[Keyboard] Fallacy (#9499)
* Add Fallacy * Accept suggestions * fixed build error, renamed readme, added keymap specific config to force enable NKRO * remove FORCE_NKRO on VIA keymap, fix header * fix info.json to accurately reflect keymap * remove additional empty layers from default_split_bs keymap * Accept keymap formatting suggestions * remove empty config files at keymap levelmaster
parent
4f9e5d4cde
commit
09a53d1aa3
|
@ -0,0 +1,69 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include "config_common.h"
|
||||
|
||||
/* USB Device descriptor parameter
|
||||
*/
|
||||
#define VENDOR_ID 0xBF00
|
||||
#define PRODUCT_ID 0xBFFA
|
||||
#define DEVICE_VER 0x0001
|
||||
#define MANUFACTURER SheuBox
|
||||
#define PRODUCT Fallacy
|
||||
#define DESCRIPTION Aluminum Alice Clone
|
||||
|
||||
/* key matrix size
|
||||
*/
|
||||
#define MATRIX_ROWS 5
|
||||
#define MATRIX_COLS 15
|
||||
|
||||
/* key matrix pins
|
||||
*/
|
||||
#define MATRIX_ROW_PINS { B1, B2, B3, C6, C7 }
|
||||
#define MATRIX_COL_PINS { E6, F0, F1, F4, F5, F6, F7, B6, B5, B4, D7, D6, D4, D5, D3 }
|
||||
#define UNUSED_PINS { B0, B7 }
|
||||
|
||||
/* COL2ROW or ROW2COL
|
||||
*/
|
||||
#define DIODE_DIRECTION COL2ROW
|
||||
|
||||
/* IS31FL3731 driver address (for status LEDs)
|
||||
* Using the default defines here, but using a custom implementation
|
||||
*/
|
||||
#define LED_DRIVER_ADDR_1 0b1110100
|
||||
#define LED_DRIVER_COUNT 1
|
||||
#define LED_DRIVER_LED_COUNT 3
|
||||
|
||||
/* Set 0 if debouncing isn't needed
|
||||
*/
|
||||
#define DEBOUNCE 5
|
||||
|
||||
/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap
|
||||
*/
|
||||
#define LOCKING_SUPPORT_ENABLE
|
||||
|
||||
/* Locking resynchronize hack
|
||||
*/
|
||||
#define LOCKING_RESYNC_ENABLE
|
||||
|
||||
/* prevent stuck modifiers
|
||||
*/
|
||||
#define PREVENT_STUCK_MODIFIERS
|
||||
|
||||
#define RGB_DI_PIN D2
|
||||
#define RGBLIGHT_ANIMATIONS
|
||||
#define RGBLED_NUM 14
|
|
@ -0,0 +1,40 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include "fallacy.h"
|
||||
#include "indicators.h"
|
||||
|
||||
void matrix_init_kb(void) {
|
||||
init_fallacy_leds();
|
||||
matrix_init_user();
|
||||
}
|
||||
|
||||
void matrix_scan_kb(void) {
|
||||
update_fallacy_leds();
|
||||
matrix_scan_user();
|
||||
}
|
||||
|
||||
/* update LED driver with usb led_state
|
||||
*/
|
||||
bool led_update_kb(led_t led_state) {
|
||||
bool res = led_update_user(led_state);
|
||||
if(res) {
|
||||
set_fallacy_led(2, led_state.caps_lock); /* caps */
|
||||
set_fallacy_led(1, led_state.num_lock); /* num lock */
|
||||
set_fallacy_led(0, led_state.scroll_lock); /* scroll lock */
|
||||
}
|
||||
return res;
|
||||
}
|
|
@ -0,0 +1,54 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include "quantum.h"
|
||||
|
||||
/* All keys in matrix active:
|
||||
* - Split Backspace
|
||||
* - Split Right Shift
|
||||
*/
|
||||
#define LAYOUT_all( \
|
||||
K100, K000, K001, K002, K003, K004, K005, K006, K007, K008, K009, K010, K011, K012, K013, K014, \
|
||||
K200, K101, K102, K103, K104, K105, K106, K107, K108, K109, K110, K111, K112, K113, K114, \
|
||||
K300, K201, K202, K203, K204, K205, K206, K207, K208, K209, K210, K211, K212, K214, \
|
||||
K301, K302, K303, K304, K305, K306, K307, K308, K309, K310, K311, K312, K313, K314, \
|
||||
K401, K403, K405, K406, K408, K410, K414 \
|
||||
) { \
|
||||
{ K000, K001, K002, K003, K004, K005, K006, K007, K008, K009, K010, K011, K012, K013, K014 }, \
|
||||
{ K100, K101, K102, K103, K104, K105, K106, K107, K108, K109, K110, K111, K112, K113, K114 }, \
|
||||
{ K200, K201, K202, K203, K204, K205, K206, K207, K208, K209, K210, K211, K212, KC_NO, K214 }, \
|
||||
{ K300, K301, K302, K303, K304, K305, K306, K307, K308, K309, K310, K311, K312, K313, K314 }, \
|
||||
{ KC_NO, K401, KC_NO, K403, KC_NO, K405, K406, KC_NO, K408, KC_NO, K410, KC_NO, KC_NO, KC_NO, K414 } \
|
||||
}
|
||||
|
||||
/* Disable position 013 and 314 for:
|
||||
* - Full size Backspace
|
||||
* - Full size Right Shift
|
||||
*/
|
||||
#define LAYOUT_default( \
|
||||
K100, K000, K001, K002, K003, K004, K005, K006, K007, K008, K009, K010, K011, K012, K014, \
|
||||
K200, K101, K102, K103, K104, K105, K106, K107, K108, K109, K110, K111, K112, K113, K114, \
|
||||
K300, K201, K202, K203, K204, K205, K206, K207, K208, K209, K210, K211, K212, K214, \
|
||||
K301, K302, K303, K304, K305, K306, K307, K308, K309, K310, K311, K312, K313, K314, \
|
||||
K401, K403, K405, K406, K408, K410, K414 \
|
||||
) { \
|
||||
{ K000, K001, K002, K003, K004, K005, K006, K007, K008, K009, K010, K011, K012, KC_NO, K014 }, \
|
||||
{ K100, K101, K102, K103, K104, K105, K106, K107, K108, K109, K110, K111, K112, K113, K114 }, \
|
||||
{ K200, K201, K202, K203, K204, K205, K206, K207, K208, K209, K210, K211, K212, KC_NO, K214 }, \
|
||||
{ K300, K301, K302, K303, K304, K305, K306, K307, K308, K309, K310, K311, K312, K313, K314 }, \
|
||||
{ KC_NO, K401, KC_NO, K403, KC_NO, K405, K406, KC_NO, K408, KC_NO, K410, KC_NO, KC_NO, KC_NO, K414 } \
|
||||
}
|
|
@ -0,0 +1,61 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
|
||||
#include "indicators.h"
|
||||
#include "drivers/issi/is31fl3731-simple.h"
|
||||
#include "i2c_master.h"
|
||||
|
||||
/* Set up IS31FL3731 for use in powering indicator LEDs. Absolutely overkill for this job but it was already in the design.
|
||||
* init IS31FL3731 and i2c
|
||||
*/
|
||||
void init_fallacy_leds(void) {
|
||||
i2c_init();
|
||||
IS31FL3731_init(LED_DRIVER_ADDR_1);
|
||||
|
||||
for (int i = 0; i < LED_DRIVER_LED_COUNT; i++) {
|
||||
IS31FL3731_set_led_control_register(i, true);
|
||||
}
|
||||
|
||||
IS31FL3731_update_led_control_registers(LED_DRIVER_ADDR_1, 0);
|
||||
}
|
||||
|
||||
|
||||
/* update the buffer
|
||||
*/
|
||||
void update_fallacy_leds(void) {
|
||||
IS31FL3731_update_pwm_buffers(LED_DRIVER_ADDR_1, 0);
|
||||
}
|
||||
|
||||
|
||||
/* wrapper to actually set the LED PWM
|
||||
*/
|
||||
void set_fallacy_led(int index, bool state) {
|
||||
if (state) {
|
||||
IS31FL3731_set_value(index, 128);
|
||||
}
|
||||
else {
|
||||
IS31FL3731_set_value(index, 0);
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
/* define LED matrix
|
||||
*/
|
||||
const is31_led g_is31_leds[LED_DRIVER_LED_COUNT] = {
|
||||
{0, C1_1},
|
||||
{0, C2_1},
|
||||
{0, C3_1},
|
||||
};
|
|
@ -0,0 +1,23 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
#include "quantum.h"
|
||||
|
||||
|
||||
void init_fallacy_leds(void);
|
||||
void update_fallacy_leds(void);
|
||||
void set_fallacy_led(int index, bool state);
|
|
@ -0,0 +1,147 @@
|
|||
{
|
||||
"keyboard_name": "Fallacy",
|
||||
"maintainer": "Toraifu",
|
||||
"width": 18.75,
|
||||
"height": 5,
|
||||
"layouts": {
|
||||
"LAYOUT_all": {
|
||||
"layout": [
|
||||
{"label":"Esc", "x":0.5, "y":0},
|
||||
{"label":"~", "x":1.75, "y":0},
|
||||
{"label":"!", "x":2.75, "y":0},
|
||||
{"label":"@", "x":3.75, "y":0},
|
||||
{"label":"#", "x":4.75, "y":0},
|
||||
{"label":"$", "x":5.75, "y":0},
|
||||
{"label":"%", "x":6.75, "y":0},
|
||||
{"label":"^", "x":7.75, "y":0},
|
||||
{"label":"&", "x":10, "y":0},
|
||||
{"label":"*", "x":11, "y":0},
|
||||
{"label":"(", "x":12, "y":0},
|
||||
{"label":")", "x":13, "y":0},
|
||||
{"label":"_", "x":14, "y":0},
|
||||
{"label":"+", "x":15, "y":0},
|
||||
{"label":"|", "x":16, "y":0},
|
||||
{"label":"~", "x":17, "y":0},
|
||||
{"label":"PgUp", "x":0.25, "y":1},
|
||||
{"label":"Tab", "x":1.5, "y":1, "w":1.5},
|
||||
{"label":"Q", "x":3, "y":1},
|
||||
{"label":"W", "x":4, "y":1},
|
||||
{"label":"E", "x":5, "y":1},
|
||||
{"label":"R", "x":6, "y":1},
|
||||
{"label":"T", "x":7, "y":1},
|
||||
{"label":"Y", "x":9.75, "y":1},
|
||||
{"label":"U", "x":10.75, "y":1},
|
||||
{"label":"I", "x":11.75, "y":1},
|
||||
{"label":"O", "x":12.75, "y":1},
|
||||
{"label":"P", "x":13.75, "y":1},
|
||||
{"label":"{", "x":14.75, "y":1},
|
||||
{"label":"}", "x":15.75, "y":1},
|
||||
{"label":"Backspace", "x":16.75, "y":1, "w":1.5},
|
||||
{"label":"PgDn", "x":0, "y":2},
|
||||
{"label":"Control", "x":1.25, "y":2, "w":1.75},
|
||||
{"label":"A", "x":3, "y":2},
|
||||
{"label":"S", "x":4, "y":2},
|
||||
{"label":"D", "x":5, "y":2},
|
||||
{"label":"F", "x":6, "y":2},
|
||||
{"label":"G", "x":7, "y":2},
|
||||
{"label":"H", "x":10.25, "y":2},
|
||||
{"label":"J", "x":11.25, "y":2},
|
||||
{"label":"K", "x":12.25, "y":2},
|
||||
{"label":"L", "x":13.25, "y":2},
|
||||
{"label":":", "x":14.25, "y":2},
|
||||
{"label":"\"", "x":15.25, "y":2},
|
||||
{"label":"Enter", "x":16.25, "y":2, "w":2.25},
|
||||
{"label":"Shift", "x":1, "y":3, "w":2.25},
|
||||
{"label":"Z", "x":3.25, "y":3},
|
||||
{"label":"X", "x":4.25, "y":3},
|
||||
{"label":"C", "x":5.25, "y":3},
|
||||
{"label":"V", "x":6.25, "y":3},
|
||||
{"label":"B", "x":7.25, "y":3},
|
||||
{"label":"B", "x":10, "y":3},
|
||||
{"label":"N", "x":11, "y":3},
|
||||
{"label":"M", "x":12, "y":3},
|
||||
{"label":"<", "x":13, "y":3},
|
||||
{"label":">", "x":14, "y":3},
|
||||
{"label":"?", "x":15, "y":3},
|
||||
{"label":"Shift", "x":16, "y":3, "w":1.75},
|
||||
{"label":"Fn1", "x":17.75, "y":3},
|
||||
{"label":"Ctrl", "x":1, "y":4, "w":1.5},
|
||||
{"label":"Alt", "x":4, "y":4, "w":1.5},
|
||||
{"label":"LSpace", "x":5.5, "y":4, "w":2.25},
|
||||
{"label":"Fn2", "x":7.75, "y":4},
|
||||
{"label":"RSpace", "x":10, "y":4, "w":2.75},
|
||||
{"label":"RAlt", "x":12.75, "y":4, "w":1.5},
|
||||
{"label":"RCtl", "x":17, "y":4, "w":1.5}
|
||||
]
|
||||
},
|
||||
"LAYOUT_default": {
|
||||
"layout": [
|
||||
{"label":"Esc", "x":0.5, "y":0},
|
||||
{"label":"~", "x":1.75, "y":0},
|
||||
{"label":"!", "x":2.75, "y":0},
|
||||
{"label":"@", "x":3.75, "y":0},
|
||||
{"label":"#", "x":4.75, "y":0},
|
||||
{"label":"$", "x":5.75, "y":0},
|
||||
{"label":"%", "x":6.75, "y":0},
|
||||
{"label":"^", "x":7.75, "y":0},
|
||||
{"label":"&", "x":10, "y":0},
|
||||
{"label":"*", "x":11, "y":0},
|
||||
{"label":"(", "x":12, "y":0},
|
||||
{"label":")", "x":13, "y":0},
|
||||
{"label":"_", "x":14, "y":0},
|
||||
{"label":"+", "x":15, "y":0},
|
||||
{"label":"Backspace", "x":16, "y":0, "w":2},
|
||||
{"label":"PgUp", "x":0.25, "y":1},
|
||||
{"label":"Tab", "x":1.5, "y":1, "w":1.5},
|
||||
{"label":"Q", "x":3, "y":1},
|
||||
{"label":"W", "x":4, "y":1},
|
||||
{"label":"E", "x":5, "y":1},
|
||||
{"label":"R", "x":6, "y":1},
|
||||
{"label":"T", "x":7, "y":1},
|
||||
{"label":"Y", "x":9.75, "y":1},
|
||||
{"label":"U", "x":10.75, "y":1},
|
||||
{"label":"I", "x":11.75, "y":1},
|
||||
{"label":"O", "x":12.75, "y":1},
|
||||
{"label":"P", "x":13.75, "y":1},
|
||||
{"label":"{", "x":14.75, "y":1},
|
||||
{"label":"}", "x":15.75, "y":1},
|
||||
{"label":"Backspace", "x":16.75, "y":1, "w":1.5},
|
||||
{"label":"PgDn", "x":0, "y":2},
|
||||
{"label":"Control", "x":1.25, "y":2, "w":1.75},
|
||||
{"label":"A", "x":3, "y":2},
|
||||
{"label":"S", "x":4, "y":2},
|
||||
{"label":"D", "x":5, "y":2},
|
||||
{"label":"F", "x":6, "y":2},
|
||||
{"label":"G", "x":7, "y":2},
|
||||
{"label":"H", "x":10.25, "y":2},
|
||||
{"label":"J", "x":11.25, "y":2},
|
||||
{"label":"K", "x":12.25, "y":2},
|
||||
{"label":"L", "x":13.25, "y":2},
|
||||
{"label":":", "x":14.25, "y":2},
|
||||
{"label":"\"", "x":15.25, "y":2},
|
||||
{"label":"Enter", "x":16.25, "y":2, "w":2.25},
|
||||
{"label":"Shift", "x":1, "y":3, "w":2.25},
|
||||
{"label":"Z", "x":3.25, "y":3},
|
||||
{"label":"X", "x":4.25, "y":3},
|
||||
{"label":"C", "x":5.25, "y":3},
|
||||
{"label":"V", "x":6.25, "y":3},
|
||||
{"label":"B", "x":7.25, "y":3},
|
||||
{"label":"B", "x":10, "y":3},
|
||||
{"label":"N", "x":11, "y":3},
|
||||
{"label":"M", "x":12, "y":3},
|
||||
{"label":"<", "x":13, "y":3},
|
||||
{"label":">", "x":14, "y":3},
|
||||
{"label":"?", "x":15, "y":3},
|
||||
{"label":"Shift", "x":16, "y":3, "w":1.75},
|
||||
{"label":"Fn1", "x":17.75, "y":3},
|
||||
{"label":"Ctrl", "x":1, "y":4, "w":1.5},
|
||||
{"label":"Alt", "x":4, "y":4, "w":1.5},
|
||||
{"label":"LSpace", "x":5.5, "y":4, "w":2.25},
|
||||
{"label":"Fn2", "x":7.75, "y":4},
|
||||
{"label":"RSpace", "x":10, "y":4, "w":2.75},
|
||||
{"label":"RAlt", "x":12.75, "y":4, "w":1.5},
|
||||
{"label":"RCtl", "x":17, "y":4, "w":1.5}
|
||||
]
|
||||
}
|
||||
}
|
||||
}
|
|
@ -0,0 +1,34 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
|
||||
[0] = LAYOUT_default(
|
||||
KC_ESC, KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC,
|
||||
KC_PGUP, KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS,
|
||||
KC_PGDN, KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_RGUI, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, MO(1),
|
||||
KC_LCTL, KC_LALT, KC_SPC, KC_LGUI, KC_SPC, KC_RALT, KC_RCTL),
|
||||
|
||||
[1] = LAYOUT_default(
|
||||
KC_TRNS, RESET, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F11, KC_F12, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS),
|
||||
|
||||
};
|
|
@ -0,0 +1,33 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
|
||||
[0] = LAYOUT_all(
|
||||
KC_ESC, KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSLS, KC_BSPC,
|
||||
KC_PGUP, KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS,
|
||||
KC_PGDN, KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_RGUI, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, MO(1),
|
||||
KC_LCTL, KC_LALT, KC_SPC, KC_LGUI, KC_SPC, KC_RALT, KC_RCTL),
|
||||
|
||||
[1] = LAYOUT_all(
|
||||
KC_TRNS, RESET, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS),
|
||||
};
|
|
@ -0,0 +1,47 @@
|
|||
/* Copyright 2020 B. Fletcher (toraifu) <typefast@kyaa.gg>
|
||||
*
|
||||
* This program is free software: you can redistribute it and/or modify
|
||||
* it under the terms of the GNU General Public License as published by
|
||||
* the Free Software Foundation, either version 2 of the License, or
|
||||
* (at your option) any later version.
|
||||
*
|
||||
* This program is distributed in the hope that it will be useful,
|
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
* GNU General Public License for more details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License
|
||||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
#include QMK_KEYBOARD_H
|
||||
|
||||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
|
||||
[0] = LAYOUT_all(
|
||||
KC_ESC, KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSLS, KC_BSPC,
|
||||
KC_PGUP, KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS,
|
||||
KC_PGDN, KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
|
||||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_RGUI, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, MO(1),
|
||||
KC_LCTL, KC_LALT, KC_SPC, KC_LGUI, KC_SPC, KC_RALT, KC_RCTL),
|
||||
|
||||
[1] = LAYOUT_all(
|
||||
KC_TRNS, RESET, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS),
|
||||
|
||||
[2] = LAYOUT_all(
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS),
|
||||
|
||||
[3] = LAYOUT_all(
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
|
||||
KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS),
|
||||
};
|
|
@ -0,0 +1 @@
|
|||
VIA_ENABLE = yes
|
|
@ -0,0 +1,21 @@
|
|||
# Fallacy
|
||||
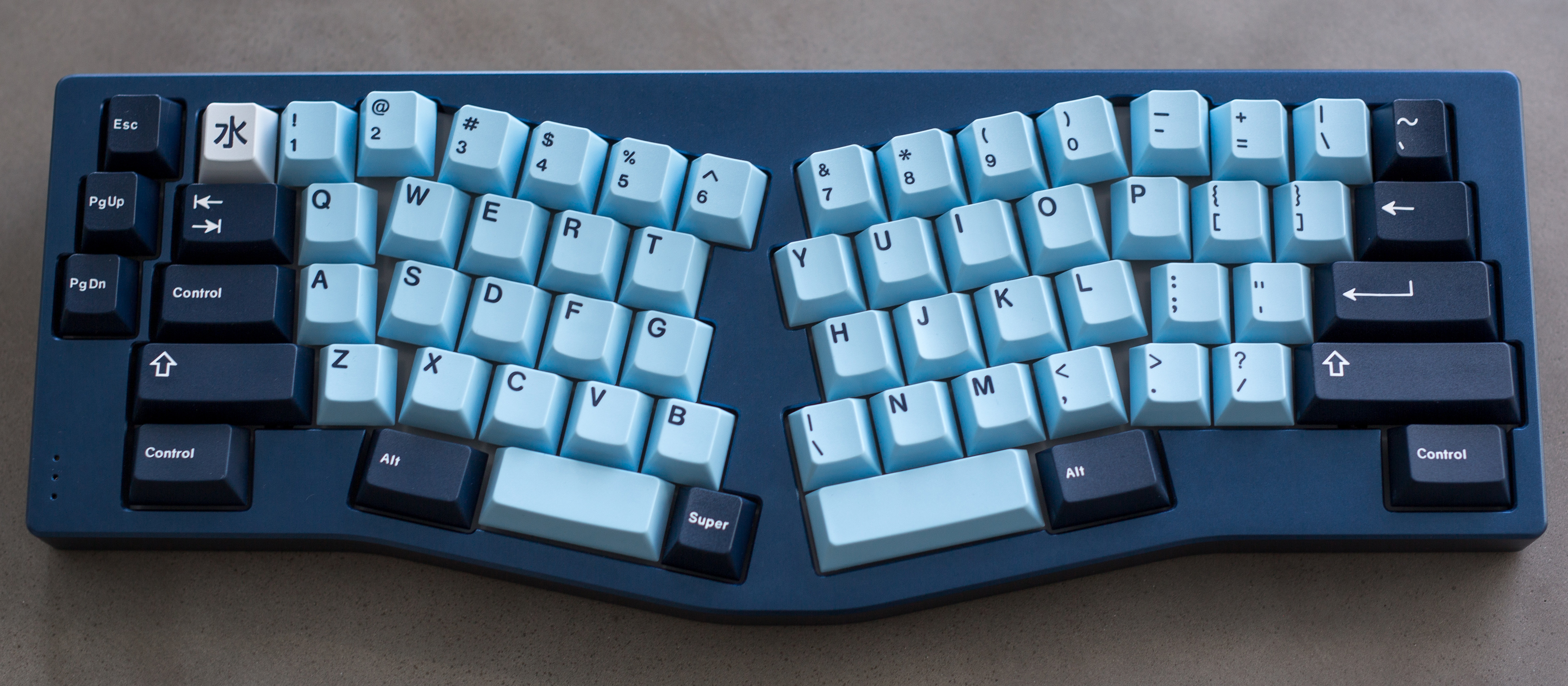
|
||||
|
||||
A PCB designed for the Fallacy custom keyboard, but fully compatible with the Alice and its clones.
|
||||
|
||||
* Keyboard Maintainer: [toraifu](https://github.com/toraifu)
|
||||
* Hardware: [geekhack](https://geekhack.org/index.php?topic=103728.0)
|
||||
* Other general info: [fallacy](https://github.com/toraifu/fallacy)
|
||||
|
||||
### Features:
|
||||
* VIA support
|
||||
* As minimal as possible design (no in-switch LEDs)
|
||||
* Support for the use of fourteen WS2812B RGB-LEDs for keyboards for which underglow is desired.
|
||||
* Misc features such as ESD protection, indicator LEDs, etc.
|
||||
|
||||
|
||||
Make example for this keyboard (after setting up your build environment):
|
||||
|
||||
make fallacy:default
|
||||
|
||||
See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
|
|
@ -0,0 +1,29 @@
|
|||
# MCU name
|
||||
MCU = atmega32u4
|
||||
|
||||
# Bootloader selection
|
||||
BOOTLOADER = atmel-dfu
|
||||
|
||||
# Build Options
|
||||
# change yes to no to disable
|
||||
#
|
||||
BOOTMAGIC_ENABLE = lite # Virtual DIP switch configuration
|
||||
MOUSEKEY_ENABLE = no # Mouse keys
|
||||
EXTRAKEY_ENABLE = yes # Audio control and System control
|
||||
CONSOLE_ENABLE = no # Console for debug
|
||||
COMMAND_ENABLE = yes # Commands for debug and configuration
|
||||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
|
||||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
|
||||
# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
|
||||
NKRO_ENABLE = yes # USB Nkey Rollover
|
||||
BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality
|
||||
RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow
|
||||
MIDI_ENABLE = no # MIDI support
|
||||
BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID
|
||||
AUDIO_ENABLE = no # Audio output
|
||||
FAUXCLICKY_ENABLE = no # Use buzzer to emulate clicky switches
|
||||
|
||||
# project specific files
|
||||
SRC += indicators.c \
|
||||
drivers/issi/is31fl3731-simple.c
|
||||
QUANTUM_LIB_SRC += i2c_master.c
|
Loading…
Reference in New Issue