diff --git a/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/config.h b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/config.h
new file mode 100644
index 000000000..c6de1b416
--- /dev/null
+++ b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/config.h
@@ -0,0 +1,31 @@
+/* Copyright 2021, 2022 Jonavin Eng
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#pragma once
+
+#define TAPPING_TOGGLE 2
+// TT set to two taps
+
+/* Handle GRAVESC combo keys */
+#define GRAVE_ESC_ALT_OVERRIDE
+ //Always send Escape if Alt is pressed
+#define GRAVE_ESC_CTRL_OVERRIDE
+ //Always send Escape if Control is pressed
+
+#define TAPPING_TERM 180
+
+#define ENCODER_DIRECTION_FLIP // compensate for opposite encoder direction
+#define ENCODER_DEFAULTACTIONS_INDEX 1 // Set default encoder functions to encoder 1
diff --git a/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/keymap.c b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/keymap.c
new file mode 100644
index 000000000..29c12b041
--- /dev/null
+++ b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/keymap.c
@@ -0,0 +1,67 @@
+/* Copyright 2021 W. Alex Ronke, a.k.a. NoPunIn10Did (w.alex.ronke@gmail.com)
+ * Copyright 2022 Jonavin Eng, @Jonavin
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#include QMK_KEYBOARD_H
+#include "jonavin.h"
+
+#define L2_SPC LT(2,KC_SPC)
+#define L3_SPC LT(3,KC_SPC)
+#define RWINALT RALT_T(KC_RGUI)
+#define ISO_LT KC_NUBS
+#define ISO_GT LSFT(KC_NUBS)
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+
+[_BASE] = LAYOUT_48(
+
+ KC_TAB ,KC_Q ,KC_W ,KC_E ,KC_R ,KC_T ,KC_Y ,KC_U ,KC_I ,KC_O ,KC_P ,KC_LBRC,KC_BSPC , KC_DEL ,
+ TT(_RAISE) ,KC_A ,KC_S ,KC_D ,KC_F ,KC_G ,KC_H ,KC_J ,KC_K ,KC_L ,KC_SCLN,KC_QUOT,KC_ENT , KC_PGUP,
+ KC_LSFTCAPS ,KC_Z ,KC_X ,KC_C ,KC_V ,KC_B ,KC_N ,KC_M ,KC_COMM,KC_DOT ,KC_SLSH, KC_SFTENT ,KC_UP , KC_PGDN,
+ KC_LCTL ,KC_LALT ,KC_LGUI ,KC_SPC ,L3_SPC ,MO(_LOWER) ,MO(_FN1) ,KC_RCTL ,KC_LEFT,KC_DOWN,KC_RGHT
+ ),
+[_FN1] = LAYOUT_48(
+ KC_GRV ,KC_F1 ,KC_F2 ,KC_F3 ,KC_F4 ,KC_F5 ,KC_F6 ,KC_F7 ,KC_F8 ,KC_F9 ,KC_F10 ,KC_RBRC,KC_DEL , KC_INS ,
+ KC_CAPS ,KC_F11 ,KC_F12 ,XXXXXXX,XXXXXXX,XXXXXXX,XXXXXXX,KC_PSCR, KC_SLCK, KC_PAUS,XXXXXXX,XXXXXXX,XXXXXXX , KC_HOME,
+ _______ ,XXXXXXX,XXXXXXX,XXXXXXX,XXXXXXX,XXXXXXX,KC_NLCK ,XXXXXXX ,XXXXXXX,XXXXXXX,XXXXXXX,_______ ,KC_PGUP, KC_END ,
+ _______ ,_______ ,KC_WINLCK,XXXXXXX ,XXXXXXX ,_______,_______ ,_______ ,KC_HOME,KC_PGDN,KC_END
+ ),
+[_LOWER] = LAYOUT_48(
+ KC_TILD ,KC_EXLM, KC_AT, KC_HASH,KC_DLR,KC_PERC,KC_CIRC, KC_AMPR, KC_ASTR,KC_LPRN, KC_RPRN,KC_MINS,KC_EQL , RESET,
+ _______ ,KC_MINS, KC_EQL,XXXXXXX,XXXXXXX,XXXXXXX,XXXXXXX,KC_QUES,KC_SLSH,KC_PIPE,KC_BSLS,KC_TILD, XXXXXXX ,XXXXXXX,
+ _______ ,KC_UNDS, KC_PLUS,XXXXXXX,KC_LCBR, KC_RCBR, KC_LBRC, KC_RBRC,KC_LT,KC_GT, XXXXXXX ,_______ ,XXXXXXX,XXXXXXX,
+ _______ ,_______ ,_______,XXXXXXX ,XXXXXXX ,_______,_______ ,_______ ,XXXXXXX,XXXXXXX,XXXXXXX
+ ),
+[_RAISE] = LAYOUT_48(
+ KC_ESC, KC_HOME,KC_UP, KC_END, KC_PGUP,KC_PMNS,KC_PPLS,KC_P7, KC_P8, KC_P9, KC_P0, KC_PMNS, KC_PEQL , KC_TSTOG,
+ TT(_RAISE),KC_LEFT,KC_DOWN,KC_RIGHT,KC_PGDN,KC_PSLS,KC_TAB,KC_P4, KC_P5, KC_P6, KC_PDOT,KC_PAST,KC_PENT , XXXXXXX,
+ _______, XXXXXXX,KC_DEL, KC_INS, KC_NO, KC_PAST,KC_P0, KC_P1, KC_P2, KC_P3, KC_PSLS ,_______, XXXXXXX,XXXXXXX,
+ _______ ,_______ ,_______,KC_BSPC ,XXXXXXX ,_______,_______ ,_______ ,XXXXXXX,XXXXXXX,XXXXXXX
+ )
+};
+
+#ifdef ENCODER_ENABLE // Encoder Functionality
+bool encoder_update_keymap(uint8_t index, bool clockwise) {
+ switch (index) {
+ case 0: // Top left encoder
+ encoder_action_volume(clockwise);
+ break;
+ default:
+ break;
+ }
+ return true; // fall to encoder_update_user and encoder_update_kb definitions
+}
+#endif
diff --git a/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/readme.md b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/readme.md
new file mode 100644
index 000000000..885e6b0dd
--- /dev/null
+++ b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/readme.md
@@ -0,0 +1,54 @@
+Jonavin Kastenwagen 48 Keymap
+Designed to match my other keymaps
+
+NOTE: Uses userpace user/jonavin
+
+Feature changes from Default
+ - Bottom row mapping LCtrl, LAlt, LGui, spacebars, MO(2)/LOWER, MO(1)/FN, RCtrl
+ - CAPS as MO(3)/RAISE - tap to toggle
+ - numpad in MO(3) layer
+ - double tap L Shift for CAPS
+ - RESET moved to under encoder in MO(2)
+ - implement Win Key Lock
+ - GRV Escape overides
+ - ENCODERS
+ - TOP LEFT
+ - Volume up/down
+ - TOP RIGHT
+ - Left Shift held - change layers
+ - Right Shift held - Page Up/Down
+ - Left Ctrl held - navigate next/prev words
+ - Left Alt held - change media next/prev track
+ - RAISE + Encode push toggles between volume change and tab scrolling
+ - ENCODER_DIRECTION_FLIP has been defined to avoid issues with encoder going in opposition direction
+
+OPTIONS in rules.mk
+---------------------------------------
+
+STARTUP_NUMLOCK_ON = yes
+- turns on NUMLOCK by default
+
+ENCODER_DEFAULTACTIONS_ENABLE = yes
+- Enabled default encoder funtions
+- When enabled, use this in the keymap for an additional encoder processing
+- bool encoder_update_keymap(uint8_t index, bool clockwise)
+
+OPTION: set ENCODER_DEFAULTACTIONS_INDEX in config.h to the encoder number if the encoder is not index 0 -- set to 1 for top right encoder
+
+TD_LSFT_CAPSLOCK_ENABLE = yes
+- This will enable double tap on Left Shift to toggle CAPSLOCK
+- KC_LSFTCAPS to bind to left Shift to enable feature
+- KC_LSFTCAPSWIN does the same thing but will not turn on CAPS when Win Lkey is disabled
+
+INVERT_NUMLOCK_INDICATOR
+- inverts the Num lock indicator, LED is on when num lock is off
+
+ALTTAB_SCROLL_ENABLE
+- When ENCODER_DEFAULTACTIONS_ENABLE = yes,
+ Enables Alt-Tab scrolling functions in default encoder,
+ bind KS_TSTOG to a key to enable/disable Alt-Tab vs Volume control
+- When defining your own encoder functions use encoder_action_alttabscroll(bool clockwise) to assign the encodr action
+
+
+Layout
+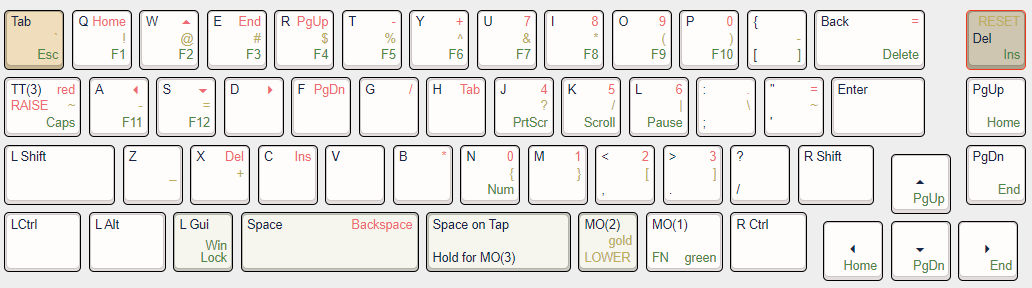
diff --git a/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/rules.mk b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/rules.mk
new file mode 100644
index 000000000..97ccf0c35
--- /dev/null
+++ b/keyboards/nopunin10did/kastenwagen48/keymaps/jonavin/rules.mk
@@ -0,0 +1,11 @@
+VIA_ENABLE = yes # VIA support
+LTO_ENABLE = yes #
+
+TAP_DANCE_ENABLE = yes
+
+TD_LSFT_CAPSLOCK_ENABLE = yes
+STARTUP_NUMLOCK_ON = yes
+
+ENCODER_DEFAULTACTIONS_ENABLE = yes
+INVERT_NUMLOCK_INDICATOR = yes
+ALTTAB_SCROLL_ENABLE = yes
diff --git a/users/jonavin/readme.md b/users/jonavin/readme.md
index 7712eda1e..204032ae1 100644
--- a/users/jonavin/readme.md
+++ b/users/jonavin/readme.md
@@ -120,6 +120,5 @@ LIST OF COMPATIBLE KEYMAPS
- mechwild/murphpad
- mechwild/OBE
- kbdfans/kdb67
-- nopunin10did/kastenwagen (*)
+- nopunin10did/kastenwagen48
- (*) coming soon