diff --git a/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/config.h b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/config.h
new file mode 100644
index 000000000..9d842a791
--- /dev/null
+++ b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/config.h
@@ -0,0 +1,36 @@
+/* Copyright 2021 Jonavin Eng
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#pragma once
+
+#define TAPPING_TOGGLE 2
+// TT set to two taps
+
+/* Handle GRAVESC combo keys */
+#define GRAVE_ESC_ALT_OVERRIDE
+ //Always send Escape if Alt is pressed
+#define GRAVE_ESC_CTRL_OVERRIDE
+ //Always send Escape if Control is pressed
+
+#define TAPPING_TERM 180
+
+#ifdef RGB_MATRIX_ENABLE
+ #ifdef RGB_MATRIX_STARTUP_MODE
+ #undef RGB_MATRIX_STARTUP_MODE
+ #endif
+ #define RGB_MATRIX_STARTUP_MODE RGB_MATRIX_SOLID_COLOR
+ #define RGB_DISABLE_WHEN_USB_SUSPENDED
+#endif
diff --git a/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/keymap.c b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/keymap.c
new file mode 100644
index 000000000..1b85354be
--- /dev/null
+++ b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/keymap.c
@@ -0,0 +1,137 @@
+/* Copyright 2021 Jonavin
+
+This program is free software: you can redistribute it and/or modify
+it under the terms of the GNU General Public License as published by
+the Free Software Foundation, either version 2 of the License, or
+(at your option) any later version.
+
+This program is distributed in the hope that it will be useful,
+but WITHOUT ANY WARRANTY; without even the implied warranty of
+MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+GNU General Public License for more details.
+
+You should have received a copy of the GNU General Public License
+along with this program. If not, see .
+*/
+
+#include QMK_KEYBOARD_H
+#include "rgb_matrix_map.h"
+#include "jonavin.h"
+
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ [_BASE] = LAYOUT_65_ansi_blocker(
+ KC_GESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_DEL,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLASH, KC_PGUP,
+ TT(_LOWER), KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_PGDN,
+ KC_LSFTCAPSWIN, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, KC_END,
+ KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, MO(1), KC_RCTL, KC_LEFT, KC_DOWN, KC_RIGHT
+ ),
+ [_FN1] = LAYOUT_65_ansi_blocker(
+ KC_GESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_DEL, KC_CALC,
+ _______, RGB_TOG, RGB_MOD, RGB_HUI, RGB_HUD, RGB_SAI, RGB_SAD, KC_PSCR, KC_SLCK, KC_PAUS, _______, _______, _______, RESET, KC_HOME,
+ KC_CAPS, RGB_SPI, RGB_SPD, RGB_VAD, RGB_VAI, _______, _______, _______, _______, _______, _______, _______, EEP_RST, KC_END,
+ KC_LSFT, RGB_NITE, _______, _______, _______, _______, KC_NLCK, _______, RGB_TOD, RGB_TOI, KC_MPLY, _______, KC_VOLU, KC_MUTE,
+ _______, KC_WINLCK, _______, _______, _______, _______, KC_MPRV, KC_VOLD, KC_MNXT
+ ),
+ [_LOWER] = LAYOUT_65_ansi_blocker(
+ KC_TILD, _______, _______, _______, _______, _______, _______, KC_P7, KC_P8, KC_P9, KC_P0, KC_PMNS, KC_PPLS, _______, _______,
+ _______, KC_HOME, KC_UP, KC_END, KC_PGUP, _______, KC_TAB, KC_P4, KC_P5, KC_P6, KC_PDOT, _______, _______, _______, KC_HOME,
+ _______, KC_LEFT, KC_DOWN, KC_RGHT, KC_PGDN, _______, _______, KC_P1, KC_P2, KC_P3, KC_NO, KC_PAST, KC_PENT, KC_END,
+ _______, KC_NO, KC_DEL, KC_INS, KC_NO, KC_NO, KC_NO, KC_P0, KC_00, KC_PDOT, KC_PSLS, _______, _______, _______,
+ _______, _______, _______, KC_BSPC, _______, _______, _______, _______, _______
+ ),
+ [_RAISE] = LAYOUT_65_ansi_blocker(
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, KC_SPC, _______, _______, _______, _______, _______
+ ),
+};
+
+#ifdef RGB_MATRIX_ENABLE
+ // Capslock, Scroll lock and Numlock indicator
+ void rgb_matrix_indicators_advanced_user(uint8_t led_min, uint8_t led_max) {
+ if (get_rgb_nightmode()) rgb_matrix_set_color_all(RGB_OFF);
+ if (IS_HOST_LED_ON(USB_LED_SCROLL_LOCK)) {
+ rgb_matrix_set_color(LED_I, RGB_GREEN);
+ }
+
+ #ifdef INVERT_NUMLOCK_INDICATOR
+ if (!IS_HOST_LED_ON(USB_LED_NUM_LOCK)) { // on if NUM lock is OFF
+ rgb_matrix_set_color(LED_B, RGB_GREEN);
+ rgb_matrix_set_color(LED_N, RGB_GREEN);
+ rgb_matrix_set_color(LED_M, RGB_GREEN);
+ }
+ #else
+ if (IS_HOST_LED_ON(USB_LED_NUM_LOCK)) { // Normal, on if NUM lock is ON
+ rgb_matrix_set_color(LED_B, RGB_GREEN);
+ rgb_matrix_set_color(LED_N, RGB_GREEN);
+ rgb_matrix_set_color(LED_M, RGB_GREEN);
+ }
+ #endif // INVERT_NUMLOCK_INDICATOR
+
+ if (IS_HOST_LED_ON(USB_LED_CAPS_LOCK)) {
+ rgb_matrix_set_color(LED_CAPS, RGB_RED);
+ rgb_matrix_set_color(LED_Q, RGB_RED);
+ rgb_matrix_set_color(LED_A, RGB_RED);
+ }
+ if (keymap_config.no_gui) {
+ rgb_matrix_set_color(LED_LCTL, RGB_RED); //light up Win key when disabled
+ rgb_matrix_set_color(LED_LWIN, RGB_RED); //light up Win key when disabled
+ rgb_matrix_set_color(LED_LALT, RGB_RED); //light up Win key when disabled
+ }
+ switch(get_highest_layer(layer_state)){ // special handling per layer
+ case _FN1: // on Fn layer select what the encoder does when pressed
+ rgb_matrix_set_color(LED_FN, RGB_GOLD);
+ rgb_matrix_set_color(LED_RCTL, RGB_GOLD);
+
+ #ifdef IDLE_TIMEOUT_ENABLE
+ // Add RGB Timeout Indicator -- shows 0 to 139 using num row and qwerty row
+ uint16_t timeout_threshold = get_timeout_threshold();
+ if (timeout_threshold <= 10) rgb_matrix_set_color(LED_LIST_NUMROW[timeout_threshold], RGB_GOLD);
+ else if (timeout_threshold < 140) {
+ rgb_matrix_set_color(LED_LIST_NUMROW[(timeout_threshold % 10)], RGB_GOLD);
+ rgb_matrix_set_color(LED_LIST_QWERTYROW[(timeout_threshold / 10)], RGB_GOLD);
+ } else { // >= 140 minutes, just show these 3 lights
+ rgb_matrix_set_color(LED_LIST_NUMROW[10], RGB_GOLD);
+ rgb_matrix_set_color(LED_LIST_NUMROW[11], RGB_GOLD);
+ rgb_matrix_set_color(LED_LIST_NUMROW[12], RGB_GOLD);
+ }
+ #endif // IDLE_TIMEOUT_ENABLE
+
+ break;
+ case _LOWER:
+ for (uint8_t i=0; i
+ - setting to zero disables timeout
+ - indicators in FN layer using RGB in number and qwerty rows to show the timeout in minutes
+ - LED address location map as enum definition in rgb_matrix_map.h
+ - LED group lists for arrows, numpad, qwerty row, num row LEDs
+ - default startup in single colour mode
+ - Capslock, Scroll Lock, and Num Lock (not set) indicator
+ - Fn key light up GOLD when Fn layer activate
+ - Win Key light up red when Win Lock mode enabled
+ - Layer 2 activation lights up Numpad area
+ - Fn + Z to turn off all RGB lights except rgb indicators; press again to toggle
+
+rules.mk OPTIONS - Active features from userspace
+STARTUP_NUMLOCK_ON = yes
+ - turns on NUMLOCK by default
+
+TD_LSFT_CAPSLOCK_ENABLE = yes
+ - This will enable double tap on Left Shift to toggle CAPSLOCK when using KC_LSFTCAPS
+
+IDLE_TIMEOUT_ENABLE = yes
+ - Enables Timer functionality; for RGB idle timeouts that can be changed dynamically
+
+INVERT_NUMLOCK_INDICATOR = yes
+ - inverts the Num lock indicator, LED is on when num lock is off
+
+## All layers diagram
+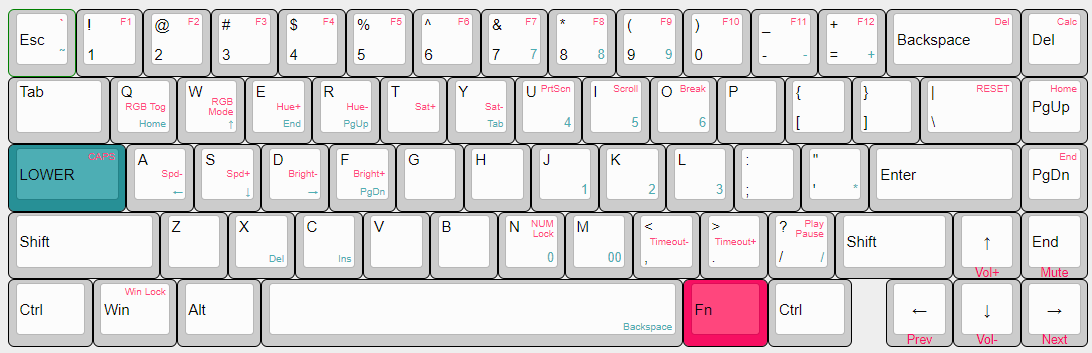
+
diff --git a/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/rgb_matrix_map.h b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/rgb_matrix_map.h
new file mode 100644
index 000000000..66608ba6e
--- /dev/null
+++ b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/rgb_matrix_map.h
@@ -0,0 +1,109 @@
+/* Copyright 2021 Jonavin Eng
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#ifdef RGB_MATRIX_ENABLE
+
+ // Custom RGB Colours
+ #define RGB_GODSPEED 0x00, 0xE4, 0xFF // colour for matching keycaps
+ #define RGB_NAUTILUS 0x00, 0xA4, 0xA9 // Naurilus Font colours
+ #define RGB_PURPLELITE 0x80, 0x00, 0x80 // Purple colours
+
+ // RGB LED locations
+ enum led_location_map {
+ LED_ESC, // 0
+ LED_1, // 1
+ LED_2, // 2
+ LED_3, // 3
+ LED_4, // 4
+ LED_5, // 5
+ LED_6, // 6
+ LED_7, // 7
+ LED_8, // 8
+ LED_9, // 9
+ LED_0, // 10
+ LED_MINS, // 11
+ LED_EQL, // 12
+ LED_BSPC, // 13
+ LED_DEL, // 14
+ LED_TAB, // 15
+ LED_Q, // 16
+ LED_W, // 17
+ LED_E, // 18
+ LED_R, // 19
+ LED_T, // 20
+ LED_Y, // 21
+ LED_U, // 22
+ LED_I, // 23
+ LED_O, // 24
+ LED_P, // 25
+ LED_LBRC, // 26 [
+ LED_RBRC, // 27 ]
+ LED_BSLS, // 28 |
+ LED_PGUP, // 29
+ LED_CAPS, // 30
+ LED_A, // 31
+ LED_S, // 32
+ LED_D, // 33
+ LED_F, // 34
+ LED_G, // 35
+ LED_H, // 36
+ LED_J, // 37
+ LED_K, // 38
+ LED_L, // 39
+ LED_SCLN, // 40 ;
+ LED_QUOT, // 41 "
+ LED_ENT, // 42
+ LED_PGDN, // 43
+ LED_LSFT, // 44
+ LED_Z, // 45
+ LED_X, // 46
+ LED_C, // 47
+ LED_V, // 48
+ LED_B, // 49
+ LED_N, // 50
+ LED_M, // 51
+ LED_COMM, // 52 ,
+ LED_DOT, // 53 .
+ LED_SLSH, // 54 /
+ LED_RSFT, // 55
+ LED_UP, // 56
+ LED_END, // 57
+ LED_LCTL, // 58
+ LED_LWIN, // 59
+ LED_LALT, // 60
+ LED_SPC, // 61
+ LED_FN, // 62
+ LED_RCTL, // 63
+ LED_LEFT, // 64
+ LED_DOWN, // 65
+ LED_RIGHT // 66
+ };
+
+ const uint8_t LED_LIST_WASD[] = { LED_W, LED_A, LED_S, LED_D };
+
+ const uint8_t LED_LIST_ARROWS[] = { LED_LEFT, LED_RIGHT, LED_UP, LED_DOWN };
+
+ const uint8_t LED_LIST_NUMROW[] = { LED_ESC, LED_1, LED_2, LED_3, LED_4, LED_5, LED_6, LED_7, LED_8, LED_9, LED_0, LED_MINS, LED_EQL, LED_BSPC, LED_DEL};
+ const uint8_t LED_LIST_QWERTYROW[] = { LED_TAB, LED_Q, LED_W, LED_E, LED_R, LED_T, LED_Y, LED_U, LED_I, LED_O, LED_P, LED_LBRC, LED_RBRC, LED_BSLS, LED_PGUP};
+
+ const uint8_t LED_LIST_NUMPAD[] = {
+ LED_7, LED_8, LED_9,
+ LED_U, LED_I, LED_O,
+ LED_J, LED_K, LED_L,
+ LED_M, LED_COMM, LED_DOT
+ };
+
+#endif
diff --git a/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/rules.mk b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/rules.mk
new file mode 100644
index 000000000..91153c75a
--- /dev/null
+++ b/keyboards/kbdfans/kbd67/mkiirgb/keymaps/jonavin/rules.mk
@@ -0,0 +1,10 @@
+VIA_ENABLE = yes
+LTO_ENABLE = yes
+MOUSEKEY_ENABLE = no
+TAP_DANCE_ENABLE = yes
+BOOTMAGIC_ENABLE = yes # Enable Bootmagic Lite
+
+TD_LSFT_CAPSLOCK_ENABLE = yes
+IDLE_TIMEOUT_ENABLE = yes
+STARTUP_NUMLOCK_ON = yes
+INVERT_NUMLOCK_INDICATOR = yes
diff --git a/users/jonavin/config.h b/users/jonavin/config.h
index 7b6e335e3..d694bc537 100644
--- a/users/jonavin/config.h
+++ b/users/jonavin/config.h
@@ -26,6 +26,9 @@
#define TAPPING_TERM_PER_KEY
#ifdef RGB_MATRIX_ENABLE
+ #ifdef RGB_MATRIX_STARTUP_MODE
+ #undef RGB_MATRIX_STARTUP_MODE
+ #endif
#define RGB_MATRIX_STARTUP_MODE RGB_MATRIX_SOLID_COLOR
-# define RGB_DISABLE_WHEN_USB_SUSPENDED
+ #define RGB_DISABLE_WHEN_USB_SUSPENDED
#endif
diff --git a/users/jonavin/readme.md b/users/jonavin/readme.md
index 8ec0e0ea1..7712eda1e 100644
--- a/users/jonavin/readme.md
+++ b/users/jonavin/readme.md
@@ -119,6 +119,7 @@ LIST OF COMPATIBLE KEYMAPS
- mechwild/mercutio
- mechwild/murphpad
- mechwild/OBE
+- kbdfans/kdb67
- nopunin10did/kastenwagen (*)
(*) coming soon