
/*== all animations enable ==*/
|
||||
#define RGBLIGHT_ANIMATIONS
|
||||
// /*== or choose animations ==*/
|
||||
// #define RGBLIGHT_EFFECT_BREATHING
|
||||
// #define RGBLIGHT_EFFECT_RAINBOW_MOOD
|
||||
// #define RGBLIGHT_EFFECT_RAINBOW_SWIRL
|
||||
// #define RGBLIGHT_EFFECT_SNAKE
|
||||
// #define RGBLIGHT_EFFECT_KNIGHT
|
||||
// #define RGBLIGHT_EFFECT_CHRISTMAS
|
||||
// #define RGBLIGHT_EFFECT_STATIC_GRADIENT
|
||||
// #define RGBLIGHT_EFFECT_RGB_TEST
|
||||
// #define RGBLIGHT_EFFECT_ALTERNATING
|
||||
#endif
|
|
@ -0,0 +1,541 @@
|
|||
{
|
||||
"keyboard_name": "NEO Keys Palette G67 Soldered",
|
||||
"url": "",
|
||||
"maintainer": "qmk",
|
||||
"width": 16,
|
||||
"height": 5,
|
||||
"layouts": {
|
||||
"LAYOUT_all": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0},
|
||||
{"x":14, "y":0},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":13.5, "y":1, "w":1.5},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2},
|
||||
{"x":13.75, "y":2, "w":1.25},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":1.25},
|
||||
{"x":1.25, "y":3},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.25},
|
||||
{"x":1.25, "y":4, "w":1.25},
|
||||
{"x":2.5, "y":4, "w":1.25},
|
||||
{"x":3.75, "y":4, "w":6.25},
|
||||
{"x":10, "y":4},
|
||||
{"x":11, "y":4},
|
||||
{"x":12, "y":4},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_65_ansi": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":13.5, "y":1, "w":1.5},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2, "w":2.25},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":2.25},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.25},
|
||||
{"x":1.25, "y":4, "w":1.25},
|
||||
{"x":2.5, "y":4, "w":1.25},
|
||||
{"x":3.75, "y":4, "w":6.25},
|
||||
{"x":10, "y":4},
|
||||
{"x":11, "y":4},
|
||||
{"x":12, "y":4},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_65_ansi_blocker": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":13.5, "y":1, "w":1.5},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2, "w":2.25},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":2.25},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.25},
|
||||
{"x":1.25, "y":4, "w":1.25},
|
||||
{"x":2.5, "y":4, "w":1.25},
|
||||
{"x":3.75, "y":4, "w":6.25},
|
||||
{"x":10, "y":4, "w":1.25},
|
||||
{"x":11.25, "y":4, "w":1.25},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_65_ansi_blocker_tsangan": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":13.5, "y":1, "w":1.5},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2, "w":2.25},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":2.25},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.5},
|
||||
{"x":1.5, "y":4},
|
||||
{"x":2.5, "y":4, "w":1.5},
|
||||
{"x":4, "y":4, "w":7},
|
||||
{"x":11, "y":4, "w":1.5},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_65_iso": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2},
|
||||
{"x":13.75, "y":1, "w":1.25, "h":2},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":1.25},
|
||||
{"x":1.25, "y":3},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.25},
|
||||
{"x":1.25, "y":4, "w":1.25},
|
||||
{"x":2.5, "y":4, "w":1.25},
|
||||
{"x":3.75, "y":4, "w":6.25},
|
||||
{"x":10, "y":4},
|
||||
{"x":11, "y":4},
|
||||
{"x":12, "y":4},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_65_iso_blocker": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2},
|
||||
{"x":13.75, "y":1, "w":1.25, "h":2},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":1.25},
|
||||
{"x":1.25, "y":3},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.25},
|
||||
{"x":1.25, "y":4, "w":1.25},
|
||||
{"x":2.5, "y":4, "w":1.25},
|
||||
{"x":3.75, "y":4, "w":6.25},
|
||||
{"x":10, "y":4, "w":1.25},
|
||||
{"x":11.25, "y":4, "w":1.25},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_65_iso_blocker_tsangan": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
{"x":4, "y":0},
|
||||
{"x":5, "y":0},
|
||||
{"x":6, "y":0},
|
||||
{"x":7, "y":0},
|
||||
{"x":8, "y":0},
|
||||
{"x":9, "y":0},
|
||||
{"x":10, "y":0},
|
||||
{"x":11, "y":0},
|
||||
{"x":12, "y":0},
|
||||
{"x":13, "y":0, "w":2},
|
||||
{"x":15, "y":0},
|
||||
|
||||
{"x":0, "y":1, "w":1.5},
|
||||
{"x":1.5, "y":1},
|
||||
{"x":2.5, "y":1},
|
||||
{"x":3.5, "y":1},
|
||||
{"x":4.5, "y":1},
|
||||
{"x":5.5, "y":1},
|
||||
{"x":6.5, "y":1},
|
||||
{"x":7.5, "y":1},
|
||||
{"x":8.5, "y":1},
|
||||
{"x":9.5, "y":1},
|
||||
{"x":10.5, "y":1},
|
||||
{"x":11.5, "y":1},
|
||||
{"x":12.5, "y":1},
|
||||
{"x":15, "y":1},
|
||||
|
||||
{"x":0, "y":2, "w":1.75},
|
||||
{"x":1.75, "y":2},
|
||||
{"x":2.75, "y":2},
|
||||
{"x":3.75, "y":2},
|
||||
{"x":4.75, "y":2},
|
||||
{"x":5.75, "y":2},
|
||||
{"x":6.75, "y":2},
|
||||
{"x":7.75, "y":2},
|
||||
{"x":8.75, "y":2},
|
||||
{"x":9.75, "y":2},
|
||||
{"x":10.75, "y":2},
|
||||
{"x":11.75, "y":2},
|
||||
{"x":12.75, "y":2},
|
||||
{"x":13.75, "y":1, "w":1.25, "h":2},
|
||||
{"x":15, "y":2},
|
||||
|
||||
{"x":0, "y":3, "w":1.25},
|
||||
{"x":1.25, "y":3},
|
||||
{"x":2.25, "y":3},
|
||||
{"x":3.25, "y":3},
|
||||
{"x":4.25, "y":3},
|
||||
{"x":5.25, "y":3},
|
||||
{"x":6.25, "y":3},
|
||||
{"x":7.25, "y":3},
|
||||
{"x":8.25, "y":3},
|
||||
{"x":9.25, "y":3},
|
||||
{"x":10.25, "y":3},
|
||||
{"x":11.25, "y":3},
|
||||
{"x":12.25, "y":3, "w":1.75},
|
||||
{"x":14, "y":3},
|
||||
{"x":15, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":1.5},
|
||||
{"x":1.5, "y":4},
|
||||
{"x":2.5, "y":4, "w":1.5},
|
||||
{"x":4, "y":4, "w":7},
|
||||
{"x":11, "y":4, "w":1.5},
|
||||
{"x":13, "y":4},
|
||||
{"x":14, "y":4},
|
||||
{"x":15, "y":4}
|
||||
]
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,34 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[0] = LAYOUT_all(
|
||||
KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_BSLS, KC_GRV,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_GRV, KC_ENT, KC_PGUP,
|
||||
KC_LSFT, KC_BSLS, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, KC_PGDN,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, MO(1), KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
[1] = LAYOUT_all(
|
||||
_______, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, _______, _______, _______,
|
||||
_______, RGB_TOG, KC_UP, RGB_MOD, RGB_HUI, RGB_HUD, RGB_SAI, RGB_SAD, RGB_VAI, RGB_VAD, KC_PSCR, KC_SLCK, KC_PAUS, _______, _______,
|
||||
_______, KC_LEFT, KC_DOWN, KC_RGHT, _______, _______, _______, _______, _______, _______, KC_INS, KC_HOME, KC_PGUP, _______, _______,
|
||||
_______, _______, RESET, BL_DEC, BL_TOGG, BL_INC, KC_VOLD, KC_MUTE, KC_VOLU, _______, KC_DEL, KC_END, KC_PGDN, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
};
|
|
@ -0,0 +1,48 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[0] = LAYOUT_all(
|
||||
KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_BSLS, KC_GRV,
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL,
|
||||
KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_GRV, KC_ENT, KC_PGUP,
|
||||
KC_LSFT, KC_BSLS, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, KC_PGDN,
|
||||
KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, MO(1), KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
[1] = LAYOUT_all(
|
||||
_______, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, _______, _______, _______,
|
||||
_______, RGB_TOG, KC_UP, RGB_MOD, RGB_HUI, RGB_HUD, RGB_SAI, RGB_SAD, RGB_VAI, RGB_VAD, KC_PSCR, KC_SLCK, KC_PAUS, _______, _______,
|
||||
_______, KC_LEFT, KC_DOWN, KC_RGHT, _______, _______, _______, _______, _______, _______, KC_INS, KC_HOME, KC_PGUP, _______, _______,
|
||||
_______, _______, RESET, BL_DEC, BL_TOGG, BL_INC, KC_VOLD, KC_MUTE, KC_VOLU, _______, KC_DEL, KC_END, KC_PGDN, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
[2] = LAYOUT_all(
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
[3] = LAYOUT_all(
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
};
|
|
@ -0,0 +1,2 @@
|
|||
VIA_ENABLE = yes
|
||||
LTO_ENABLE = yes
|
|
@ -0,0 +1,21 @@
|
|||
# Palette G67 Soldered
|
||||
|
||||
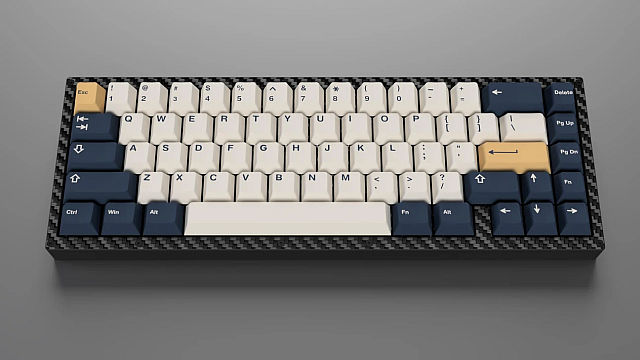
|
||||
|
||||
A blockered 65% with RGB underglow, USB Type C, and footprints for in-switch backlight.
|
||||
|
||||
* Keyboard Maintainer: [The QMK Community](https://github.com/qmk)
|
||||
* Hardware Supported: Palette G67 Soldered (ATmega32U4)
|
||||
* Hardware Availability: [NEO Keys](https://www.neokeys.net/)
|
||||
|
||||
Make example for this keyboard (after setting up your build environment):
|
||||
|
||||
make neokeys/palette_g67/soldered:default
|
||||
|
||||
Flashing example for this keyboard:
|
||||
|
||||
make neokeys/palette_g67/soldered:default:flash
|
||||
|
||||
To reset the board into bootloader mode, hold the key at the top left of the keyboard while connecting the USB cable (also erases persistent settings).
|
||||
|
||||
See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
|
|
@ -0,0 +1,24 @@
|
|||
# MCU name
|
||||
MCU = atmega32u4
|
||||
|
||||
# Bootloader selection
|
||||
BOOTLOADER = atmel-dfu
|
||||
|
||||
# Build Options
|
||||
# change yes to no to disable
|
||||
#
|
||||
BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration
|
||||
MOUSEKEY_ENABLE = yes # Mouse keys
|
||||
EXTRAKEY_ENABLE = yes # Audio control and System control
|
||||
CONSOLE_ENABLE = no # Console for debug
|
||||
COMMAND_ENABLE = no # Commands for debug and configuration
|
||||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
|
||||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
|
||||
# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
|
||||
NKRO_ENABLE = no # USB Nkey Rollover
|
||||
BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality
|
||||
RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow
|
||||
BLUETOOTH_ENABLE = no # Enable Bluetooth
|
||||
AUDIO_ENABLE = no # Audio output
|
||||
|
||||
LAYOUTS = 65_ansi 65_ansi_blocker 65_ansi_blocker_tsangan 65_iso 65_iso_blocker # 65_iso_blocker_tsangan
|
|
@ -0,0 +1,17 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include "soldered.h"
|
|
@ -0,0 +1,117 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "quantum.h"
|
||||
|
||||
#define LAYOUT_all( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1E, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, K2F, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K48, K49, K4B, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0D, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, K1E, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, KC_NO, K2F }, \
|
||||
{ K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, K48, K49, KC_NO, K4B, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
||||
|
||||
#define LAYOUT_65_ansi( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1E, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2D, K2F, \
|
||||
K30, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K48, K49, K4B, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, KC_NO, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, K1E, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, KC_NO, K2D, KC_NO, K2F }, \
|
||||
{ K30, KC_NO, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, K48, K49, KC_NO, K4B, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
||||
|
||||
#define LAYOUT_65_ansi_blocker( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1E, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2D, K2F, \
|
||||
K30, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K48, K49, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, KC_NO, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, K1E, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, KC_NO, K2D, KC_NO, K2F }, \
|
||||
{ K30, KC_NO, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, K48, K49, KC_NO, KC_NO, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
||||
|
||||
#define LAYOUT_65_ansi_blocker_tsangan( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1E, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2D, K2F, \
|
||||
K30, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K49, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, KC_NO, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, K1E, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, KC_NO, K2D, KC_NO, K2F }, \
|
||||
{ K30, KC_NO, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, KC_NO, K49, KC_NO, KC_NO, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
||||
|
||||
#define LAYOUT_65_iso( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, K2F, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K48, K49, K4B, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, KC_NO, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, KC_NO, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, KC_NO, K2F }, \
|
||||
{ K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, K48, K49, KC_NO, K4B, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
||||
|
||||
#define LAYOUT_65_iso_blocker( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, K2F, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K48, K49, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, KC_NO, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, KC_NO, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, KC_NO, K2F }, \
|
||||
{ K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, K48, K49, KC_NO, KC_NO, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
||||
|
||||
#define LAYOUT_65_iso_blocker_tsangan( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, K0E, K0F, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, K1F, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, K2F, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, K3E, K3F, \
|
||||
K40, K41, K42, K47, K49, K4C, K4D, K4F \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, K0C, KC_NO, K0E, K0F }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, K1C, KC_NO, KC_NO, K1F }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, K2C, K2D, KC_NO, K2F }, \
|
||||
{ K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B, K3C, KC_NO, K3E, K3F }, \
|
||||
{ K40, K41, K42, KC_NO, KC_NO, KC_NO, KC_NO, K47, KC_NO, K49, KC_NO, KC_NO, K4C, K4D, KC_NO, K4F }, \
|
||||
}
|
|
@ -0,0 +1,6 @@
|
|||
# polycarbdiet
|
||||
QMK folder for custom PCB firmware.
|
||||
|
||||
Github: polycarbdiet
|
||||
Discord: polycarb_diet
|
||||
Reddit: u/polycarb_diet
|
|
@ -0,0 +1,61 @@
|
|||
/* Copyright 2020 Muhammad Galib (polycarbdiet) <pd.keyboards@gmail.com>
|
||||
|
||||
This program is free software: you can redistribute it and/or modify
|
||||
it under the terms of the GNU General Public License as published by
|
||||
the Free Software Foundation, either version 2 of the License, or
|
||||
(at your option) any later version.
|
||||
|
||||
This program is distributed in the hope that it will be useful,
|
||||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
GNU General Public License for more details.
|
||||
|
||||
You should have received a copy of the GNU General Public License
|
||||
along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "config_common.h"
|
||||
|
||||
/* USB Device descriptor parameter */
|
||||
#define VENDOR_ID 0x5040 // PD = polycarbdiet
|
||||
#define PRODUCT_ID 0x7320 // S20
|
||||
#define DEVICE_VER 0x0001
|
||||
#define MANUFACTURER polycarbdiet
|
||||
#define PRODUCT S20
|
||||
|
||||
/* key matrix size */
|
||||
#define MATRIX_ROWS 5
|
||||
#define MATRIX_COLS 4
|
||||
|
||||
// 1 2 3 4 5
|
||||
#define MATRIX_ROW_PINS { B7, E6, D0, D1, D5 }
|
||||
#define MATRIX_COL_PINS { C6, C7, D4, D6 }
|
||||
#define UNUSED_PINS
|
||||
|
||||
/* COL2ROW, ROW2COL */
|
||||
#define DIODE_DIRECTION ROW2COL
|
||||
|
||||
#define BACKLIGHT_PIN B6
|
||||
#define BACKLIGHT_LEVELS 3
|
||||
#define BACKLIGHT_BREATHING
|
||||
|
||||
#define RGB_DI_PIN B3
|
||||
#define RGBLED_NUM 4
|
||||
#define RGBLIGHT_HUE_STEP 8
|
||||
#define RGBLIGHT_SAT_STEP 8
|
||||
#define RGBLIGHT_VAL_STEP 8
|
||||
#define RGBLIGHT_LIMIT_VAL 255 /* The maximum brightness level */
|
||||
#define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */
|
||||
|
||||
#define RGBLIGHT_ANIMATIONS
|
||||
|
||||
#define DEBOUNCE 5
|
||||
|
||||
/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */
|
||||
#define LOCKING_SUPPORT_ENABLE
|
||||
/* Locking resynchronize hack */
|
||||
#define LOCKING_RESYNC_ENABLE
|
||||
|
||||
#define FORCE_NKRO
|
|
@ -0,0 +1,88 @@
|
|||
{
|
||||
"keyboard_name": "S20 revA",
|
||||
"url": "",
|
||||
"maintainer": "polycarbdiet",
|
||||
"width": 4,
|
||||
"height": 5,
|
||||
"layouts": {
|
||||
"LAYOUT_ortho_5x4": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
|
||||
{"x":0, "y":1},
|
||||
{"x":1, "y":1},
|
||||
{"x":2, "y":1},
|
||||
{"x":3, "y":1},
|
||||
|
||||
{"x":0, "y":2},
|
||||
{"x":1, "y":2},
|
||||
{"x":2, "y":2},
|
||||
{"x":3, "y":2},
|
||||
|
||||
{"x":0, "y":3},
|
||||
{"x":1, "y":3},
|
||||
{"x":2, "y":3},
|
||||
{"x":3, "y":3},
|
||||
|
||||
{"x":0, "y":4},
|
||||
{"x":1, "y":4},
|
||||
{"x":2, "y":4},
|
||||
{"x":3, "y":4}
|
||||
]
|
||||
},
|
||||
"LAYOUT_numpad_5x4":{
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
|
||||
{"x":0, "y":1},
|
||||
{"x":1, "y":1},
|
||||
{"x":2, "y":1},
|
||||
|
||||
{"x":0, "y":2},
|
||||
{"x":1, "y":2},
|
||||
{"x":2, "y":2},
|
||||
{"x":3, "y":1, "h":2},
|
||||
{"x":0, "y":3},
|
||||
{"x":1, "y":3},
|
||||
{"x":2, "y":3},
|
||||
|
||||
{"x":0, "y":4, "w":2},
|
||||
{"x":2, "y":4},
|
||||
{"x":3, "y":3, "h":2}
|
||||
]
|
||||
},
|
||||
"LAYOUT_enter": {
|
||||
"layout": [
|
||||
{"x":0, "y":0},
|
||||
{"x":1, "y":0},
|
||||
{"x":2, "y":0},
|
||||
{"x":3, "y":0},
|
||||
|
||||
{"x":0, "y":1},
|
||||
{"x":1, "y":1},
|
||||
{"x":2, "y":1},
|
||||
{"x":3, "y":1},
|
||||
|
||||
{"x":0, "y":2},
|
||||
{"x":1, "y":2},
|
||||
{"x":2, "y":2},
|
||||
{"x":3, "y":2},
|
||||
|
||||
{"x":0, "y":3},
|
||||
{"x":1, "y":3},
|
||||
{"x":2, "y":3},
|
||||
{"x":3, "y":3, "h":2},
|
||||
|
||||
{"x":0, "y":4},
|
||||
{"x":1, "y":4},
|
||||
{"x":2, "y":4}
|
||||
]
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,47 @@
|
|||
/* Copyright 2020 Muhammad Galib (polycarbdiet) <pd.keyboards@gmail.com>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[0] = LAYOUT_ortho_5x4(
|
||||
KC_ESC, KC_PAST, KC_PSLS, KC_BSPC,
|
||||
KC_P7, KC_P8, KC_P9, KC_PMNS,
|
||||
KC_P4, KC_P5, KC_P6, KC_PPLS,
|
||||
KC_P1, KC_P2, KC_P3, KC_PENT,
|
||||
KC_P0, KC_PDOT, MO(1), RGB_TOG
|
||||
),
|
||||
[1] = LAYOUT_ortho_5x4(
|
||||
KC_NLCK, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_MPRV, KC_MPLY, KC_MNXT, KC_TRNS,
|
||||
KC_VOLD, KC_MUTE, KC_VOLU, KC_TRNS,
|
||||
RESET, MO(2), KC_TRNS, KC_TRNS
|
||||
),
|
||||
[2] = LAYOUT_ortho_5x4(
|
||||
RGB_TOG, RGB_HUI, RGB_SAI, RGB_VAI,
|
||||
RGB_MOD, RGB_HUD, RGB_SAD, RGB_VAD,
|
||||
BL_TOGG, BL_ON, BL_INC, BL_STEP,
|
||||
KC_TRNS, BL_OFF, BL_DEC, BL_BRTG,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS
|
||||
),
|
||||
[3] = LAYOUT_ortho_5x4(
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS
|
||||
)
|
||||
};
|
|
@ -0,0 +1 @@
|
|||
# The default keymap for S20
|
|
@ -0,0 +1,27 @@
|
|||
# S20
|
||||
|
||||

|
||||
|
||||
The S20 keyboard is a FR4 sandwich keyboard which was orginally designed to add extra functionality to a user's current keyboard setup.
|
||||
|
||||
* Keyboard Maintainer: [polycarbdiet](https://github.com/polycarbdiet)
|
||||
* Hardware Supported: S20 - FR4 sandwich MacroPad/NumberPad
|
||||
* Hardware Availability: Custom, limitied quantaties.
|
||||
|
||||
Make example for this keyboard (after setting up your build environment):
|
||||
|
||||
make polycarbdiet/s20:default
|
||||
|
||||
Flashing example for this keyboard:
|
||||
|
||||
make polycarbdiet/s20:default:flash
|
||||
|
||||
**Reset Method:**
|
||||
- Press the `RESET` button on the under side of the PCB
|
||||
|
||||
**Bootloader Method:**
|
||||
- Hold down the key located at `K00`, commonly programmed as `ESC`, while plugging in the keyboard.
|
||||
- Hold down the key located at `K00`, commonly programmed as `ESC`, and press the RESET button on the PCB.
|
||||
- Hold down the key located at `K42` and then press the key located at `K40`.
|
||||
|
||||
See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
|
|
@ -0,0 +1,23 @@
|
|||
# MCU name
|
||||
MCU = atmega32u4
|
||||
|
||||
# Bootloader selection
|
||||
BOOTLOADER = atmel-dfu
|
||||
|
||||
# Build Options
|
||||
|
||||
BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration
|
||||
MOUSEKEY_ENABLE = yes # Mouse keys
|
||||
EXTRAKEY_ENABLE = yes # Audio control and System control
|
||||
CONSOLE_ENABLE = no # Console for debug
|
||||
COMMAND_ENABLE = no # Commands for debug and configuration
|
||||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
|
||||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
|
||||
# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
|
||||
NKRO_ENABLE = yes # USB Nkey Rollover
|
||||
BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality
|
||||
RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow
|
||||
BLUETOOTH_ENABLE = no # Enable Bluetooth
|
||||
AUDIO_ENABLE = no # Audio output
|
||||
|
||||
LAYOUTS = ortho_5x4 numpad_5x4
|
|
@ -0,0 +1,17 @@
|
|||
/* Copyright 2020 Muhammad Galib (polycarbdiet) <pd.keyboards@gmail.com>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include "s20.h"
|
|
@ -0,0 +1,61 @@
|
|||
/* Copyright 2020 Muhammad Galib (polycarbdiet) <pd.keyboards@gmail.com>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "quantum.h"
|
||||
|
||||
#define LAYOUT_ortho_5x4( \
|
||||
K00, K01, K02, K03, \
|
||||
K10, K11, K12, K13, \
|
||||
K20, K21, K22, K23, \
|
||||
K30, K31, K32, K33, \
|
||||
K40, K41, K42, K43 \
|
||||
) { \
|
||||
{ K00, K01, K02, K03 }, \
|
||||
{ K10, K11, K12, K13 }, \
|
||||
{ K20, K21, K22, K23 }, \
|
||||
{ K30, K31, K32, K33 }, \
|
||||
{ K40, K41, K42, K43 } \
|
||||
}
|
||||
|
||||
#define LAYOUT_numpad_5x4( \
|
||||
K00, K01, K02, K03, \
|
||||
K10, K11, K12, \
|
||||
K20, K21, K22, K13, \
|
||||
K30, K31, K32, \
|
||||
K40, K42, K33 \
|
||||
) { \
|
||||
{ K00, K01, K02, K03 }, \
|
||||
{ K10, K11, K12, K13 }, \
|
||||
{ K20, K21, K22, KC_NO }, \
|
||||
{ K30, K31, K32, K33 }, \
|
||||
{ K40, KC_NO, K42, KC_NO } \
|
||||
}
|
||||
|
||||
#define LAYOUT_enter( \
|
||||
K00, K01, K02, K03, \
|
||||
K10, K11, K12, K13, \
|
||||
K20, K21, K22, K23, \
|
||||
K30, K31, K32, K33, \
|
||||
K40, K41, K42 \
|
||||
) { \
|
||||
{ K00, K01, K02, K03 }, \
|
||||
{ K10, K11, K12, K13 }, \
|
||||
{ K20, K21, K22, K23 }, \
|
||||
{ K30, K31, K32, K33 }, \
|
||||
{ K40, K41, K42, KC_NO } \
|
||||
}
|
|
@ -0,0 +1,71 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "config_common.h"
|
||||
|
||||
/* USB Device descriptor parameter */
|
||||
#define VENDOR_ID 0x594D // "YM"
|
||||
#define PRODUCT_ID 0x4440 // "D" + 40
|
||||
#define DEVICE_VER 0x0001
|
||||
#define MANUFACTURER YMDK
|
||||
#define PRODUCT YMD40 v2
|
||||
|
||||
/* key matrix size */
|
||||
#define MATRIX_ROWS 4
|
||||
#define MATRIX_COLS 12
|
||||
|
||||
/*
|
||||
* Keyboard Matrix Assignments
|
||||
*
|
||||
* Change this to how you wired your keyboard
|
||||
* COLS: AVR pins used for columns, left to right
|
||||
* ROWS: AVR pins used for rows, top to bottom
|
||||
* DIODE_DIRECTION: COL2ROW = COL = Anode (+), ROW = Cathode (-, marked on diode)
|
||||
* ROW2COL = ROW = Anode (+), COL = Cathode (-, marked on diode)
|
||||
*
|
||||
*/
|
||||
#define MATRIX_ROW_PINS { D0, B3, B2, B1 }
|
||||
#define MATRIX_COL_PINS { F1, F0, B0, C7, F4, F5, F6, F7, D4, D6, B4, D7 }
|
||||
|
||||
#define DIODE_DIRECTION COL2ROW
|
||||
|
||||
#define BACKLIGHT_PIN B6
|
||||
#define BACKLIGHT_BREATHING
|
||||
#define BACKLIGHT_LEVELS 3
|
||||
|
||||
#if defined(RGBLIGHT_ENABLE)
|
||||
#define RGB_DI_PIN E2
|
||||
#define RGBLED_NUM 8
|
||||
#define RGBLIGHT_HUE_STEP 8
|
||||
#define RGBLIGHT_SAT_STEP 8
|
||||
#define RGBLIGHT_VAL_STEP 8
|
||||
#define RGBLIGHT_LIMIT_VAL 150 /* The maximum brightness level */
|
||||
#define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */
|
||||
/*== all animations enable ==*/
|
||||
#define RGBLIGHT_ANIMATIONS
|
||||
// /*== or choose animations ==*/
|
||||
// #define RGBLIGHT_EFFECT_BREATHING
|
||||
// #define RGBLIGHT_EFFECT_RAINBOW_MOOD
|
||||
// #define RGBLIGHT_EFFECT_RAINBOW_SWIRL
|
||||
// #define RGBLIGHT_EFFECT_SNAKE
|
||||
// #define RGBLIGHT_EFFECT_KNIGHT
|
||||
// #define RGBLIGHT_EFFECT_CHRISTMAS
|
||||
// #define RGBLIGHT_EFFECT_STATIC_GRADIENT
|
||||
// #define RGBLIGHT_EFFECT_RGB_TEST
|
||||
// #define RGBLIGHT_EFFECT_ALTERNATING
|
||||
#endif
|
|
@ -0,0 +1,64 @@
|
|||
{
|
||||
"keyboard_name": "ymd40v2",
|
||||
"url": "",
|
||||
"maintainer": "qmk",
|
||||
"width": 12,
|
||||
"height": 4,
|
||||
"layouts": {
|
||||
"LAYOUT_ortho_4x12": {
|
||||
"layout": [
|
||||
{"label":"K00 (D0,F1)", "x":0, "y":0},
|
||||
{"label":"K01 (D0,F0)", "x":1, "y":0},
|
||||
{"label":"K02 (D0,B0)", "x":2, "y":0},
|
||||
{"label":"K03 (D0,C7)", "x":3, "y":0},
|
||||
{"label":"K04 (D0,F4)", "x":4, "y":0},
|
||||
{"label":"K05 (D0,F5)", "x":5, "y":0},
|
||||
{"label":"K06 (D0,F6)", "x":6, "y":0},
|
||||
{"label":"K07 (D0,F7)", "x":7, "y":0},
|
||||
{"label":"K08 (D0,D4)", "x":8, "y":0},
|
||||
{"label":"K09 (D0,D6)", "x":9, "y":0},
|
||||
{"label":"K0A (D0,B4)", "x":10, "y":0},
|
||||
{"label":"K0B (D0,D7)", "x":11, "y":0},
|
||||
|
||||
{"label":"K10 (B3,F1)", "x":0, "y":1},
|
||||
{"label":"K11 (B3,F0)", "x":1, "y":1},
|
||||
{"label":"K12 (B3,B0)", "x":2, "y":1},
|
||||
{"label":"K13 (B3,C7)", "x":3, "y":1},
|
||||
{"label":"K14 (B3,F4)", "x":4, "y":1},
|
||||
{"label":"K15 (B3,F5)", "x":5, "y":1},
|
||||
{"label":"K16 (B3,F6)", "x":6, "y":1},
|
||||
{"label":"K17 (B3,F7)", "x":7, "y":1},
|
||||
{"label":"K18 (B3,D4)", "x":8, "y":1},
|
||||
{"label":"K19 (B3,D6)", "x":9, "y":1},
|
||||
{"label":"K1A (B3,B4)", "x":10, "y":1},
|
||||
{"label":"K1B (B3,D7)", "x":11, "y":1},
|
||||
|
||||
{"label":"K20 (B2,F1)", "x":0, "y":2},
|
||||
{"label":"K21 (B2,F0)", "x":1, "y":2},
|
||||
{"label":"K22 (B2,B0)", "x":2, "y":2},
|
||||
{"label":"K23 (B2,C7)", "x":3, "y":2},
|
||||
{"label":"K24 (B2,F4)", "x":4, "y":2},
|
||||
{"label":"K25 (B2,F5)", "x":5, "y":2},
|
||||
{"label":"K26 (B2,F6)", "x":6, "y":2},
|
||||
{"label":"K27 (B2,F7)", "x":7, "y":2},
|
||||
{"label":"K28 (B2,D4)", "x":8, "y":2},
|
||||
{"label":"K29 (B2,D6)", "x":9, "y":2},
|
||||
{"label":"K2A (B2,B4)", "x":10, "y":2},
|
||||
{"label":"K2B (B2,D7)", "x":11, "y":2},
|
||||
|
||||
{"label":"K30 (B1,F1)", "x":0, "y":3},
|
||||
{"label":"K31 (B1,F0)", "x":1, "y":3},
|
||||
{"label":"K32 (B1,B0)", "x":2, "y":3},
|
||||
{"label":"K33 (B1,C7)", "x":3, "y":3},
|
||||
{"label":"K34 (B1,F4)", "x":4, "y":3},
|
||||
{"label":"K35 (B1,F5)", "x":5, "y":3},
|
||||
{"label":"K36 (B1,F6)", "x":6, "y":3},
|
||||
{"label":"K37 (B1,F7)", "x":7, "y":3},
|
||||
{"label":"K38 (B1,D4)", "x":8, "y":3},
|
||||
{"label":"K39 (B1,D6)", "x":9, "y":3},
|
||||
{"label":"K3A (B1,B4)", "x":10, "y":3},
|
||||
{"label":"K3B (B1,D7)", "x":11, "y":3}
|
||||
]
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,55 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
enum layer_names {
|
||||
_QWERTY,
|
||||
_LOWER,
|
||||
_RAISE,
|
||||
_ADJUST
|
||||
};
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[_QWERTY] = LAYOUT_ortho_4x12(
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_BSPC,
|
||||
KC_ESC, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_ENT,
|
||||
KC_CAPS, KC_LCTL, KC_LALT, KC_LGUI, MO(1), KC_SPC, KC_SPC, MO(2), KC_UP, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
[_LOWER] = LAYOUT_ortho_4x12(
|
||||
KC_TILD, KC_EXLM, KC_AT, KC_HASH, KC_DLR, KC_PERC, KC_CIRC, KC_AMPR, KC_ASTR, KC_LPRN, KC_RPRN, KC_BSPC,
|
||||
KC_DEL, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_UNDS, KC_PLUS, KC_LCBR, KC_RCBR, KC_PIPE,
|
||||
_______, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, S(KC_NUHS), S(KC_NUBS), KC_HOME, KC_END, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, KC_MNXT, KC_VOLD, KC_VOLU, KC_MPLY
|
||||
),
|
||||
[_RAISE] = LAYOUT_ortho_4x12(
|
||||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_BSPC,
|
||||
KC_DEL, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_MINS, KC_EQL, KC_LBRC, KC_RBRC, KC_BSLS,
|
||||
_______, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_NUHS, KC_NUBS, KC_PGUP, KC_PGDN, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, KC_MNXT, KC_VOLD, KC_VOLU, KC_MPLY
|
||||
),
|
||||
[_ADJUST] = LAYOUT_ortho_4x12(
|
||||
RESET, RGB_TOG, RGB_HUI, RGB_SAI, RGB_VAI, _______, _______, _______, _______, _______, _______, DEBUG,
|
||||
_______, RGB_MOD, RGB_HUD, RGB_SAD, RGB_VAD, AG_NORM, AG_SWAP, _______, _______, _______, _______, _______,
|
||||
_______, BL_TOGG, BL_DEC, BL_INC, BL_BRTG, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
)
|
||||
};
|
||||
|
||||
layer_state_t layer_state_set_user(layer_state_t state) {
|
||||
return update_tri_layer_state(state, _LOWER, _RAISE, _ADJUST);
|
||||
}
|
|
@ -0,0 +1 @@
|
|||
# The default keymap for YMD40 v2
|
|
@ -0,0 +1,32 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
[0] = LAYOUT_ortho_4x12(
|
||||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_BSPC,
|
||||
KC_ESC, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_ENT,
|
||||
KC_CAPS, KC_LCTL, KC_LALT, KC_LGUI, KC_DEL, KC_SPC, KC_SPC, RGB_MOD, KC_UP, KC_LEFT, KC_DOWN, KC_RGHT
|
||||
),
|
||||
[1] = LAYOUT_ortho_4x12(
|
||||
RESET, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
|
||||
_______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
|
||||
),
|
||||
};
|
|
@ -0,0 +1,3 @@
|
|||
# The factory keymap for YMD40 v2
|
||||
|
||||
This is the keymap assigned by the KBFirmware-format JSON file distributed by the vendor. This is included mainly for reference; if you wish to create your own keymap, the `default` keymap is a better starting point.
|
|
@ -0,0 +1,22 @@
|
|||
# YMD40 v2
|
||||
|
||||
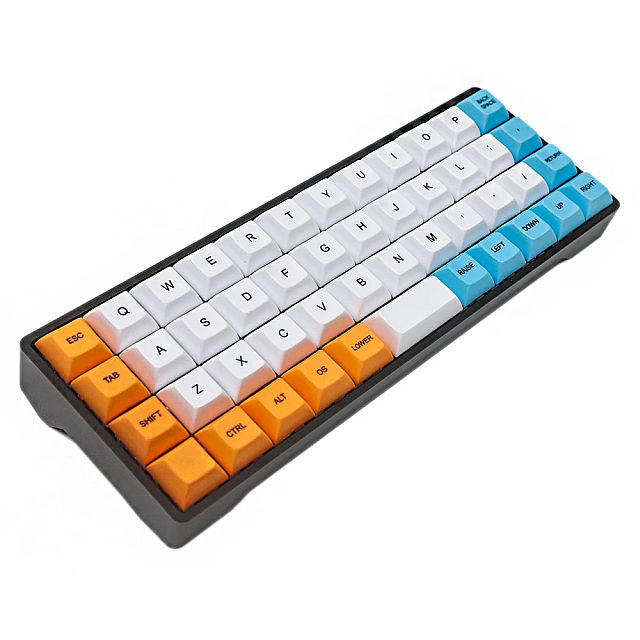\
|
||||
[PCB photo](https://raw.githubusercontent.com/noroadsleft/qmk_images/master/keyboards/ymdk/ymd40/v2/ymdk_ymd40_v2_pcb.jpg)
|
||||
|
||||
A 40% ortholinear keyboard with in-switch LED support, underglow, and USB Type C.
|
||||
|
||||
* Keyboard Maintainer: [The QMK Community](https://github.com/qmk)
|
||||
* Hardware Supported: YMD40v2 PCB (ATmega32U4)
|
||||
* Hardware Availability: [AliExpress](https://www.aliexpress.com/i/32821953148.html), [ymdkey.com](https://ymdkey.com/collections/40-mini-diy/products/ymd40-v2-diy-kit-qmk-type-c-pcb-cnc-case-plate-for-40-mini-cute-mechanical-keyboard-similar-to-planck-layout)
|
||||
|
||||
Make example for this keyboard (after setting up your build environment):
|
||||
|
||||
make ymdk/ymd40/v2:default
|
||||
|
||||
Flashing example for this keyboard:
|
||||
|
||||
make ymdk/ymd40/v2:default:flash
|
||||
|
||||
To reset the board into bootloader mode, hold the key at the top left of the keyboard while connecting the USB cable (also erases persistent settings).
|
||||
|
||||
See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
|
|
@ -0,0 +1,28 @@
|
|||
# MCU name
|
||||
MCU = atmega32u4
|
||||
|
||||
# Bootloader selection
|
||||
BOOTLOADER = atmel-dfu
|
||||
|
||||
# Build Options
|
||||
# change yes to no to disable
|
||||
#
|
||||
BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration
|
||||
MOUSEKEY_ENABLE = yes # Mouse keys
|
||||
EXTRAKEY_ENABLE = yes # Audio control and System control
|
||||
CONSOLE_ENABLE = no # Console for debug
|
||||
COMMAND_ENABLE = no # Commands for debug and configuration
|
||||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
|
||||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
|
||||
# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
|
||||
NKRO_ENABLE = no # USB Nkey Rollover
|
||||
BACKLIGHT_ENABLE = yes # Enable keyboard backlight functionality
|
||||
RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow
|
||||
BLUETOOTH_ENABLE = no # Enable Bluetooth
|
||||
AUDIO_ENABLE = no # Audio output
|
||||
|
||||
KEY_LOCK_ENABLE = no # Enable KC_LOCK support
|
||||
|
||||
AUDIO_SUPPORTED = no
|
||||
|
||||
LAYOUTS = ortho_4x12
|
|
@ -0,0 +1,17 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include "v2.h"
|
|
@ -0,0 +1,31 @@
|
|||
/* Copyright 2021 James Young (@noroadsleft)
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include "quantum.h"
|
||||
|
||||
#define LAYOUT_ortho_4x12( \
|
||||
K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B, \
|
||||
K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B, \
|
||||
K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B, \
|
||||
K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B \
|
||||
) { \
|
||||
{ K00, K01, K02, K03, K04, K05, K06, K07, K08, K09, K0A, K0B }, \
|
||||
{ K10, K11, K12, K13, K14, K15, K16, K17, K18, K19, K1A, K1B }, \
|
||||
{ K20, K21, K22, K23, K24, K25, K26, K27, K28, K29, K2A, K2B }, \
|
||||
{ K30, K31, K32, K33, K34, K35, K36, K37, K38, K39, K3A, K3B }, \
|
||||
}
|
|
@ -16,357 +16,14 @@
|
|||
|
||||
#pragma once
|
||||
|
||||
#ifndef __ASSEMBLER__
|
||||
# include "pin_defs.h"
|
||||
#endif
|
||||
|
||||
/* diode directions */
|
||||
#define COL2ROW 0
|
||||
#define ROW2COL 1
|
||||
|
||||
// useful for direct pin mapping
|
||||
#define NO_PIN (pin_t)(~0)
|
||||
|
||||
#ifdef __AVR__
|
||||
# ifndef __ASSEMBLER__
|
||||
# include <avr/io.h>
|
||||
# endif
|
||||
# define PORT_SHIFTER 4 // this may be 4 for all AVR chips
|
||||
|
||||
// If you want to add more to this list, reference the PINx definitions in these header
|
||||
// files: https://github.com/vancegroup-mirrors/avr-libc/tree/master/avr-libc/include/avr
|
||||
|
||||
# if defined(__AVR_ATmega32U4__) || defined(__AVR_ATmega16U4__)
|
||||
# define ADDRESS_BASE 0x00
|
||||
# define PINB_ADDRESS 0x3
|
||||
# define PINC_ADDRESS 0x6
|
||||
# define PIND_ADDRESS 0x9
|
||||
# define PINE_ADDRESS 0xC
|
||||
# define PINF_ADDRESS 0xF
|
||||
# elif defined(__AVR_AT90USB162__) || defined(__AVR_ATmega16U2__) || defined(__AVR_ATmega32U2__) || defined(__AVR_ATmega328P__) || defined(__AVR_ATmega328__)
|
||||
# define ADDRESS_BASE 0x00
|
||||
# define PINB_ADDRESS 0x3
|
||||
# define PINC_ADDRESS 0x6
|
||||
# define PIND_ADDRESS 0x9
|
||||
# elif defined(__AVR_AT90USB646__) || defined(__AVR_AT90USB647__) || defined(__AVR_AT90USB1286__) || defined(__AVR_AT90USB1287__)
|
||||
# define ADDRESS_BASE 0x00
|
||||
# define PINA_ADDRESS 0x0
|
||||
# define PINB_ADDRESS 0x3
|
||||
# define PINC_ADDRESS 0x6
|
||||
# define PIND_ADDRESS 0x9
|
||||
# define PINE_ADDRESS 0xC
|
||||
# define PINF_ADDRESS 0xF
|
||||
# elif defined(__AVR_ATmega32A__)
|
||||
# define ADDRESS_BASE 0x10
|
||||
# define PIND_ADDRESS 0x0
|
||||
# define PINC_ADDRESS 0x3
|
||||
# define PINB_ADDRESS 0x6
|
||||
# define PINA_ADDRESS 0x9
|
||||
# elif defined(__AVR_ATtiny85__)
|
||||
# define ADDRESS_BASE 0x10
|
||||
# define PINB_ADDRESS 0x6
|
||||
# else
|
||||
# error "Pins are not defined"
|
||||
# endif
|
||||
|
||||
/* I/O pins */
|
||||
# define PINDEF(port, pin) ((PIN##port##_ADDRESS << PORT_SHIFTER) | pin)
|
||||
|
||||
# ifdef PORTA
|
||||
# define A0 PINDEF(A, 0)
|
||||
# define A1 PINDEF(A, 1)
|
||||
# define A2 PINDEF(A, 2)
|
||||
# define A3 PINDEF(A, 3)
|
||||
# define A4 PINDEF(A, 4)
|
||||
# define A5 PINDEF(A, 5)
|
||||
# define A6 PINDEF(A, 6)
|
||||
# define A7 PINDEF(A, 7)
|
||||
# endif
|
||||
# ifdef PORTB
|
||||
# define B0 PINDEF(B, 0)
|
||||
# define B1 PINDEF(B, 1)
|
||||
# define B2 PINDEF(B, 2)
|
||||
# define B3 PINDEF(B, 3)
|
||||
# define B4 PINDEF(B, 4)
|
||||
# define B5 PINDEF(B, 5)
|
||||
# define B6 PINDEF(B, 6)
|
||||
# define B7 PINDEF(B, 7)
|
||||
# endif
|
||||
# ifdef PORTC
|
||||
# define C0 PINDEF(C, 0)
|
||||
# define C1 PINDEF(C, 1)
|
||||
# define C2 PINDEF(C, 2)
|
||||
# define C3 PINDEF(C, 3)
|
||||
# define C4 PINDEF(C, 4)
|
||||
# define C5 PINDEF(C, 5)
|
||||
# define C6 PINDEF(C, 6)
|
||||
# define C7 PINDEF(C, 7)
|
||||
# endif
|
||||
# ifdef PORTD
|
||||
# define D0 PINDEF(D, 0)
|
||||
# define D1 PINDEF(D, 1)
|
||||
# define D2 PINDEF(D, 2)
|
||||
# define D3 PINDEF(D, 3)
|
||||
# define D4 PINDEF(D, 4)
|
||||
# define D5 PINDEF(D, 5)
|
||||
# define D6 PINDEF(D, 6)
|
||||
# define D7 PINDEF(D, 7)
|
||||
# endif
|
||||
# ifdef PORTE
|
||||
# define E0 PINDEF(E, 0)
|
||||
# define E1 PINDEF(E, 1)
|
||||
# define E2 PINDEF(E, 2)
|
||||
# define E3 PINDEF(E, 3)
|
||||
# define E4 PINDEF(E, 4)
|
||||
# define E5 PINDEF(E, 5)
|
||||
# define E6 PINDEF(E, 6)
|
||||
# define E7 PINDEF(E, 7)
|
||||
# endif
|
||||
# ifdef PORTF
|
||||
# define F0 PINDEF(F, 0)
|
||||
# define F1 PINDEF(F, 1)
|
||||
# define F2 PINDEF(F, 2)
|
||||
# define F3 PINDEF(F, 3)
|
||||
# define F4 PINDEF(F, 4)
|
||||
# define F5 PINDEF(F, 5)
|
||||
# define F6 PINDEF(F, 6)
|
||||
# define F7 PINDEF(F, 7)
|
||||
# endif
|
||||
|
||||
# ifndef __ASSEMBLER__
|
||||
# define _PIN_ADDRESS(p, offset) _SFR_IO8(ADDRESS_BASE + ((p) >> PORT_SHIFTER) + (offset))
|
||||
// Port X Input Pins Address
|
||||
# define PINx_ADDRESS(p) _PIN_ADDRESS(p, 0)
|
||||
// Port X Data Direction Register, 0:input 1:output
|
||||
# define DDRx_ADDRESS(p) _PIN_ADDRESS(p, 1)
|
||||
// Port X Data Register
|
||||
# define PORTx_ADDRESS(p) _PIN_ADDRESS(p, 2)
|
||||
# endif
|
||||
|
||||
#elif defined(PROTOCOL_CHIBIOS)
|
||||
// Defines mapping for Proton C replacement
|
||||
# ifdef CONVERT_TO_PROTON_C
|
||||
// Left side (front)
|
||||
# define D3 PAL_LINE(GPIOA, 9)
|
||||
# define D2 PAL_LINE(GPIOA, 10)
|
||||
// GND
|
||||
// GND
|
||||
# define D1 PAL_LINE(GPIOB, 7)
|
||||
# define D0 PAL_LINE(GPIOB, 6)
|
||||
# define D4 PAL_LINE(GPIOB, 5)
|
||||
# define C6 PAL_LINE(GPIOB, 4)
|
||||
# define D7 PAL_LINE(GPIOB, 3)
|
||||
# define E6 PAL_LINE(GPIOB, 2)
|
||||
# define B4 PAL_LINE(GPIOB, 1)
|
||||
# define B5 PAL_LINE(GPIOB, 0)
|
||||
|
||||
// Right side (front)
|
||||
// RAW
|
||||
// GND
|
||||
// RESET
|
||||
// VCC
|
||||
# define F4 PAL_LINE(GPIOA, 2)
|
||||
# define F5 PAL_LINE(GPIOA, 1)
|
||||
# define F6 PAL_LINE(GPIOA, 0)
|
||||
# define F7 PAL_LINE(GPIOB, 8)
|
||||
# define B1 PAL_LINE(GPIOB, 13)
|
||||
# define B3 PAL_LINE(GPIOB, 14)
|
||||
# define B2 PAL_LINE(GPIOB, 15)
|
||||
# define B6 PAL_LINE(GPIOB, 9)
|
||||
|
||||
// LEDs (only D5/C13 uses an actual LED)
|
||||
# ifdef CONVERT_TO_PROTON_C_RXLED
|
||||
# define D5 PAL_LINE(GPIOC, 14)
|
||||
# define B0 PAL_LINE(GPIOC, 13)
|
||||
# else
|
||||
# define D5 PAL_LINE(GPIOC, 13)
|
||||
# define B0 PAL_LINE(GPIOC, 14)
|
||||
# endif
|
||||
# else
|
||||
# define A0 PAL_LINE(GPIOA, 0)
|
||||
# define A1 PAL_LINE(GPIOA, 1)
|
||||
# define A2 PAL_LINE(GPIOA, 2)
|
||||
# define A3 PAL_LINE(GPIOA, 3)
|
||||
# define A4 PAL_LINE(GPIOA, 4)
|
||||
# define A5 PAL_LINE(GPIOA, 5)
|
||||
# define A6 PAL_LINE(GPIOA, 6)
|
||||
# define A7 PAL_LINE(GPIOA, 7)
|
||||
# define A8 PAL_LINE(GPIOA, 8)
|
||||
# define A9 PAL_LINE(GPIOA, 9)
|
||||
# define A10 PAL_LINE(GPIOA, 10)
|
||||
# define A11 PAL_LINE(GPIOA, 11)
|
||||
# define A12 PAL_LINE(GPIOA, 12)
|
||||
# define A13 PAL_LINE(GPIOA, 13)
|
||||
# define A14 PAL_LINE(GPIOA, 14)
|
||||
# define A15 PAL_LINE(GPIOA, 15)
|
||||
# define B0 PAL_LINE(GPIOB, 0)
|
||||
# define B1 PAL_LINE(GPIOB, 1)
|
||||
# define B2 PAL_LINE(GPIOB, 2)
|
||||
# define B3 PAL_LINE(GPIOB, 3)
|
||||
# define B4 PAL_LINE(GPIOB, 4)
|
||||
# define B5 PAL_LINE(GPIOB, 5)
|
||||
# define B6 PAL_LINE(GPIOB, 6)
|
||||
# define B7 PAL_LINE(GPIOB, 7)
|
||||
# define B8 PAL_LINE(GPIOB, 8)
|
||||
# define B9 PAL_LINE(GPIOB, 9)
|
||||
# define B10 PAL_LINE(GPIOB, 10)
|
||||
# define B11 PAL_LINE(GPIOB, 11)
|
||||
# define B12 PAL_LINE(GPIOB, 12)
|
||||
# define B13 PAL_LINE(GPIOB, 13)
|
||||
# define B14 PAL_LINE(GPIOB, 14)
|
||||
# define B15 PAL_LINE(GPIOB, 15)
|
||||
# define B16 PAL_LINE(GPIOB, 16)
|
||||
# define B17 PAL_LINE(GPIOB, 17)
|
||||
# define B18 PAL_LINE(GPIOB, 18)
|
||||
# define B19 PAL_LINE(GPIOB, 19)
|
||||
# define C0 PAL_LINE(GPIOC, 0)
|
||||
# define C1 PAL_LINE(GPIOC, 1)
|
||||
# define C2 PAL_LINE(GPIOC, 2)
|
||||
# define C3 PAL_LINE(GPIOC, 3)
|
||||
# define C4 PAL_LINE(GPIOC, 4)
|
||||
# define C5 PAL_LINE(GPIOC, 5)
|
||||
# define C6 PAL_LINE(GPIOC, 6)
|
||||
# define C7 PAL_LINE(GPIOC, 7)
|
||||
# define C8 PAL_LINE(GPIOC, 8)
|
||||
# define C9 PAL_LINE(GPIOC, 9)
|
||||
# define C10 PAL_LINE(GPIOC, 10)
|
||||
# define C11 PAL_LINE(GPIOC, 11)
|
||||
# define C12 PAL_LINE(GPIOC, 12)
|
||||
# define C13 PAL_LINE(GPIOC, 13)
|
||||
# define C14 PAL_LINE(GPIOC, 14)
|
||||
# define C15 PAL_LINE(GPIOC, 15)
|
||||
# define D0 PAL_LINE(GPIOD, 0)
|
||||
# define D1 PAL_LINE(GPIOD, 1)
|
||||
# define D2 PAL_LINE(GPIOD, 2)
|
||||
# define D3 PAL_LINE(GPIOD, 3)
|
||||
# define D4 PAL_LINE(GPIOD, 4)
|
||||
# define D5 PAL_LINE(GPIOD, 5)
|
||||
# define D6 PAL_LINE(GPIOD, 6)
|
||||
# define D7 PAL_LINE(GPIOD, 7)
|
||||
# define D8 PAL_LINE(GPIOD, 8)
|
||||
# define D9 PAL_LINE(GPIOD, 9)
|
||||
# define D10 PAL_LINE(GPIOD, 10)
|
||||
# define D11 PAL_LINE(GPIOD, 11)
|
||||
# define D12 PAL_LINE(GPIOD, 12)
|
||||
# define D13 PAL_LINE(GPIOD, 13)
|
||||
# define D14 PAL_LINE(GPIOD, 14)
|
||||
# define D15 PAL_LINE(GPIOD, 15)
|
||||
# define E0 PAL_LINE(GPIOE, 0)
|
||||
# define E1 PAL_LINE(GPIOE, 1)
|
||||
# define E2 PAL_LINE(GPIOE, 2)
|
||||
# define E3 PAL_LINE(GPIOE, 3)
|
||||
# define E4 PAL_LINE(GPIOE, 4)
|
||||
# define E5 PAL_LINE(GPIOE, 5)
|
||||
# define E6 PAL_LINE(GPIOE, 6)
|
||||
# define E7 PAL_LINE(GPIOE, 7)
|
||||
# define E8 PAL_LINE(GPIOE, 8)
|
||||
# define E9 PAL_LINE(GPIOE, 9)
|
||||
# define E10 PAL_LINE(GPIOE, 10)
|
||||
# define E11 PAL_LINE(GPIOE, 11)
|
||||
# define E12 PAL_LINE(GPIOE, 12)
|
||||
# define E13 PAL_LINE(GPIOE, 13)
|
||||
# define E14 PAL_LINE(GPIOE, 14)
|
||||
# define E15 PAL_LINE(GPIOE, 15)
|
||||
# define F0 PAL_LINE(GPIOF, 0)
|
||||
# define F1 PAL_LINE(GPIOF, 1)
|
||||
# define F2 PAL_LINE(GPIOF, 2)
|
||||
# define F3 PAL_LINE(GPIOF, 3)
|
||||
# define F4 PAL_LINE(GPIOF, 4)
|
||||
# define F5 PAL_LINE(GPIOF, 5)
|
||||
# define F6 PAL_LINE(GPIOF, 6)
|
||||
# define F7 PAL_LINE(GPIOF, 7)
|
||||
# define F8 PAL_LINE(GPIOF, 8)
|
||||
# define F9 PAL_LINE(GPIOF, 9)
|
||||
# define F10 PAL_LINE(GPIOF, 10)
|
||||
# define F11 PAL_LINE(GPIOF, 11)
|
||||
# define F12 PAL_LINE(GPIOF, 12)
|
||||
# define F13 PAL_LINE(GPIOF, 13)
|
||||
# define F14 PAL_LINE(GPIOF, 14)
|
||||
# define F15 PAL_LINE(GPIOF, 15)
|
||||
# define G0 PAL_LINE(GPIOG, 0)
|
||||
# define G1 PAL_LINE(GPIOG, 1)
|
||||
# define G2 PAL_LINE(GPIOG, 2)
|
||||
# define G3 PAL_LINE(GPIOG, 3)
|
||||
# define G4 PAL_LINE(GPIOG, 4)
|
||||
# define G5 PAL_LINE(GPIOG, 5)
|
||||
# define G6 PAL_LINE(GPIOG, 6)
|
||||
# define G7 PAL_LINE(GPIOG, 7)
|
||||
# define G8 PAL_LINE(GPIOG, 8)
|
||||
# define G9 PAL_LINE(GPIOG, 9)
|
||||
# define G10 PAL_LINE(GPIOG, 10)
|
||||
# define G11 PAL_LINE(GPIOG, 11)
|
||||
# define G12 PAL_LINE(GPIOG, 12)
|
||||
# define G13 PAL_LINE(GPIOG, 13)
|
||||
# define G14 PAL_LINE(GPIOG, 14)
|
||||
# define G15 PAL_LINE(GPIOG, 15)
|
||||
# define H0 PAL_LINE(GPIOH, 0)
|
||||
# define H1 PAL_LINE(GPIOH, 1)
|
||||
# define H2 PAL_LINE(GPIOH, 2)
|
||||
# define H3 PAL_LINE(GPIOH, 3)
|
||||
# define H4 PAL_LINE(GPIOH, 4)
|
||||
# define H5 PAL_LINE(GPIOH, 5)
|
||||
# define H6 PAL_LINE(GPIOH, 6)
|
||||
# define H7 PAL_LINE(GPIOH, 7)
|
||||
# define H8 PAL_LINE(GPIOH, 8)
|
||||
# define H9 PAL_LINE(GPIOH, 9)
|
||||
# define H10 PAL_LINE(GPIOH, 10)
|
||||
# define H11 PAL_LINE(GPIOH, 11)
|
||||
# define H12 PAL_LINE(GPIOH, 12)
|
||||
# define H13 PAL_LINE(GPIOH, 13)
|
||||
# define H14 PAL_LINE(GPIOH, 14)
|
||||
# define H15 PAL_LINE(GPIOH, 15)
|
||||
# define I0 PAL_LINE(GPIOI, 0)
|
||||
# define I1 PAL_LINE(GPIOI, 1)
|
||||
# define I2 PAL_LINE(GPIOI, 2)
|
||||
# define I3 PAL_LINE(GPIOI, 3)
|
||||
# define I4 PAL_LINE(GPIOI, 4)
|
||||
# define I5 PAL_LINE(GPIOI, 5)
|
||||
# define I6 PAL_LINE(GPIOI, 6)
|
||||
# define I7 PAL_LINE(GPIOI, 7)
|
||||
# define I8 PAL_LINE(GPIOI, 8)
|
||||
# define I9 PAL_LINE(GPIOI, 9)
|
||||
# define I10 PAL_LINE(GPIOI, 10)
|
||||
# define I11 PAL_LINE(GPIOI, 11)
|
||||
# define I12 PAL_LINE(GPIOI, 12)
|
||||
# define I13 PAL_LINE(GPIOI, 13)
|
||||
# define I14 PAL_LINE(GPIOI, 14)
|
||||
# define I15 PAL_LINE(GPIOI, 15)
|
||||
# define J0 PAL_LINE(GPIOJ, 0)
|
||||
# define J1 PAL_LINE(GPIOJ, 1)
|
||||
# define J2 PAL_LINE(GPIOJ, 2)
|
||||
# define J3 PAL_LINE(GPIOJ, 3)
|
||||
# define J4 PAL_LINE(GPIOJ, 4)
|
||||
# define J5 PAL_LINE(GPIOJ, 5)
|
||||
# define J6 PAL_LINE(GPIOJ, 6)
|
||||
# define J7 PAL_LINE(GPIOJ, 7)
|
||||
# define J8 PAL_LINE(GPIOJ, 8)
|
||||
# define J9 PAL_LINE(GPIOJ, 9)
|
||||
# define J10 PAL_LINE(GPIOJ, 10)
|
||||
# define J11 PAL_LINE(GPIOJ, 11)
|
||||
# define J12 PAL_LINE(GPIOJ, 12)
|
||||
# define J13 PAL_LINE(GPIOJ, 13)
|
||||
# define J14 PAL_LINE(GPIOJ, 14)
|
||||
# define J15 PAL_LINE(GPIOJ, 15)
|
||||
// Keyboards can `#define KEYBOARD_REQUIRES_GPIOK` if they need to access GPIO-K pins. These conflict with a whole
|
||||
// bunch of layout definitions, so it's intentionally left out unless absolutely required -- in that case, the
|
||||
// keyboard designer should use a different symbol when defining their layout macros.
|
||||
# ifdef KEYBOARD_REQUIRES_GPIOK
|
||||
# define K0 PAL_LINE(GPIOK, 0)
|
||||
# define K1 PAL_LINE(GPIOK, 1)
|
||||
# define K2 PAL_LINE(GPIOK, 2)
|
||||
# define K3 PAL_LINE(GPIOK, 3)
|
||||
# define K4 PAL_LINE(GPIOK, 4)
|
||||
# define K5 PAL_LINE(GPIOK, 5)
|
||||
# define K6 PAL_LINE(GPIOK, 6)
|
||||
# define K7 PAL_LINE(GPIOK, 7)
|
||||
# define K8 PAL_LINE(GPIOK, 8)
|
||||
# define K9 PAL_LINE(GPIOK, 9)
|
||||
# define K10 PAL_LINE(GPIOK, 10)
|
||||
# define K11 PAL_LINE(GPIOK, 11)
|
||||
# define K12 PAL_LINE(GPIOK, 12)
|
||||
# define K13 PAL_LINE(GPIOK, 13)
|
||||
# define K14 PAL_LINE(GPIOK, 14)
|
||||
# define K15 PAL_LINE(GPIOK, 15)
|
||||
# endif
|
||||
# endif
|
||||
#endif
|
||||
|
||||
#define API_SYSEX_MAX_SIZE 32
|
||||
|
||||
#include "song_list.h"
|
||||
|
|
|
@ -27,7 +27,7 @@
|
|||
#include <string.h>
|
||||
#include <math.h>
|
||||
|
||||
led_config_t led_matrix_config;
|
||||
led_eeconfig_t led_matrix_eeconfig;
|
||||
|
||||
#ifndef MAX
|
||||
# define MAX(X, Y) ((X) > (Y) ? (X) : (Y))
|
||||
|
@ -70,40 +70,32 @@ void eeconfig_update_led_matrix(uint32_t config_value) { eeprom_update_dword(EEC
|
|||
|
||||
void eeconfig_update_led_matrix_default(void) {
|
||||
dprintf("eeconfig_update_led_matrix_default\n");
|
||||
led_matrix_config.enable = 1;
|
||||
led_matrix_config.mode = LED_MATRIX_UNIFORM_BRIGHTNESS;
|
||||
led_matrix_config.val = 128;
|
||||
led_matrix_config.speed = 0;
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
led_matrix_eeconfig.enable = 1;
|
||||
led_matrix_eeconfig.mode = LED_MATRIX_UNIFORM_BRIGHTNESS;
|
||||
led_matrix_eeconfig.val = 128;
|
||||
led_matrix_eeconfig.speed = 0;
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void eeconfig_debug_led_matrix(void) {
|
||||
dprintf("led_matrix_config eeprom\n");
|
||||
dprintf("led_matrix_config.enable = %d\n", led_matrix_config.enable);
|
||||
dprintf("led_matrix_config.mode = %d\n", led_matrix_config.mode);
|
||||
dprintf("led_matrix_config.val = %d\n", led_matrix_config.val);
|
||||
dprintf("led_matrix_config.speed = %d\n", led_matrix_config.speed);
|
||||
dprintf("led_matrix_eeconfig eeprom\n");
|
||||
dprintf("led_matrix_eeconfig.enable = %d\n", led_matrix_eeconfig.enable);
|
||||
dprintf("led_matrix_eeconfig.mode = %d\n", led_matrix_eeconfig.mode);
|
||||
dprintf("led_matrix_eeconfig.val = %d\n", led_matrix_eeconfig.val);
|
||||
dprintf("led_matrix_eeconfig.speed = %d\n", led_matrix_eeconfig.speed);
|
||||
}
|
||||
|
||||
// Last led hit
|
||||
#ifndef LED_HITS_TO_REMEMBER
|
||||
# define LED_HITS_TO_REMEMBER 8
|
||||
#endif
|
||||
uint8_t g_last_led_hit[LED_HITS_TO_REMEMBER] = {255};
|
||||
uint8_t g_last_led_count = 0;
|
||||
|
||||
void map_row_column_to_led(uint8_t row, uint8_t column, uint8_t *led_i, uint8_t *led_count) {
|
||||
led_matrix led;
|
||||
*led_count = 0;
|
||||
|
||||
for (uint8_t i = 0; i < LED_DRIVER_LED_COUNT; i++) {
|
||||
// map_index_to_led(i, &led);
|
||||
led = g_leds[i];
|
||||
if (row == led.matrix_co.row && column == led.matrix_co.col) {
|
||||
led_i[*led_count] = i;
|
||||
(*led_count)++;
|
||||
}
|
||||
uint8_t map_row_column_to_led(uint8_t row, uint8_t column, uint8_t *led_i) {
|
||||
uint8_t led_count = 0;
|
||||
uint8_t led_index = g_led_config.matrix_co[row][column];
|
||||
if (led_index != NO_LED) {
|
||||
led_i[led_count] = led_index;
|
||||
led_count++;
|
||||
}
|
||||
return led_count;
|
||||
}
|
||||
|
||||
void led_matrix_update_pwm_buffers(void) { led_matrix_driver.flush(); }
|
||||
|
@ -114,8 +106,8 @@ void led_matrix_set_index_value_all(uint8_t value) { led_matrix_driver.set_value
|
|||
|
||||
bool process_led_matrix(uint16_t keycode, keyrecord_t *record) {
|
||||
if (record->event.pressed) {
|
||||
uint8_t led[8], led_count;
|
||||
map_row_column_to_led(record->event.key.row, record->event.key.col, led, &led_count);
|
||||
uint8_t led[8];
|
||||
uint8_t led_count = map_row_column_to_led(record->event.key.row, record->event.key.col, led);
|
||||
if (led_count > 0) {
|
||||
for (uint8_t i = LED_HITS_TO_REMEMBER; i > 1; i--) {
|
||||
g_last_led_hit[i - 1] = g_last_led_hit[i - 2];
|
||||
|
@ -127,8 +119,8 @@ bool process_led_matrix(uint16_t keycode, keyrecord_t *record) {
|
|||
g_any_key_hit = 0;
|
||||
} else {
|
||||
#ifdef LED_MATRIX_KEYRELEASES
|
||||
uint8_t led[8], led_count;
|
||||
map_row_column_to_led(record->event.key.row, record->event.key.col, led, &led_count);
|
||||
uint8_t led[8];
|
||||
uint8_t led_count = map_row_column_to_led(record->event.key.row, record->event.key.col, led);
|
||||
for (uint8_t i = 0; i < led_count; i++) g_key_hit[led[i]] = 255;
|
||||
|
||||
g_any_key_hit = 255;
|
||||
|
@ -143,12 +135,12 @@ void led_matrix_set_suspend_state(bool state) { g_suspend_state = state; }
|
|||
void led_matrix_all_off(void) { led_matrix_set_index_value_all(0); }
|
||||
|
||||
// Uniform brightness
|
||||
void led_matrix_uniform_brightness(void) { led_matrix_set_index_value_all(LED_MATRIX_MAXIMUM_BRIGHTNESS / BACKLIGHT_LEVELS * led_matrix_config.val); }
|
||||
void led_matrix_uniform_brightness(void) { led_matrix_set_index_value_all(LED_MATRIX_MAXIMUM_BRIGHTNESS / BACKLIGHT_LEVELS * led_matrix_eeconfig.val); }
|
||||
|
||||
void led_matrix_custom(void) {}
|
||||
|
||||
void led_matrix_task(void) {
|
||||
if (!led_matrix_config.enable) {
|
||||
if (!led_matrix_eeconfig.enable) {
|
||||
led_matrix_all_off();
|
||||
led_matrix_indicators();
|
||||
return;
|
||||
|
@ -170,7 +162,7 @@ void led_matrix_task(void) {
|
|||
// Ideally we would also stop sending zeros to the LED driver PWM buffers
|
||||
// while suspended and just do a software shutdown. This is a cheap hack for now.
|
||||
bool suspend_backlight = ((g_suspend_state && LED_DISABLE_WHEN_USB_SUSPENDED) || (LED_DISABLE_AFTER_TIMEOUT > 0 && g_any_key_hit > LED_DISABLE_AFTER_TIMEOUT * 60 * 20));
|
||||
uint8_t effect = suspend_backlight ? 0 : led_matrix_config.mode;
|
||||
uint8_t effect = suspend_backlight ? 0 : led_matrix_eeconfig.mode;
|
||||
|
||||
// this gets ticked at 20 Hz.
|
||||
// each effect can opt to do calculations
|
||||
|
@ -211,8 +203,8 @@ __attribute__((weak)) void led_matrix_indicators_user(void) {}
|
|||
// else
|
||||
// {
|
||||
// // This needs updated to something like
|
||||
// // uint8_t led[8], led_count;
|
||||
// // map_row_column_to_led(row,column,led,&led_count);
|
||||
// // uint8_t led[8];
|
||||
// // uint8_t led_count = map_row_column_to_led(row, column, led);
|
||||
// // for(uint8_t i = 0; i < led_count; i++)
|
||||
// map_row_column_to_led(row, column, index);
|
||||
// }
|
||||
|
@ -235,12 +227,12 @@ void led_matrix_init(void) {
|
|||
eeconfig_update_led_matrix_default();
|
||||
}
|
||||
|
||||
led_matrix_config.raw = eeconfig_read_led_matrix();
|
||||
led_matrix_eeconfig.raw = eeconfig_read_led_matrix();
|
||||
|
||||
if (!led_matrix_config.mode) {
|
||||
dprintf("led_matrix_init_drivers led_matrix_config.mode = 0. Write default values to EEPROM.\n");
|
||||
if (!led_matrix_eeconfig.mode) {
|
||||
dprintf("led_matrix_init_drivers led_matrix_eeconfig.mode = 0. Write default values to EEPROM.\n");
|
||||
eeconfig_update_led_matrix_default();
|
||||
led_matrix_config.raw = eeconfig_read_led_matrix();
|
||||
led_matrix_eeconfig.raw = eeconfig_read_led_matrix();
|
||||
}
|
||||
|
||||
eeconfig_debug_led_matrix(); // display current eeprom values
|
||||
|
@ -270,8 +262,8 @@ static uint8_t decrement(uint8_t value, uint8_t step, uint8_t min, uint8_t max)
|
|||
// }
|
||||
|
||||
// void backlight_set_key_value(uint8_t row, uint8_t column, uint8_t value) {
|
||||
// uint8_t led[8], led_count;
|
||||
// map_row_column_to_led(row,column,led,&led_count);
|
||||
// uint8_t led[8];
|
||||
// uint8_t led_count = map_row_column_to_led(row, column, led);
|
||||
// for(uint8_t i = 0; i < led_count; i++) {
|
||||
// if (led[i] < LED_DRIVER_LED_COUNT) {
|
||||
// void *address = backlight_get_custom_key_value_eeprom_address(led[i]);
|
||||
|
@ -283,74 +275,74 @@ static uint8_t decrement(uint8_t value, uint8_t step, uint8_t min, uint8_t max)
|
|||
uint32_t led_matrix_get_tick(void) { return g_tick; }
|
||||
|
||||
void led_matrix_toggle(void) {
|
||||
led_matrix_config.enable ^= 1;
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
led_matrix_eeconfig.enable ^= 1;
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_enable(void) {
|
||||
led_matrix_config.enable = 1;
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
led_matrix_eeconfig.enable = 1;
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_enable_noeeprom(void) { led_matrix_config.enable = 1; }
|
||||
void led_matrix_enable_noeeprom(void) { led_matrix_eeconfig.enable = 1; }
|
||||
|
||||
void led_matrix_disable(void) {
|
||||
led_matrix_config.enable = 0;
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
led_matrix_eeconfig.enable = 0;
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_disable_noeeprom(void) { led_matrix_config.enable = 0; }
|
||||
void led_matrix_disable_noeeprom(void) { led_matrix_eeconfig.enable = 0; }
|
||||
|
||||
void led_matrix_step(void) {
|
||||
led_matrix_config.mode++;
|
||||
if (led_matrix_config.mode >= LED_MATRIX_EFFECT_MAX) {
|
||||
led_matrix_config.mode = 1;
|
||||
led_matrix_eeconfig.mode++;
|
||||
if (led_matrix_eeconfig.mode >= LED_MATRIX_EFFECT_MAX) {
|
||||
led_matrix_eeconfig.mode = 1;
|
||||
}
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_step_reverse(void) {
|
||||
led_matrix_config.mode--;
|
||||
if (led_matrix_config.mode < 1) {
|
||||
led_matrix_config.mode = LED_MATRIX_EFFECT_MAX - 1;
|
||||
led_matrix_eeconfig.mode--;
|
||||
if (led_matrix_eeconfig.mode < 1) {
|
||||
led_matrix_eeconfig.mode = LED_MATRIX_EFFECT_MAX - 1;
|
||||
}
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_increase_val(void) {
|
||||
led_matrix_config.val = increment(led_matrix_config.val, 8, 0, LED_MATRIX_MAXIMUM_BRIGHTNESS);
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
led_matrix_eeconfig.val = increment(led_matrix_eeconfig.val, 8, 0, LED_MATRIX_MAXIMUM_BRIGHTNESS);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_decrease_val(void) {
|
||||
led_matrix_config.val = decrement(led_matrix_config.val, 8, 0, LED_MATRIX_MAXIMUM_BRIGHTNESS);
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
led_matrix_eeconfig.val = decrement(led_matrix_eeconfig.val, 8, 0, LED_MATRIX_MAXIMUM_BRIGHTNESS);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void led_matrix_increase_speed(void) {
|
||||
led_matrix_config.speed = increment(led_matrix_config.speed, 1, 0, 3);
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw); // EECONFIG needs to be increased to support this
|
||||
led_matrix_eeconfig.speed = increment(led_matrix_eeconfig.speed, 1, 0, 3);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw); // EECONFIG needs to be increased to support this
|
||||
}
|
||||
|
||||
void led_matrix_decrease_speed(void) {
|
||||
led_matrix_config.speed = decrement(led_matrix_config.speed, 1, 0, 3);
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw); // EECONFIG needs to be increased to support this
|
||||
led_matrix_eeconfig.speed = decrement(led_matrix_eeconfig.speed, 1, 0, 3);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw); // EECONFIG needs to be increased to support this
|
||||
}
|
||||
|
||||
void led_matrix_mode(uint8_t mode, bool eeprom_write) {
|
||||
led_matrix_config.mode = mode;
|
||||
led_matrix_eeconfig.mode = mode;
|
||||
if (eeprom_write) {
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
}
|
||||
|
||||
uint8_t led_matrix_get_mode(void) { return led_matrix_config.mode; }
|
||||
uint8_t led_matrix_get_mode(void) { return led_matrix_eeconfig.mode; }
|
||||
|
||||
void led_matrix_set_value_noeeprom(uint8_t val) { led_matrix_config.val = val; }
|
||||
void led_matrix_set_value_noeeprom(uint8_t val) { led_matrix_eeconfig.val = val; }
|
||||
|
||||
void led_matrix_set_value(uint8_t val) {
|
||||
led_matrix_set_value_noeeprom(val);
|
||||
eeconfig_update_led_matrix(led_matrix_config.raw);
|
||||
eeconfig_update_led_matrix(led_matrix_eeconfig.raw);
|
||||
}
|
||||
|
||||
void backlight_set(uint8_t val) { led_matrix_set_value(val); }
|
||||
|
|
|
@ -19,46 +19,12 @@
|
|||
|
||||
#pragma once
|
||||
|
||||
#include "led_matrix_types.h"
|
||||
|
||||
#ifndef BACKLIGHT_ENABLE
|
||||
# error You must define BACKLIGHT_ENABLE with LED_MATRIX_ENABLE
|
||||
#endif
|
||||
|
||||
typedef struct Point {
|
||||
uint8_t x;
|
||||
uint8_t y;
|
||||
} __attribute__((packed)) Point;
|
||||
|
||||
typedef struct led_matrix {
|
||||
union {
|
||||
uint8_t raw;
|
||||
struct {
|
||||
uint8_t row : 4; // 16 max
|
||||
uint8_t col : 4; // 16 max
|
||||
};
|
||||
} matrix_co;
|
||||
Point point;
|
||||
uint8_t modifier : 1;
|
||||
} __attribute__((packed)) led_matrix;
|
||||
|
||||
extern const led_matrix g_leds[LED_DRIVER_LED_COUNT];
|
||||
|
||||
typedef struct {
|
||||
uint8_t index;
|
||||
uint8_t value;
|
||||
} led_indicator;
|
||||
|
||||
typedef union {
|
||||
uint32_t raw;
|
||||
struct {
|
||||
bool enable : 1;
|
||||
uint8_t mode : 6;
|
||||
uint8_t hue : 8; // Unused by led_matrix
|
||||
uint8_t sat : 8; // Unused by led_matrix
|
||||
uint8_t val : 8;
|
||||
uint8_t speed : 8; // EECONFIG needs to be increased to support this
|
||||
};
|
||||
} led_config_t;
|
||||
|
||||
enum led_matrix_effects {
|
||||
LED_MATRIX_UNIFORM_BRIGHTNESS = 1,
|
||||
// All new effects go above this line
|
||||
|
@ -122,3 +88,7 @@ typedef struct {
|
|||
} led_matrix_driver_t;
|
||||
|
||||
extern const led_matrix_driver_t led_matrix_driver;
|
||||
|
||||
extern led_eeconfig_t led_matrix_eeconfig;
|
||||
|
||||
extern led_config_t g_led_config;
|
||||
|
|
|
@ -0,0 +1,69 @@
|
|||
/* Copyright 2021
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <stdint.h>
|
||||
#include <stdbool.h>
|
||||
|
||||
#if defined(__GNUC__)
|
||||
# define PACKED __attribute__((__packed__))
|
||||
#else
|
||||
# define PACKED
|
||||
#endif
|
||||
|
||||
#if defined(_MSC_VER)
|
||||
# pragma pack(push, 1)
|
||||
#endif
|
||||
|
||||
// Last led hit
|
||||
#ifndef LED_HITS_TO_REMEMBER
|
||||
# define LED_HITS_TO_REMEMBER 8
|
||||
#endif // LED_HITS_TO_REMEMBER
|
||||
|
||||
typedef struct PACKED {
|
||||
uint8_t x;
|
||||
uint8_t y;
|
||||
} point_t;
|
||||
|
||||
#define LED_FLAG_ALL 0xFF
|
||||
#define LED_FLAG_NONE 0x00
|
||||
#define LED_FLAG_MODIFIER 0x01
|
||||
#define LED_FLAG_KEYLIGHT 0x04
|
||||
#define LED_FLAG_INDICATOR 0x08
|
||||
|
||||
#define NO_LED 255
|
||||
|
||||
typedef struct PACKED {
|
||||
uint8_t matrix_co[MATRIX_ROWS][MATRIX_COLS];
|
||||
point_t point[LED_DRIVER_LED_COUNT];
|
||||
uint8_t flags[LED_DRIVER_LED_COUNT];
|
||||
} led_config_t;
|
||||
|
||||
typedef union {
|
||||
uint32_t raw;
|
||||
struct PACKED {
|
||||
uint8_t enable : 2;
|
||||
uint8_t mode : 6;
|
||||
uint16_t reserved;
|
||||
uint8_t val;
|
||||
uint8_t speed; // EECONFIG needs to be increased to support this
|
||||
};
|
||||
} led_eeconfig_t;
|
||||
|
||||
#if defined(_MSC_VER)
|
||||
# pragma pack(pop)
|
||||
#endif
|
|
@ -55,6 +55,8 @@
|
|||
#include "timer.h"
|
||||
#include "sync_timer.h"
|
||||
#include "config_common.h"
|
||||
#include "gpio.h"
|
||||
#include "atomic_util.h"
|
||||
#include "led.h"
|
||||
#include "action_util.h"
|
||||
#include "action_tapping.h"
|
||||
|
@ -199,20 +201,6 @@ extern layer_state_t layer_state;
|
|||
|
||||
// Function substitutions to ease GPIO manipulation
|
||||
#if defined(__AVR__)
|
||||
typedef uint8_t pin_t;
|
||||
|
||||
# define setPinInput(pin) (DDRx_ADDRESS(pin) &= ~_BV((pin)&0xF), PORTx_ADDRESS(pin) &= ~_BV((pin)&0xF))
|
||||
# define setPinInputHigh(pin) (DDRx_ADDRESS(pin) &= ~_BV((pin)&0xF), PORTx_ADDRESS(pin) |= _BV((pin)&0xF))
|
||||
# define setPinInputLow(pin) _Static_assert(0, "AVR processors cannot implement an input as pull low")
|
||||
# define setPinOutput(pin) (DDRx_ADDRESS(pin) |= _BV((pin)&0xF))
|
||||
|
||||
# define writePinHigh(pin) (PORTx_ADDRESS(pin) |= _BV((pin)&0xF))
|
||||
# define writePinLow(pin) (PORTx_ADDRESS(pin) &= ~_BV((pin)&0xF))
|
||||
# define writePin(pin, level) ((level) ? writePinHigh(pin) : writePinLow(pin))
|
||||
|
||||
# define readPin(pin) ((bool)(PINx_ADDRESS(pin) & _BV((pin)&0xF)))
|
||||
|
||||
# define togglePin(pin) (PORTx_ADDRESS(pin) ^= _BV((pin)&0xF))
|
||||
|
||||
/* The AVR series GPIOs have a one clock read delay for changes in the digital input signal.
|
||||
* But here's more margin to make it two clocks. */
|
||||
|
@ -221,25 +209,8 @@ typedef uint8_t pin_t;
|
|||
# endif
|
||||
# define waitInputPinDelay() wait_cpuclock(GPIO_INPUT_PIN_DELAY)
|
||||
|
||||
#elif defined(PROTOCOL_CHIBIOS)
|
||||
typedef ioline_t pin_t;
|
||||
#elif defined(__ARMEL__) || defined(__ARMEB__)
|
||||
|
||||
# define setPinInput(pin) palSetLineMode(pin, PAL_MODE_INPUT)
|
||||
# define setPinInputHigh(pin) palSetLineMode(pin, PAL_MODE_INPUT_PULLUP)
|
||||
# define setPinInputLow(pin) palSetLineMode(pin, PAL_MODE_INPUT_PULLDOWN)
|
||||
# define setPinOutput(pin) palSetLineMode(pin, PAL_MODE_OUTPUT_PUSHPULL)
|
||||
|
||||
# define writePinHigh(pin) palSetLine(pin)
|
||||
# define writePinLow(pin) palClearLine(pin)
|
||||
# define writePin(pin, level) ((level) ? (writePinHigh(pin)) : (writePinLow(pin)))
|
||||
|
||||
# define readPin(pin) palReadLine(pin)
|
||||
|
||||
# define togglePin(pin) palToggleLine(pin)
|
||||
|
||||
#endif
|
||||
|
||||
#if defined(__ARMEL__) || defined(__ARMEB__)
|
||||
/* For GPIOs on ARM-based MCUs, the input pins are sampled by the clock of the bus
|
||||
* to which the GPIO is connected.
|
||||
* The connected buses differ depending on the various series of MCUs.
|
||||
|
@ -258,63 +229,8 @@ typedef ioline_t pin_t;
|
|||
# endif
|
||||
# endif
|
||||
# define waitInputPinDelay() wait_cpuclock(GPIO_INPUT_PIN_DELAY)
|
||||
#endif
|
||||
|
||||
// Atomic macro to help make GPIO and other controls atomic.
|
||||
#ifdef IGNORE_ATOMIC_BLOCK
|
||||
/* do nothing atomic macro */
|
||||
# define ATOMIC_BLOCK for (uint8_t __ToDo = 1; __ToDo; __ToDo = 0)
|
||||
# define ATOMIC_BLOCK_RESTORESTATE ATOMIC_BLOCK
|
||||
# define ATOMIC_BLOCK_FORCEON ATOMIC_BLOCK
|
||||
|
||||
#elif defined(__AVR__)
|
||||
/* atomic macro for AVR */
|
||||
# include <util/atomic.h>
|
||||
|
||||
# define ATOMIC_BLOCK_RESTORESTATE ATOMIC_BLOCK(ATOMIC_RESTORESTATE)
|
||||
# define ATOMIC_BLOCK_FORCEON ATOMIC_BLOCK(ATOMIC_FORCEON)
|
||||
|
||||
#elif defined(PROTOCOL_CHIBIOS) || defined(PROTOCOL_ARM_ATSAM)
|
||||
/* atomic macro for ChibiOS / ARM ATSAM */
|
||||
# if defined(PROTOCOL_ARM_ATSAM)
|
||||
# include "arm_atsam_protocol.h"
|
||||
# endif
|
||||
|
||||
static __inline__ uint8_t __interrupt_disable__(void) {
|
||||
# if defined(PROTOCOL_CHIBIOS)
|
||||
chSysLock();
|
||||
# endif
|
||||
# if defined(PROTOCOL_ARM_ATSAM)
|
||||
__disable_irq();
|
||||
# endif
|
||||
return 1;
|
||||
}
|
||||
|
||||
static __inline__ void __interrupt_enable__(const uint8_t *__s) {
|
||||
# if defined(PROTOCOL_CHIBIOS)
|
||||
chSysUnlock();
|
||||
# endif
|
||||
# if defined(PROTOCOL_ARM_ATSAM)
|
||||
__enable_irq();
|
||||
# endif
|
||||
__asm__ volatile("" ::: "memory");
|
||||
(void)__s;
|
||||
}
|
||||
|
||||
# define ATOMIC_BLOCK(type) for (type, __ToDo = __interrupt_disable__(); __ToDo; __ToDo = 0)
|
||||
# define ATOMIC_FORCEON uint8_t sreg_save __attribute__((__cleanup__(__interrupt_enable__))) = 0
|
||||
|
||||
# define ATOMIC_BLOCK_RESTORESTATE _Static_assert(0, "ATOMIC_BLOCK_RESTORESTATE dose not implement")
|
||||
# define ATOMIC_BLOCK_FORCEON ATOMIC_BLOCK(ATOMIC_FORCEON)
|
||||
|
||||
/* Other platform */
|
||||
#else
|
||||
|
||||
# define ATOMIC_BLOCK_RESTORESTATE _Static_assert(0, "ATOMIC_BLOCK_RESTORESTATE dose not implement")
|
||||
# define ATOMIC_BLOCK_FORCEON _Static_assert(0, "ATOMIC_BLOCK_FORCEON dose not implement")
|
||||
|
||||
#endif
|
||||
|
||||
#define SEND_STRING(string) send_string_P(PSTR(string))
|
||||
#define SEND_STRING_DELAY(string, interval) send_string_with_delay_P(PSTR(string), interval)
|
||||
|
||||
|
|
|
@ -1,3 +1,19 @@
|
|||
/* Copyright 2021
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
|
||||
#include <stdint.h>
|
||||
|
|
|
@ -0,0 +1,32 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
// Macro to help make GPIO and other controls atomic.
|
||||
|
||||
#ifndef IGNORE_ATOMIC_BLOCK
|
||||
# if __has_include_next("atomic_util.h")
|
||||
# include_next "atomic_util.h" /* Include the platforms atomic.h */
|
||||
# else
|
||||
# define ATOMIC_BLOCK _Static_assert(0, "ATOMIC_BLOCK not implemented")
|
||||
# define ATOMIC_BLOCK_RESTORESTATE _Static_assert(0, "ATOMIC_BLOCK_RESTORESTATE not implemented")
|
||||
# define ATOMIC_BLOCK_FORCEON _Static_assert(0, "ATOMIC_BLOCK_FORCEON not implemented")
|
||||
# endif
|
||||
#else /* do nothing atomic macro */
|
||||
# define ATOMIC_BLOCK for (uint8_t __ToDo = 1; __ToDo; __ToDo = 0)
|
||||
# define ATOMIC_BLOCK_RESTORESTATE ATOMIC_BLOCK
|
||||
# define ATOMIC_BLOCK_FORCEON ATOMIC_BLOCK
|
||||
#endif
|
|
@ -0,0 +1,22 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
/* atomic macro for AVR */
|
||||
#include <util/atomic.h>
|
||||
|
||||
#define ATOMIC_BLOCK_RESTORESTATE ATOMIC_BLOCK(ATOMIC_RESTORESTATE)
|
||||
#define ATOMIC_BLOCK_FORCEON ATOMIC_BLOCK(ATOMIC_FORCEON)
|
|
@ -0,0 +1,34 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <avr/io.h>
|
||||
#include "pin_defs.h"
|
||||
|
||||
typedef uint8_t pin_t;
|
||||
|
||||
#define setPinInput(pin) (DDRx_ADDRESS(pin) &= ~_BV((pin)&0xF), PORTx_ADDRESS(pin) &= ~_BV((pin)&0xF))
|
||||
#define setPinInputHigh(pin) (DDRx_ADDRESS(pin) &= ~_BV((pin)&0xF), PORTx_ADDRESS(pin) |= _BV((pin)&0xF))
|
||||
#define setPinInputLow(pin) _Static_assert(0, "AVR processors cannot implement an input as pull low")
|
||||
#define setPinOutput(pin) (DDRx_ADDRESS(pin) |= _BV((pin)&0xF))
|
||||
|
||||
#define writePinHigh(pin) (PORTx_ADDRESS(pin) |= _BV((pin)&0xF))
|
||||
#define writePinLow(pin) (PORTx_ADDRESS(pin) &= ~_BV((pin)&0xF))
|
||||
#define writePin(pin, level) ((level) ? writePinHigh(pin) : writePinLow(pin))
|
||||
|
||||
#define readPin(pin) ((bool)(PINx_ADDRESS(pin) & _BV((pin)&0xF)))
|
||||
|
||||
#define togglePin(pin) (PORTx_ADDRESS(pin) ^= _BV((pin)&0xF))
|
|
@ -0,0 +1,128 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <avr/io.h>
|
||||
|
||||
#define PORT_SHIFTER 4 // this may be 4 for all AVR chips
|
||||
|
||||
// If you want to add more to this list, reference the PINx definitions in these header
|
||||
// files: https://github.com/vancegroup-mirrors/avr-libc/tree/master/avr-libc/include/avr
|
||||
|
||||
#if defined(__AVR_ATmega32U4__) || defined(__AVR_ATmega16U4__)
|
||||
# define ADDRESS_BASE 0x00
|
||||
# define PINB_ADDRESS 0x3
|
||||
# define PINC_ADDRESS 0x6
|
||||
# define PIND_ADDRESS 0x9
|
||||
# define PINE_ADDRESS 0xC
|
||||
# define PINF_ADDRESS 0xF
|
||||
#elif defined(__AVR_AT90USB162__) || defined(__AVR_ATmega32U2__) || defined(__AVR_ATmega16U2__) || defined(__AVR_ATmega328P__) || defined(__AVR_ATmega328__)
|
||||
# define ADDRESS_BASE 0x00
|
||||
# define PINB_ADDRESS 0x3
|
||||
# define PINC_ADDRESS 0x6
|
||||
# define PIND_ADDRESS 0x9
|
||||
#elif defined(__AVR_AT90USB646__) || defined(__AVR_AT90USB647__) || defined(__AVR_AT90USB1286__) || defined(__AVR_AT90USB1287__)
|
||||
# define ADDRESS_BASE 0x00
|
||||
# define PINA_ADDRESS 0x0
|
||||
# define PINB_ADDRESS 0x3
|
||||
# define PINC_ADDRESS 0x6
|
||||
# define PIND_ADDRESS 0x9
|
||||
# define PINE_ADDRESS 0xC
|
||||
# define PINF_ADDRESS 0xF
|
||||
#elif defined(__AVR_ATmega32A__)
|
||||
# define ADDRESS_BASE 0x10
|
||||
# define PIND_ADDRESS 0x0
|
||||
# define PINC_ADDRESS 0x3
|
||||
# define PINB_ADDRESS 0x6
|
||||
# define PINA_ADDRESS 0x9
|
||||
#elif defined(__AVR_ATtiny85__)
|
||||
# define ADDRESS_BASE 0x10
|
||||
# define PINB_ADDRESS 0x6
|
||||
#else
|
||||
# error "Pins are not defined"
|
||||
#endif
|
||||
|
||||
#define PINDEF(port, pin) ((PIN##port##_ADDRESS << PORT_SHIFTER) | pin)
|
||||
|
||||
#define _PIN_ADDRESS(p, offset) _SFR_IO8(ADDRESS_BASE + ((p) >> PORT_SHIFTER) + (offset))
|
||||
// Port X Input Pins Address
|
||||
#define PINx_ADDRESS(p) _PIN_ADDRESS(p, 0)
|
||||
// Port X Data Direction Register, 0:input 1:output
|
||||
#define DDRx_ADDRESS(p) _PIN_ADDRESS(p, 1)
|
||||
// Port X Data Register
|
||||
#define PORTx_ADDRESS(p) _PIN_ADDRESS(p, 2)
|
||||
|
||||
/* I/O pins */
|
||||
#ifdef PORTA
|
||||
# define A0 PINDEF(A, 0)
|
||||
# define A1 PINDEF(A, 1)
|
||||
# define A2 PINDEF(A, 2)
|
||||
# define A3 PINDEF(A, 3)
|
||||
# define A4 PINDEF(A, 4)
|
||||
# define A5 PINDEF(A, 5)
|
||||
# define A6 PINDEF(A, 6)
|
||||
# define A7 PINDEF(A, 7)
|
||||
#endif
|
||||
#ifdef PORTB
|
||||
# define B0 PINDEF(B, 0)
|
||||
# define B1 PINDEF(B, 1)
|
||||
# define B2 PINDEF(B, 2)
|
||||
# define B3 PINDEF(B, 3)
|
||||
# define B4 PINDEF(B, 4)
|
||||
# define B5 PINDEF(B, 5)
|
||||
# define B6 PINDEF(B, 6)
|
||||
# define B7 PINDEF(B, 7)
|
||||
#endif
|
||||
#ifdef PORTC
|
||||
# define C0 PINDEF(C, 0)
|
||||
# define C1 PINDEF(C, 1)
|
||||
# define C2 PINDEF(C, 2)
|
||||
# define C3 PINDEF(C, 3)
|
||||
# define C4 PINDEF(C, 4)
|
||||
# define C5 PINDEF(C, 5)
|
||||
# define C6 PINDEF(C, 6)
|
||||
# define C7 PINDEF(C, 7)
|
||||
#endif
|
||||
#ifdef PORTD
|
||||
# define D0 PINDEF(D, 0)
|
||||
# define D1 PINDEF(D, 1)
|
||||
# define D2 PINDEF(D, 2)
|
||||
# define D3 PINDEF(D, 3)
|
||||
# define D4 PINDEF(D, 4)
|
||||
# define D5 PINDEF(D, 5)
|
||||
# define D6 PINDEF(D, 6)
|
||||
# define D7 PINDEF(D, 7)
|
||||
#endif
|
||||
#ifdef PORTE
|
||||
# define E0 PINDEF(E, 0)
|
||||
# define E1 PINDEF(E, 1)
|
||||
# define E2 PINDEF(E, 2)
|
||||
# define E3 PINDEF(E, 3)
|
||||
# define E4 PINDEF(E, 4)
|
||||
# define E5 PINDEF(E, 5)
|
||||
# define E6 PINDEF(E, 6)
|
||||
# define E7 PINDEF(E, 7)
|
||||
#endif
|
||||
#ifdef PORTF
|
||||
# define F0 PINDEF(F, 0)
|
||||
# define F1 PINDEF(F, 1)
|
||||
# define F2 PINDEF(F, 2)
|
||||
# define F3 PINDEF(F, 3)
|
||||
# define F4 PINDEF(F, 4)
|
||||
# define F5 PINDEF(F, 5)
|
||||
# define F6 PINDEF(F, 6)
|
||||
# define F7 PINDEF(F, 7)
|
||||
#endif
|
|
@ -0,0 +1,37 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <ch.h>
|
||||
|
||||
static __inline__ uint8_t __interrupt_disable__(void) {
|
||||
chSysLock();
|
||||
|
||||
return 1;
|
||||
}
|
||||
|
||||
static __inline__ void __interrupt_enable__(const uint8_t *__s) {
|
||||
chSysUnlock();
|
||||
|
||||
__asm__ volatile("" ::: "memory");
|
||||
(void)__s;
|
||||
}
|
||||
|
||||
#define ATOMIC_BLOCK(type) for (type, __ToDo = __interrupt_disable__(); __ToDo; __ToDo = 0)
|
||||
#define ATOMIC_FORCEON uint8_t sreg_save __attribute__((__cleanup__(__interrupt_enable__))) = 0
|
||||
|
||||
#define ATOMIC_BLOCK_RESTORESTATE _Static_assert(0, "ATOMIC_BLOCK_RESTORESTATE not implemented")
|
||||
#define ATOMIC_BLOCK_FORCEON ATOMIC_BLOCK(ATOMIC_FORCEON)
|
|
@ -0,0 +1,34 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include <hal.h>
|
||||
#include "pin_defs.h"
|
||||
|
||||
typedef ioline_t pin_t;
|
||||
|
||||
#define setPinInput(pin) palSetLineMode(pin, PAL_MODE_INPUT)
|
||||
#define setPinInputHigh(pin) palSetLineMode(pin, PAL_MODE_INPUT_PULLUP)
|
||||
#define setPinInputLow(pin) palSetLineMode(pin, PAL_MODE_INPUT_PULLDOWN)
|
||||
#define setPinOutput(pin) palSetLineMode(pin, PAL_MODE_OUTPUT_PUSHPULL)
|
||||
|
||||
#define writePinHigh(pin) palSetLine(pin)
|
||||
#define writePinLow(pin) palClearLine(pin)
|
||||
#define writePin(pin, level) ((level) ? (writePinHigh(pin)) : (writePinLow(pin)))
|
||||
|
||||
#define readPin(pin) palReadLine(pin)
|
||||
|
||||
#define togglePin(pin) palToggleLine(pin)
|
|
@ -0,0 +1,242 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
// Defines mapping for Proton C replacement
|
||||
#ifdef CONVERT_TO_PROTON_C
|
||||
// Left side (front)
|
||||
# define D3 PAL_LINE(GPIOA, 9)
|
||||
# define D2 PAL_LINE(GPIOA, 10)
|
||||
// GND
|
||||
// GND
|
||||
# define D1 PAL_LINE(GPIOB, 7)
|
||||
# define D0 PAL_LINE(GPIOB, 6)
|
||||
# define D4 PAL_LINE(GPIOB, 5)
|
||||
# define C6 PAL_LINE(GPIOB, 4)
|
||||
# define D7 PAL_LINE(GPIOB, 3)
|
||||
# define E6 PAL_LINE(GPIOB, 2)
|
||||
# define B4 PAL_LINE(GPIOB, 1)
|
||||
# define B5 PAL_LINE(GPIOB, 0)
|
||||
|
||||
// Right side (front)
|
||||
// RAW
|
||||
// GND
|
||||
// RESET
|
||||
// VCC
|
||||
# define F4 PAL_LINE(GPIOA, 2)
|
||||
# define F5 PAL_LINE(GPIOA, 1)
|
||||
# define F6 PAL_LINE(GPIOA, 0)
|
||||
# define F7 PAL_LINE(GPIOB, 8)
|
||||
# define B1 PAL_LINE(GPIOB, 13)
|
||||
# define B3 PAL_LINE(GPIOB, 14)
|
||||
# define B2 PAL_LINE(GPIOB, 15)
|
||||
# define B6 PAL_LINE(GPIOB, 9)
|
||||
|
||||
// LEDs (only D5/C13 uses an actual LED)
|
||||
# ifdef CONVERT_TO_PROTON_C_RXLED
|
||||
# define D5 PAL_LINE(GPIOC, 14)
|
||||
# define B0 PAL_LINE(GPIOC, 13)
|
||||
# else
|
||||
# define D5 PAL_LINE(GPIOC, 13)
|
||||
# define B0 PAL_LINE(GPIOC, 14)
|
||||
# endif
|
||||
#else
|
||||
# define A0 PAL_LINE(GPIOA, 0)
|
||||
# define A1 PAL_LINE(GPIOA, 1)
|
||||
# define A2 PAL_LINE(GPIOA, 2)
|
||||
# define A3 PAL_LINE(GPIOA, 3)
|
||||
# define A4 PAL_LINE(GPIOA, 4)
|
||||
# define A5 PAL_LINE(GPIOA, 5)
|
||||
# define A6 PAL_LINE(GPIOA, 6)
|
||||
# define A7 PAL_LINE(GPIOA, 7)
|
||||
# define A8 PAL_LINE(GPIOA, 8)
|
||||
# define A9 PAL_LINE(GPIOA, 9)
|
||||
# define A10 PAL_LINE(GPIOA, 10)
|
||||
# define A11 PAL_LINE(GPIOA, 11)
|
||||
# define A12 PAL_LINE(GPIOA, 12)
|
||||
# define A13 PAL_LINE(GPIOA, 13)
|
||||
# define A14 PAL_LINE(GPIOA, 14)
|
||||
# define A15 PAL_LINE(GPIOA, 15)
|
||||
# define B0 PAL_LINE(GPIOB, 0)
|
||||
# define B1 PAL_LINE(GPIOB, 1)
|
||||
# define B2 PAL_LINE(GPIOB, 2)
|
||||
# define B3 PAL_LINE(GPIOB, 3)
|
||||
# define B4 PAL_LINE(GPIOB, 4)
|
||||
# define B5 PAL_LINE(GPIOB, 5)
|
||||
# define B6 PAL_LINE(GPIOB, 6)
|
||||
# define B7 PAL_LINE(GPIOB, 7)
|
||||
# define B8 PAL_LINE(GPIOB, 8)
|
||||
# define B9 PAL_LINE(GPIOB, 9)
|
||||
# define B10 PAL_LINE(GPIOB, 10)
|
||||
# define B11 PAL_LINE(GPIOB, 11)
|
||||
# define B12 PAL_LINE(GPIOB, 12)
|
||||
# define B13 PAL_LINE(GPIOB, 13)
|
||||
# define B14 PAL_LINE(GPIOB, 14)
|
||||
# define B15 PAL_LINE(GPIOB, 15)
|
||||
# define B16 PAL_LINE(GPIOB, 16)
|
||||
# define B17 PAL_LINE(GPIOB, 17)
|
||||
# define B18 PAL_LINE(GPIOB, 18)
|
||||
# define B19 PAL_LINE(GPIOB, 19)
|
||||
# define C0 PAL_LINE(GPIOC, 0)
|
||||
# define C1 PAL_LINE(GPIOC, 1)
|
||||
# define C2 PAL_LINE(GPIOC, 2)
|
||||
# define C3 PAL_LINE(GPIOC, 3)
|
||||
# define C4 PAL_LINE(GPIOC, 4)
|
||||
# define C5 PAL_LINE(GPIOC, 5)
|
||||
# define C6 PAL_LINE(GPIOC, 6)
|
||||
# define C7 PAL_LINE(GPIOC, 7)
|
||||
# define C8 PAL_LINE(GPIOC, 8)
|
||||
# define C9 PAL_LINE(GPIOC, 9)
|
||||
# define C10 PAL_LINE(GPIOC, 10)
|
||||
# define C11 PAL_LINE(GPIOC, 11)
|
||||
# define C12 PAL_LINE(GPIOC, 12)
|
||||
# define C13 PAL_LINE(GPIOC, 13)
|
||||
# define C14 PAL_LINE(GPIOC, 14)
|
||||
# define C15 PAL_LINE(GPIOC, 15)
|
||||
# define D0 PAL_LINE(GPIOD, 0)
|
||||
# define D1 PAL_LINE(GPIOD, 1)
|
||||
# define D2 PAL_LINE(GPIOD, 2)
|
||||
# define D3 PAL_LINE(GPIOD, 3)
|
||||
# define D4 PAL_LINE(GPIOD, 4)
|
||||
# define D5 PAL_LINE(GPIOD, 5)
|
||||
# define D6 PAL_LINE(GPIOD, 6)
|
||||
# define D7 PAL_LINE(GPIOD, 7)
|
||||
# define D8 PAL_LINE(GPIOD, 8)
|
||||
# define D9 PAL_LINE(GPIOD, 9)
|
||||
# define D10 PAL_LINE(GPIOD, 10)
|
||||
# define D11 PAL_LINE(GPIOD, 11)
|
||||
# define D12 PAL_LINE(GPIOD, 12)
|
||||
# define D13 PAL_LINE(GPIOD, 13)
|
||||
# define D14 PAL_LINE(GPIOD, 14)
|
||||
# define D15 PAL_LINE(GPIOD, 15)
|
||||
# define E0 PAL_LINE(GPIOE, 0)
|
||||
# define E1 PAL_LINE(GPIOE, 1)
|
||||
# define E2 PAL_LINE(GPIOE, 2)
|
||||
# define E3 PAL_LINE(GPIOE, 3)
|
||||
# define E4 PAL_LINE(GPIOE, 4)
|
||||
# define E5 PAL_LINE(GPIOE, 5)
|
||||
# define E6 PAL_LINE(GPIOE, 6)
|
||||
# define E7 PAL_LINE(GPIOE, 7)
|
||||
# define E8 PAL_LINE(GPIOE, 8)
|
||||
# define E9 PAL_LINE(GPIOE, 9)
|
||||
# define E10 PAL_LINE(GPIOE, 10)
|
||||
# define E11 PAL_LINE(GPIOE, 11)
|
||||
# define E12 PAL_LINE(GPIOE, 12)
|
||||
# define E13 PAL_LINE(GPIOE, 13)
|
||||
# define E14 PAL_LINE(GPIOE, 14)
|
||||
# define E15 PAL_LINE(GPIOE, 15)
|
||||
# define F0 PAL_LINE(GPIOF, 0)
|
||||
# define F1 PAL_LINE(GPIOF, 1)
|
||||
# define F2 PAL_LINE(GPIOF, 2)
|
||||
# define F3 PAL_LINE(GPIOF, 3)
|
||||
# define F4 PAL_LINE(GPIOF, 4)
|
||||
# define F5 PAL_LINE(GPIOF, 5)
|
||||
# define F6 PAL_LINE(GPIOF, 6)
|
||||
# define F7 PAL_LINE(GPIOF, 7)
|
||||
# define F8 PAL_LINE(GPIOF, 8)
|
||||
# define F9 PAL_LINE(GPIOF, 9)
|
||||
# define F10 PAL_LINE(GPIOF, 10)
|
||||
# define F11 PAL_LINE(GPIOF, 11)
|
||||
# define F12 PAL_LINE(GPIOF, 12)
|
||||
# define F13 PAL_LINE(GPIOF, 13)
|
||||
# define F14 PAL_LINE(GPIOF, 14)
|
||||
# define F15 PAL_LINE(GPIOF, 15)
|
||||
# define G0 PAL_LINE(GPIOG, 0)
|
||||
# define G1 PAL_LINE(GPIOG, 1)
|
||||
# define G2 PAL_LINE(GPIOG, 2)
|
||||
# define G3 PAL_LINE(GPIOG, 3)
|
||||
# define G4 PAL_LINE(GPIOG, 4)
|
||||
# define G5 PAL_LINE(GPIOG, 5)
|
||||
# define G6 PAL_LINE(GPIOG, 6)
|
||||
# define G7 PAL_LINE(GPIOG, 7)
|
||||
# define G8 PAL_LINE(GPIOG, 8)
|
||||
# define G9 PAL_LINE(GPIOG, 9)
|
||||
# define G10 PAL_LINE(GPIOG, 10)
|
||||
# define G11 PAL_LINE(GPIOG, 11)
|
||||
# define G12 PAL_LINE(GPIOG, 12)
|
||||
# define G13 PAL_LINE(GPIOG, 13)
|
||||
# define G14 PAL_LINE(GPIOG, 14)
|
||||
# define G15 PAL_LINE(GPIOG, 15)
|
||||
# define H0 PAL_LINE(GPIOH, 0)
|
||||
# define H1 PAL_LINE(GPIOH, 1)
|
||||
# define H2 PAL_LINE(GPIOH, 2)
|
||||
# define H3 PAL_LINE(GPIOH, 3)
|
||||
# define H4 PAL_LINE(GPIOH, 4)
|
||||
# define H5 PAL_LINE(GPIOH, 5)
|
||||
# define H6 PAL_LINE(GPIOH, 6)
|
||||
# define H7 PAL_LINE(GPIOH, 7)
|
||||
# define H8 PAL_LINE(GPIOH, 8)
|
||||
# define H9 PAL_LINE(GPIOH, 9)
|
||||
# define H10 PAL_LINE(GPIOH, 10)
|
||||
# define H11 PAL_LINE(GPIOH, 11)
|
||||
# define H12 PAL_LINE(GPIOH, 12)
|
||||
# define H13 PAL_LINE(GPIOH, 13)
|
||||
# define H14 PAL_LINE(GPIOH, 14)
|
||||
# define H15 PAL_LINE(GPIOH, 15)
|
||||
# define I0 PAL_LINE(GPIOI, 0)
|
||||
# define I1 PAL_LINE(GPIOI, 1)
|
||||
# define I2 PAL_LINE(GPIOI, 2)
|
||||
# define I3 PAL_LINE(GPIOI, 3)
|
||||
# define I4 PAL_LINE(GPIOI, 4)
|
||||
# define I5 PAL_LINE(GPIOI, 5)
|
||||
# define I6 PAL_LINE(GPIOI, 6)
|
||||
# define I7 PAL_LINE(GPIOI, 7)
|
||||
# define I8 PAL_LINE(GPIOI, 8)
|
||||
# define I9 PAL_LINE(GPIOI, 9)
|
||||
# define I10 PAL_LINE(GPIOI, 10)
|
||||
# define I11 PAL_LINE(GPIOI, 11)
|
||||
# define I12 PAL_LINE(GPIOI, 12)
|
||||
# define I13 PAL_LINE(GPIOI, 13)
|
||||
# define I14 PAL_LINE(GPIOI, 14)
|
||||
# define I15 PAL_LINE(GPIOI, 15)
|
||||
# define J0 PAL_LINE(GPIOJ, 0)
|
||||
# define J1 PAL_LINE(GPIOJ, 1)
|
||||
# define J2 PAL_LINE(GPIOJ, 2)
|
||||
# define J3 PAL_LINE(GPIOJ, 3)
|
||||
# define J4 PAL_LINE(GPIOJ, 4)
|
||||
# define J5 PAL_LINE(GPIOJ, 5)
|
||||
# define J6 PAL_LINE(GPIOJ, 6)
|
||||
# define J7 PAL_LINE(GPIOJ, 7)
|
||||
# define J8 PAL_LINE(GPIOJ, 8)
|
||||
# define J9 PAL_LINE(GPIOJ, 9)
|
||||
# define J10 PAL_LINE(GPIOJ, 10)
|
||||
# define J11 PAL_LINE(GPIOJ, 11)
|
||||
# define J12 PAL_LINE(GPIOJ, 12)
|
||||
# define J13 PAL_LINE(GPIOJ, 13)
|
||||
# define J14 PAL_LINE(GPIOJ, 14)
|
||||
# define J15 PAL_LINE(GPIOJ, 15)
|
||||
// Keyboards can `#define KEYBOARD_REQUIRES_GPIOK` if they need to access GPIO-K pins. These conflict with a whole
|
||||
// bunch of layout definitions, so it's intentionally left out unless absolutely required -- in that case, the
|
||||
// keyboard designer should use a different symbol when defining their layout macros.
|
||||
# ifdef KEYBOARD_REQUIRES_GPIOK
|
||||
# define K0 PAL_LINE(GPIOK, 0)
|
||||
# define K1 PAL_LINE(GPIOK, 1)
|
||||
# define K2 PAL_LINE(GPIOK, 2)
|
||||
# define K3 PAL_LINE(GPIOK, 3)
|
||||
# define K4 PAL_LINE(GPIOK, 4)
|
||||
# define K5 PAL_LINE(GPIOK, 5)
|
||||
# define K6 PAL_LINE(GPIOK, 6)
|
||||
# define K7 PAL_LINE(GPIOK, 7)
|
||||
# define K8 PAL_LINE(GPIOK, 8)
|
||||
# define K9 PAL_LINE(GPIOK, 9)
|
||||
# define K10 PAL_LINE(GPIOK, 10)
|
||||
# define K11 PAL_LINE(GPIOK, 11)
|
||||
# define K12 PAL_LINE(GPIOK, 12)
|
||||
# define K13 PAL_LINE(GPIOK, 13)
|
||||
# define K14 PAL_LINE(GPIOK, 14)
|
||||
# define K15 PAL_LINE(GPIOK, 15)
|
||||
# endif
|
||||
#endif
|
|
@ -0,0 +1,22 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include "pin_defs.h"
|
||||
|
||||
#if __has_include_next("gpio.h")
|
||||
# include_next "gpio.h" /* Include the platforms gpio.h */
|
||||
#endif
|
|
@ -0,0 +1,23 @@
|
|||
/* Copyright 2021 QMK
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 3 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
// useful for direct pin mapping
|
||||
#define NO_PIN (pin_t)(~0)
|
||||
|
||||
#if __has_include_next("pin_defs.h")
|
||||
# include_next "pin_defs.h" /* Include the platforms pin_defs.h */
|
||||
#endif
|
|
@ -202,7 +202,7 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
/* Windows Terminal Layer
|
||||
*
|
||||
* ,-----------------------------------. ,-----------------------------------.
|
||||
* | | | | | | | | | | | | |BkSpc|
|
||||
* | |Tab 1|Tab 2|Tab 3|Tab 4|Tab 5| |Tab 6|Tab 7|Tab 8|Tab 9| |BkSpc|
|
||||
* |-----------------------------------| |-----------------------------------|
|
||||
* | | |Split|Close| | | |FcsLf|FcsDn|FcsUp|FcsRt| | |
|
||||
* |-----------------------------------| |-----------------------------------|
|
||||
|
@ -212,13 +212,13 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
* `-----------------------------------' `-----------------------------------'
|
||||
*/
|
||||
|
||||
#define ________________WINDOWS_TERMINAL_L1________________ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX
|
||||
#define ________________WINDOWS_TERMINAL_L2________________ _______, XXXXXXX, MC_trps, MC_trpc, XXXXXXX, XXXXXXX
|
||||
#define ________________WINDOWS_TERMINAL_L1________________ XXXXXXX, MC_trt1, MC_trt2, MC_trt3, MC_trt4, MC_trt5
|
||||
#define ________________WINDOWS_TERMINAL_L2________________ _______, XXXXXXX, MC_trps, MC_trpc, XXXXXXX, MC_trtn
|
||||
#define ________________WINDOWS_TERMINAL_L3________________ _______, XXXXXXX, XXXXXXX, XXXXXXX, MC_trpv, XXXXXXX
|
||||
#define ________________WINDOWS_TERMINAL_L4________________ _______, _______, _______, _______, _______, _______
|
||||
|
||||
#define ________________WINDOWS_TERMINAL_R1________________ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_BSPC
|
||||
#define ________________WINDOWS_TERMINAL_R2________________ MC_trpl, MC_trpd, MC_trpu, MC_trpr, XXXXXXX, XXXXXXX
|
||||
#define ________________WINDOWS_TERMINAL_R1________________ MC_trt6, MC_trt7, MC_trt8, MC_trt9, XXXXXXX, KC_BSPC
|
||||
#define ________________WINDOWS_TERMINAL_R2________________ MC_trpl, MC_trpd, MC_trpu, MC_trpr, MC_trcp, XXXXXXX
|
||||
#define ________________WINDOWS_TERMINAL_R3________________ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, _______
|
||||
#define ________________WINDOWS_TERMINAL_R4________________ _______, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX
|
||||
|
||||
|
@ -297,6 +297,7 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
#define CUSTOM_MACROS(CUSTOM_NAME, CUSTOM_STRING, CUSTOM_DELIM) \
|
||||
CUSTOM_NAME(rdcc) CUSTOM_STRING(SS_LCTL(SS_LALT(SS_TAP(X_HOME)))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(lcad) CUSTOM_STRING(SS_LCTL(SS_LALT(SS_TAP(X_DELETE)))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trcp) CUSTOM_STRING(SS_LCTL(SS_LSFT("p"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trps) CUSTOM_STRING(SS_LALT(SS_LSFT("-"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trpv) CUSTOM_STRING(SS_LALT(SS_LSFT("+"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trpc) CUSTOM_STRING(SS_LCTL(SS_LSFT("w"))) CUSTOM_DELIM() \
|
||||
|
@ -304,6 +305,16 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
CUSTOM_NAME(trpd) CUSTOM_STRING(SS_LALT(SS_TAP(X_DOWN))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trpu) CUSTOM_STRING(SS_LALT(SS_TAP(X_UP))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trpr) CUSTOM_STRING(SS_LALT(SS_TAP(X_RIGHT))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trtn) CUSTOM_STRING(SS_LCTL(SS_LSFT("t"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt1) CUSTOM_STRING(SS_LCTL(SS_LALT("1"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt2) CUSTOM_STRING(SS_LCTL(SS_LALT("2"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt3) CUSTOM_STRING(SS_LCTL(SS_LALT("3"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt4) CUSTOM_STRING(SS_LCTL(SS_LALT("4"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt5) CUSTOM_STRING(SS_LCTL(SS_LALT("5"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt6) CUSTOM_STRING(SS_LCTL(SS_LALT("6"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt7) CUSTOM_STRING(SS_LCTL(SS_LALT("7"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt8) CUSTOM_STRING(SS_LCTL(SS_LALT("8"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(trt9) CUSTOM_STRING(SS_LCTL(SS_LALT("9"))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(vtdl) CUSTOM_STRING(SS_LCTL(SS_LGUI(SS_TAP(X_LEFT)))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(vtdc) CUSTOM_STRING(SS_LCTL(SS_LGUI(SS_TAP(X_F4)))) CUSTOM_DELIM() \
|
||||
CUSTOM_NAME(vtdn) CUSTOM_STRING(SS_LCTL(SS_LGUI("d"))) CUSTOM_DELIM() \
|
||||
|
|
Loading…
Reference in New Issue